: Make a File Reader For this step, you will only need to modify, and thus focus on, the following class: • ReadShapeFile.java Figure 1: Empty Window with a Title: Shape Booooi- iinggg Frame You will also need to look at only the constructors of the following classes: • Circle.java • Oval.java Pretend the rest of the code does not exist. This is how we break large programs into smaller components that a human can understand and work with. It’s also how the rest of engineering works as well. Think about how the parts of a car engine are compartmentalised nicely into components. You don’t need to hold the entire functionality of the engine in your head at one time – just the component you are working on and how it interfaces with the rest of the system. It is very important that you only modify code in the files you are asked to modify. If your development environment suggests to change code outside these files don’t do it – even if it makes things magically compile and the red text disappears. Please assume the mistake is in the code you have just written and not in the code supplied. It is important you do not modify code supplied (except where indicated) because this could cause Autograder to fail to properly read your submission. In the first step of the assignment, you’ll create a file reader that reads the shape files supplied to you. Each line of the shape file specifies a shape. The format of this line differs depending on the shape to be created. Fortunately, the first entry of the line always indicates what shape is to be created from this input. For exam- ple, to create a circle: circle To create an Oval, the line in the file would be as fol- lows: oval All these entries are on a single line of the file. I have broken them into two lines for readability. Angular brackets are placeholders for numbers/values as fol- lows: • - time in milliseconds since the start of the program after which the shape is inserted • - The starting position of the shape in the plane
2.2 Step 1: Make a File Reader
For this step, you will only need to modify, and thus
focus on, the following class:
• ReadShapeFile.java
Figure 1: Empty Window with a Title: Shape Booooi-
iinggg Frame
You will also need to look at only the constructors of
the following classes:
• Circle.java
• Oval.java
Pretend the rest of the code does not exist. This is how
we break large programs into smaller components that
a human can understand and work with. It’s also how
the rest of engineering works as well. Think about how
the parts of a car engine are compartmentalised nicely
into components. You don’t need to hold the entire
functionality of the engine in your head at one time
– just the component you are working on and how it
interfaces with the rest of the system.
It is very important that you only modify code in the
files you are asked to modify. If your development
environment suggests to change code outside these
files don’t do it – even if it makes things magically
compile and the red text disappears. Please assume the
mistake is in the code you have just written and not in
the code supplied. It is important you do not modify
code supplied (except where indicated) because this
could cause Autograder to fail to properly read your
submission.
In the first step of the assignment, you’ll create a file reader that reads the shape files supplied to you. Each
line of the shape file specifies a shape. The format of
this line differs depending on the shape to be created.
Fortunately, the first entry of the line always indicates
what shape is to be created from this input. For exam-
ple, to create a circle:
circle <insertion time> <px> <py> <vx> <vy>
<filled?> <diameter> <r> <g> <b>
To create an Oval, the line in the file would be as fol-
lows:
oval <insertion time> <px> <py> <vx> <vy>
<filled?> <width> <height> <r> <g> <b>
All these entries are on a single line of the file. I have
broken them into two lines for readability. Angular
brackets are placeholders for numbers/values as fol-
lows:
• <insertion time> - time in milliseconds
since the start of the program after which the
shape is inserted
• <px> <py> - The starting position of the shape
in the plane
• <vx> <vy> - The velocity of the shape as a vec-
tor
• <filled?> - True if the shape is filled and false
otherwise
• <r> <g> <b> - The colour of the shape
For circle only, you have:
• <diameter> - the diameter of the circle
For oval only, you have:
• <width> <height> - the width and height
(major and minor axis) of the oval.
You cannot modify the file format in this assignment.
These files will be automatically on autograder and if
you modify file format your program marking will fail.
In ReadShapeFile.java, write methods to read
files consisting of circles and ovals only. Also, you
can assume that the file is sorted by insertion time.
This means that the lines of the file must be in increas-
ing <insertion time> order. I would start by trying to read a single line of the file
followed by multiple lines, printing them to the screen
to ensure you are reading the data correctly. Then, cre-
ate instances of the shape objects and print them out.
You can do this by calling the toString() method
that has already been given to you.
If the file does not exist, quit the program grace-
fully and output Could not find <filename>
to the screen (standard out) with <filename> re-
placed by the file that will not open. However, you
can assume that each line of the file has the cor-
rect syntax. To test your code, please try to run the
TwoRedCircles.txt file. Start by printing out
each line your read to the terminal to make sure you
are reading the data correctly. Then, create instances
of the objects and use the toString() method to
print out the object and make sure that it is being cre-
ated correctly.


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

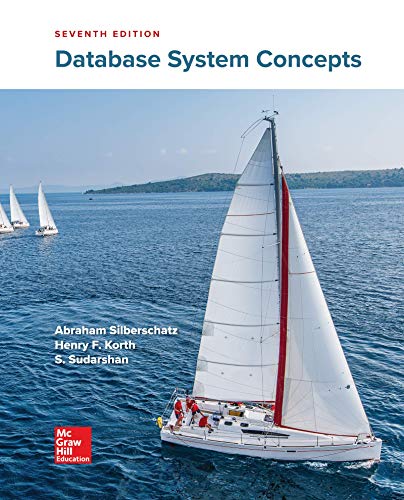
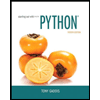
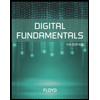
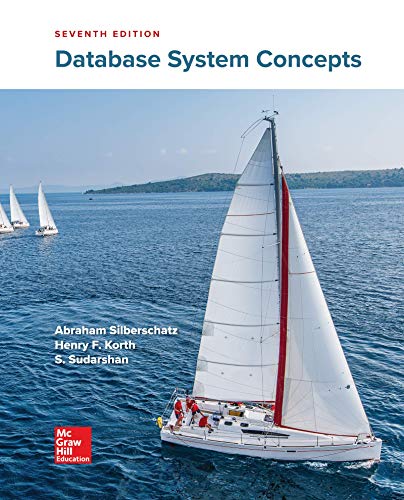
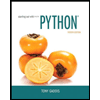
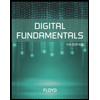
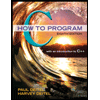
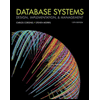
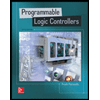