OpenGL programming (c++) The program should generate a triangle that should move in response to the left mouse button being held down and the mouse moved. The figure should stop at the defined window boundaries (N,S,E,W) making sure that the entire figure is always present in your window.
OpenGL
The program should generate a triangle that should move in response to the left mouse button being held down and the mouse moved. The figure should stop at the defined window boundaries (N,S,E,W) making sure that the entire figure is always present in your window.

To create an OpenGL program in C++ that generates a moving triangle in response to mouse events, you can follow these steps:
1)Include the necessary header files for OpenGL and GLUT:
#include <GL/glut.h>
#include <GL/gl.h>
2)Define the initial positions of the triangle.
GLfloat triangleX = 0.0;
GLfloat triangleY = 0.0;
3)Create a function that draws the triangle:
void drawTriangle()
{
glPushMatrix();
glTranslatef(triangleX, triangleY, 0.0);
glBegin(GL_TRIANGLES);
glVertex3f(-0.5, -0.5, 0.0);
glVertex3f(0.5, -0.5, 0.0);
glVertex3f(0.0, 0.5, 0.0);
glEnd();
glPopMatrix();
}
4)Create a function that responds to mouse events
void mouse(int button, int state, int x, int y)
{
if (button == GLUT_LEFT_BUTTON && state == GLUT_DOWN)
{
// Save the initial position of the mouse
lastX = x;
lastY = y;
}
}
5)Create a function that moves the triangle when the mouse is movedCreate a function that moves the triangle when the mouse is moved
void motion(int x, int y)
{
// Calculate the distance the mouse moved
GLfloat deltaX = x - lastX;
GLfloat deltaY = y - lastY;
// Update the position of the triangle
triangleX += deltaX / windowWidth;
triangleY -= deltaY / windowHeight;
// Make sure the triangle stays within the window boundaries
if (triangleX > 0.5)
{
triangleX = 0.5;
}
else if (triangleX < -0.5)
{
triangleX = -0.5;
}
if (triangleY > 0.5)
{
triangleY = 0.5;
}
else if (triangleY < -0.5)
{
triangleY = -0.5;
}
// Save the current position of the mouse
lastX = x;
lastY = y;
// Redraw the scene
glutPostRedisplay();
}
Step by step
Solved in 2 steps

lastX = x; and lastY = y;
they are giving me an error "undefined" how do I fix it?
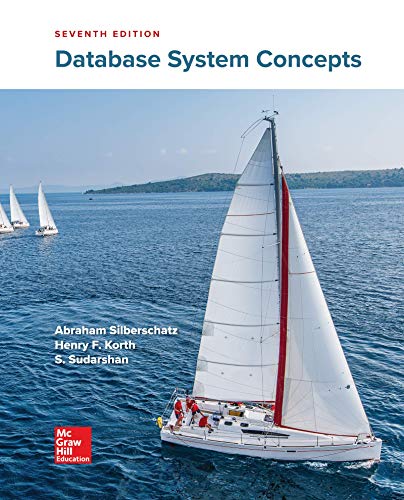
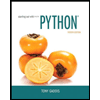
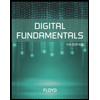
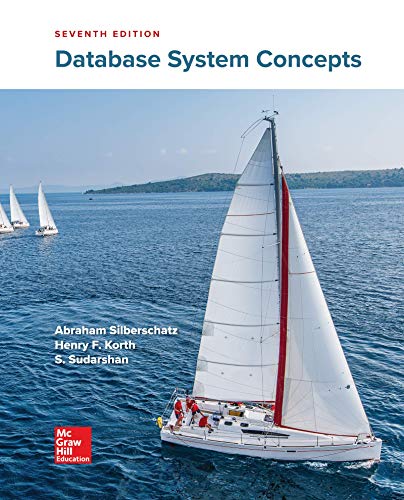
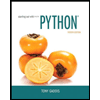
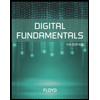
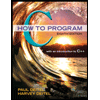
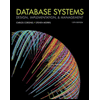
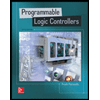