/llcpInt.cpp:28:14: error: no viable overloaded '=' temp=headPtr; ~~~~^~~~~~~~ ./llcpInt.h:6:8: note: candidate function (the implicit copy assignment operator) not viable: no known conversion from 'Node *' to 'const Node' for 1st argument; dereference the argument with * struct Node ^ ./llcpInt.h:6:8: note: candidate function (the implicit move assignment operator) not viable: no known conversion from 'Node *' to 'Node' for 1st argument; dereference the argument with * struct Node ^ ./llcpInt.cpp:30:10: error: cannot delete expression of type 'Node' delete temp; ^ ~~~~ ./llcpInt.cpp:38:15: error: no viable overloaded '=' temp=currentNode->link; ~~~~^~~~~~~~~~~~~~~~~~ ./llcpInt.h:6:8: note: candidate function (the implicit copy assignment operator) not viable: no known conversion from 'Node *' to 'const Node' for 1st argument; dereference the argument with * struct Node ^ ./llcpInt.h:6:8: note: candidate function (the implicit move assignment operator) not viable: no known conversion from 'Node *' to 'Node' for 1st argument; dereference the argument with * struct Node ^ ./llcpInt.cpp:39:33: error: member reference type 'Node' is not a pointer; did you mean to use '.'? currentNode->link=temp->link; ~~~~^~ . ./llcpInt.cpp:40:11: error: cannot delete expression of type 'Node' delete temp; ^ ~~~~ 5 errors generated. make: *** [Makefile:10: main] Error 1 */ bool DelOddCopEven(Node* headPtr) { Node* currentNode, temp; if (headPtr == 0) // empty or 1-node return true; else if ( headPtr->data %2!= 0){ currentNode=headPtr->link; temp=headPtr; headPtr=currentNode; delete temp; } else{ currentNode=headPtr; } while (currentNode->link != 0) // not at last node { if (currentNode->link->data %2!=0){ temp=currentNode->link; currentNode->link=temp->link; delete temp; currentNode=currentNode->link; } else{ struct Node* dup; dup->data=currentNode->link->data; dup->link=currentNode->link->link; currentNode->link->link=dup; } } return true; }
./llcpInt.cpp:28:14: error: no viable overloaded '='
temp=headPtr;
~~~~^~~~~~~~
./llcpInt.h:6:8: note: candidate function (the implicit copy assignment operator) not viable: no known conversion from 'Node *' to 'const Node' for 1st argument; dereference the argument with *
struct Node
^
./llcpInt.h:6:8: note: candidate function (the implicit move assignment operator) not viable: no known conversion from 'Node *' to 'Node' for 1st argument; dereference the argument with *
struct Node
^
./llcpInt.cpp:30:10: error: cannot delete expression of type 'Node'
delete temp;
^ ~~~~
./llcpInt.cpp:38:15: error: no viable overloaded '='
temp=currentNode->link;
~~~~^~~~~~~~~~~~~~~~~~
./llcpInt.h:6:8: note: candidate function (the implicit copy assignment operator) not viable: no known conversion from 'Node *' to 'const Node' for 1st argument; dereference the argument with *
struct Node
^
./llcpInt.h:6:8: note: candidate function (the implicit move assignment operator) not viable: no known conversion from 'Node *' to 'Node' for 1st argument; dereference the argument with *
struct Node
^
./llcpInt.cpp:39:33: error: member reference type 'Node' is not a pointer; did you mean to use '.'?
currentNode->link=temp->link;
~~~~^~
.
./llcpInt.cpp:40:11: error: cannot delete expression of type 'Node'
delete temp;
^ ~~~~
5 errors generated.
make: *** [Makefile:10: main] Error 1
*/
bool DelOddCopEven(Node* headPtr)
{
Node* currentNode, temp;
if (headPtr == 0) // empty or 1-node
return true;
else if ( headPtr->data %2!= 0){
currentNode=headPtr->link;
temp=headPtr;
headPtr=currentNode;
delete temp;
}
else{
currentNode=headPtr;
}
while (currentNode->link != 0) // not at last node
{
if (currentNode->link->data %2!=0){
temp=currentNode->link;
currentNode->link=temp->link;
delete temp;
currentNode=currentNode->link;
}
else{
struct Node* dup;
dup->data=currentNode->link->data;
dup->link=currentNode->link->link;
currentNode->link->link=dup;
}
}
return true;
}

Step by step
Solved in 3 steps

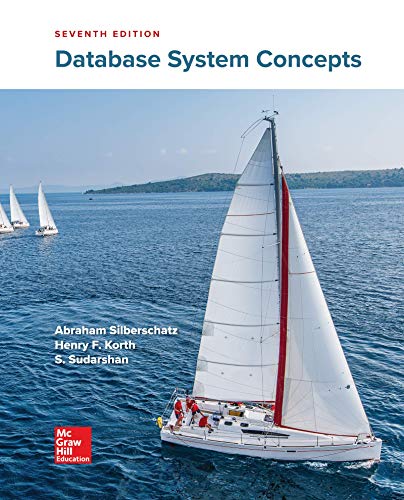
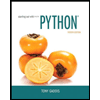
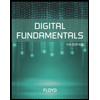
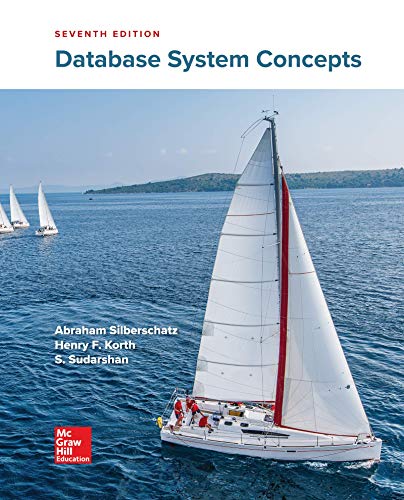
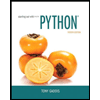
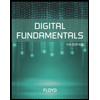
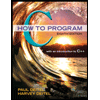
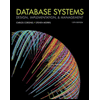
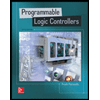