Make a C-Language code of the problem: Modify the code such that the program will be able to generate a list of the first N perfect square numbers and give out its sum. Perfect squares are the squares of whole numbers: 1, 4, 9, 16, 25, 36, ... etc. See the sample output in the picture #include bool isPerfectSquare(int x){ //insert code for isPerfectSquare() function here } int getSum(int *arr, int size){ //insert code for getSum() function here } void printArray(int *arr, int size){ //insert code for printArray() function here } int main(){ //insert code for main() function here return 0; } Note: Do not change the initial function definitions. You are free to use more functions and variables as you see fit.
Make a C-Language code of the problem:
Modify the code such that the
Perfect squares are the squares of whole numbers: 1, 4, 9, 16, 25, 36, ... etc.
See the sample output in the picture
#include <stdio.h>
bool isPerfectSquare(int x){
//insert code for isPerfectSquare() function here
}
int getSum(int *arr, int size){
//insert code for getSum() function here
}
void printArray(int *arr, int size){
//insert code for printArray() function here
}
int main(){
//insert code for main() function here
return 0;
}
Note: Do not change the initial function definitions. You are free to use more functions and variables as you see fit.


Step by step
Solved in 3 steps with 1 images

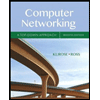
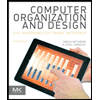
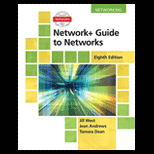
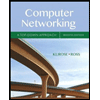
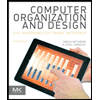
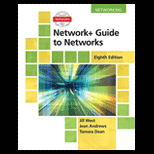
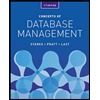
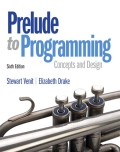
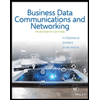