LLNode.java by Dale/Joyce/Weems Chapter 3 // // Implements nodes for a Linked List. //---------------------------------------------------------------------------- package Linkedqueue; public class LLNode { private LLNode link; private T info; public LLNode(T info) { this.info = info; link = null; } public void setInfo(T info) // Sets info of this LLNode. { this.info = info; } public T getInfo() // Returns info of this LLONode. { return info; } public void setLink(LLNode link) // Sets link of this LLNode. { this.link = link; } public LLNode getLink() // Returns link of this LLNode. { return link; } } //---------------------------------------------------------------------------- // LLNode.java by Dale/Joyce/Weems Chapter 3 // // Implements nodes for a Linked List. //---------------------------------------------------------------------------- package Linkededstack; public class LLNode { private LLNode link; private T info; public LLNode(T info) { this.info = info; link = null; } public void setInfo(T info) // Sets info of this LLNode. { this.info = info; } public T getInfo() // Returns info of this LLONode. { return info; } public void setLink(LLNode link) // Sets link of this LLNode. { this.link = link; } public LLNode getLink() // Returns link of this LLNode. { return link; } } /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package Linked_List; /** * * @author lab1257-15 */ public class LLNode { private LLNode link; private T info;
//----------------------------------------------------------------------------
// LLNode.java by Dale/Joyce/Weems Chapter 3
//
// Implements <T> nodes for a Linked List.
//----------------------------------------------------------------------------
package Linkedqueue;
public class LLNode<T>
{
private LLNode<T> link;
private T info;
public LLNode(T info)
{
this.info = info;
link = null;
}
public void setInfo(T info)
// Sets info of this LLNode.
{
this.info = info;
}
public T getInfo()
// Returns info of this LLONode.
{
return info;
}
public void setLink(LLNode<T> link)
// Sets link of this LLNode.
{
this.link = link;
}
public LLNode<T> getLink()
// Returns link of this LLNode.
{
return link;
}
}
//----------------------------------------------------------------------------
// LLNode.java by Dale/Joyce/Weems Chapter 3
//
// Implements <T> nodes for a Linked List.
//----------------------------------------------------------------------------
package Linkededstack;
public class LLNode<T>
{
private LLNode<T> link;
private T info;
public LLNode(T info)
{
this.info = info;
link = null;
}
public void setInfo(T info)
// Sets info of this LLNode.
{
this.info = info;
}
public T getInfo()
// Returns info of this LLONode.
{
return info;
}
public void setLink(LLNode<T> link)
// Sets link of this LLNode.
{
this.link = link;
}
public LLNode<T> getLink()
// Returns link of this LLNode.
{
return link;
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Linked_List;
/**
*
* @author lab1257-15
*/
public class LLNode<T>
{
private LLNode<T> link;
private T info;
public LLNode(T info)
{
this.info = info;
link = null;
}
public void setInfo(T info)
// Sets info of this LLNode.
{
this.info = info;
}
public T getInfo()
// Returns info of this LLONode.
{
return info;
}
public void setLink(LLNode<T> link)
// Sets link of this LLNode.
{
this.link = link;
}
public LLNode<T> getLink()
// Returns link of this LLNode.
{
return link;
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Linked_List;
import java.util.Scanner;
/**
*
* @author lab1257-15
*/
public class Demo {
public static boolean has42 (RefUnsortedList<Integer> t)
{
t.reset();
for (int i=1; i<=t.size();i++)
if (t.getNext()==42)
return true;
return false;
}
public static void RemoveAll(RefUnsortedList<Integer> t, int v)
{
while(t.contains(v))
{
t.remove(v);
}
}
public static void main(String[] args) {
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

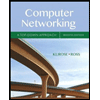
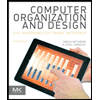
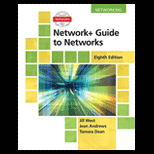
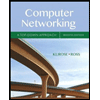
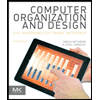
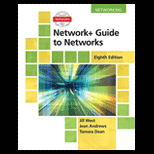
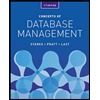
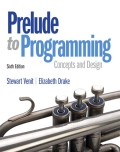
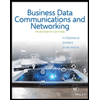