main question: in python Implement the simplest client-server gRPC application "HelloWorld", which consists in sending a message to a remote server and receiving a response from it. *I will try to explain much I can because each time run it it doesn't work. please I do my best to explain the task and provide the code please help me do it for me cause I stuck in, I separate each code to be clear: Let's consider the simplest example of realization of the application using remote method invocation on GRPC technology. In the PyCharm environment, create a new project named GRPC with program packages written in Python. Open a terminal window and type the following commands python -m pip install --upgrade pip python -m pip install grpcio python -m pip install grpcio-tools after: The gRPC protocol, like many other RPCs, is based on the idea of describing services and methods that can be invoked remotely. By default, gRPC uses Protocol Buffer to describe services and message structure. For .proto files we will create a separate directory in our project structure with the name "protos": In this directory, create a file called "helloworld.proto" in the file helloword.proto inside the directory protos we will run this: -------------------------------------------------------------------------------------------------------------------------------- syntax = "proto3"; package helloworld; service Greeter { rpc SayHello (HelloRequest) returns (HelloReply) {} } message HelloRequest { string name = 1; string surname = 2; } message HelloReply { string message = 1; } --------------------------------------------------------------------------------------------------------------- After that, we need to compile this file by typing the command in the terminal: python -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. protos/helloworld.proto Create 2 new python files named "Server.py" and "Client.py" At this point, your project should contain the following files: Files с names "helloworld_pb2" & "helloworld_pb2_grpc" are generated automatically when "helloworld.proto" is compiled Realize the source code of the server.py: -------------------------------------------------------------------------------------------------------------------------------------- from concurrent import futures import time import grpc import helloworld_pb2 import helloworld_pb2_grpc _ONE_DAY_IN_SECONDS = 60 * 60 * 24 class Greeter(helloworld_pb2_grpc.GreeterServicer): def SayHello(self, request, context): return helloworld_pb2.HelloReply(message='Hello, %s %s!' % (request.name, request.surname)) def serve(): server = grpc.server(futures.ThreadPoolExecutor(max_workers=10)) helloworld_pb2_grpc.add_GreeterServicer_to_server(Greeter(), server) server.add_insecure_port('[::]:50051') server.start() try: while True: time.sleep(_ONE_DAY_IN_SECONDS) except KeyboardInterrupt: server.stop(0) if __name__ == '__main__': greeter_instance = Greeter() greeter_instance.serve() --------------------------------------------------------------------------------------------------------------------------- Implement the client.py source code: ------------------------------------------------------------------------------------------------------------------------ import grpc import helloworld_pb2 import helloworld_pb2_grpc def run(): channel = grpc.insecure_channel('localhost:50051') stub = helloworld_pb2_grpc.GreeterStub(channel) response = stub.SayHello(helloworld_pb2.HelloRequest(name='dear', surname='friend')) print("Greeter client received: \n" + response.message + '\n') if __name__ == '__main__': run() ---------------------------------------------------------------------------------------------------------------- After that, we can call it in: response = stub.SayHello(helloworld_pb2.HelloRequest(name='dear', surname='friend'))
|
main question: in python Implement the simplest client-server gRPC application "HelloWorld", which consists in sending a message to a remote server and receiving a response from it. *I will try to explain much I can because each time run it it doesn't work. please I do my best to explain the task and provide the code please help me do it for me cause I stuck in, I separate each code to be clear: Let's consider the simplest example of realization of the application using remote method invocation with program packages written in Python. Open a terminal window and type the following commands python -m pip install --upgrade pip after: The gRPC protocol, like many other RPCs, is based on the idea of describing services and In this directory, create a file called "helloworld.proto" in the file helloword.proto inside the directory protos we will run this: -------------------------------------------------------------------------------------------------------------------------------- syntax = "proto3"; service Greeter { message HelloRequest { message HelloReply { --------------------------------------------------------------------------------------------------------------- After that, we need to compile this file by typing the command in the terminal: python -m grpc_tools.protoc -I. --python_out=. --grpc_python_out=. protos/helloworld.proto Create 2 new python files named "Server.py" and "Client.py" At this Realize the source code of the server.py: -------------------------------------------------------------------------------------------------------------------------------------- from concurrent import futures _ONE_DAY_IN_SECONDS = 60 * 60 * 24 class Greeter(helloworld_pb2_grpc.GreeterServicer): def SayHello(self, request, context): def serve(): if __name__ == '__main__': --------------------------------------------------------------------------------------------------------------------------- Implement the client.py source code: ------------------------------------------------------------------------------------------------------------------------ import grpc def run(): if __name__ == '__main__': ---------------------------------------------------------------------------------------------------------------- After that, we can call it in: response = stub.SayHello(helloworld_pb2.HelloRequest(name='dear', |



Answer:
The given python files and the steps you followed are seems to be correct, but there was a small problem regarding to the server.py program. Let us resolve that.
The serve() function should be called at the end, not the greeter_instance.serve().
server.py:
from concurrent import futures
import time
import grpc
import helloworld_pb2
import helloworld_pb2_grpc
_ONE_DAY_IN_SECONDS = 60 * 60 * 24
class Greeter(helloworld_pb2_grpc.GreeterServicer):
def SayHello(self, request, context):
return helloworld_pb2.HelloReply(message='Hello, %s %s!' % (request.name, request.surname))
def serve():
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
helloworld_pb2_grpc.add_GreeterServicer_to_server(Greeter(), server)
server.add_insecure_port('[::]:50051')
server.start()
try:
while True:
time.sleep(_ONE_DAY_IN_SECONDS)
except KeyboardInterrupt:
server.stop(0)
if __name__ == '__main__':
serve() #modification
Step by step
Solved in 3 steps

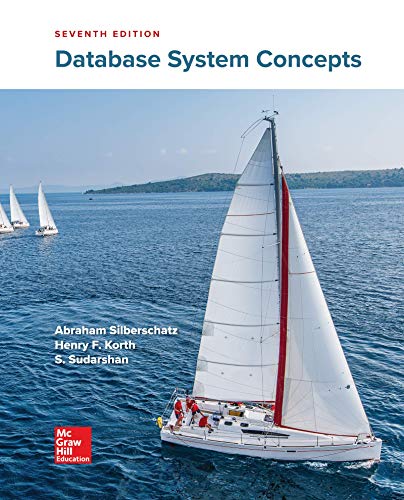
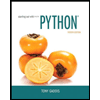
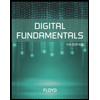
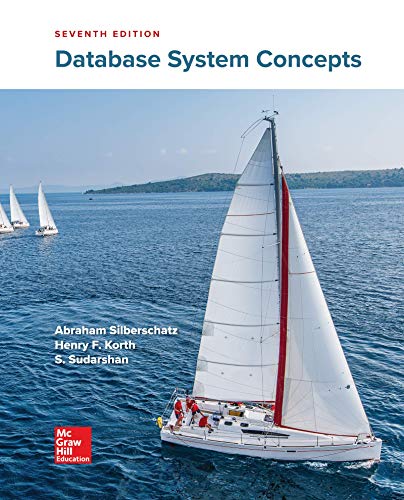
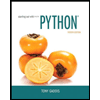
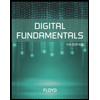
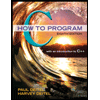
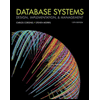
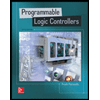