Jump to level String figDataName is read from input. The try block opens a file named figDataName using a try-with-resources statement, and reads an integer from the file into figData. Write an exception handler to catch a FileNotFoundException and output figDataName, followed by ": File not available". End with a newline. Ex: If the input is fig1.txt, then the output is: Value read from figl.txt: 36 Ex: If the input is nosuchfile.txt, then the output is: nosuchfile.txt: File not available 1 import java.util.Scanner; 2 import java.io.FileInputStream; 3 import java.io.FileNotFoundException; 4 5 public class ReadDataFile { 6 7 8 9 10 11 12 13 14 15 16 17 18 ReadDataFile.java fig1.txt fig2.txt fig3.txt 2015 public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String figDataName; int figData; figDataName = scnr.next(); try (Scanner fInScanner = new Scanner(new FileInputStream(figDataName))) { figData = fInScanner.nextInt (); System.out.println("Value read from " + figDataName + ": + figData); } /* fInScanner is automatically closed after the try block terminates.
Jump to level String figDataName is read from input. The try block opens a file named figDataName using a try-with-resources statement, and reads an integer from the file into figData. Write an exception handler to catch a FileNotFoundException and output figDataName, followed by ": File not available". End with a newline. Ex: If the input is fig1.txt, then the output is: Value read from figl.txt: 36 Ex: If the input is nosuchfile.txt, then the output is: nosuchfile.txt: File not available 1 import java.util.Scanner; 2 import java.io.FileInputStream; 3 import java.io.FileNotFoundException; 4 5 public class ReadDataFile { 6 7 8 9 10 11 12 13 14 15 16 17 18 ReadDataFile.java fig1.txt fig2.txt fig3.txt 2015 public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String figDataName; int figData; figDataName = scnr.next(); try (Scanner fInScanner = new Scanner(new FileInputStream(figDataName))) { figData = fInScanner.nextInt (); System.out.println("Value read from " + figDataName + ": + figData); } /* fInScanner is automatically closed after the try block terminates.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
JAVA code can ony be added after line 16 of code
fig1.txt
36
fig2.txt
29
fig3.txt
25
![## Handling File Input with Exception Handling in Java
### Overview
In this example, we are learning how to use Java to read an integer from a file and handle exceptions using a try-with-resources statement. The program reads the filename from input and attempts to open and read from it. If the file does not exist, an exception is caught, and an error message is displayed.
### Instructions
1. **Read input for filename:**
A string `figDataName` is read from input, which is the name of the file you wish to read.
2. **Try-with-resources statement:**
This block of code opens a file using the provided filename and attempts to read an integer from it into the variable `figData`.
3. **Exception Handling:**
If the file is not found, a `FileNotFoundException` is caught. The program then outputs the filename followed by `": File not available"`.
### Example Outputs
- If the input filename is `fig1.txt`, and the file exists with the content `36`, the output will be:
```
Value read from fig1.txt: 36
```
- If the input filename is `nosuchfile.txt`, and the file does not exist, the output will be:
```
nosuchfile.txt: File not available
```
### Java Code
Here's a breakdown of the Java code used for this task:
```java
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class ReadDataFile {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String figDataName;
int figData;
figDataName = scnr.next();
try (Scanner finScanner = new Scanner(new FileInputStream(figDataName))) {
figData = finScanner.nextInt();
System.out.println("Value read from " + figDataName + ": " + figData);
} catch (FileNotFoundException e) {
System.out.println(figDataName + ": File not available");
}
}
}
```
### Key Concepts
- **Try-with-resources:** This ensures that the `Scanner` is closed automatically after the try block is terminated.
- **FileNotFoundException:** This exception is specific to the scenario where the specified file is not present.
### Additional Resources
- [Java Documentation on Try-with](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2F77e8ea36-0f2e-4ce2-8628-db396c755cae%2Fwzawg3_processed.png&w=3840&q=75)
Transcribed Image Text:## Handling File Input with Exception Handling in Java
### Overview
In this example, we are learning how to use Java to read an integer from a file and handle exceptions using a try-with-resources statement. The program reads the filename from input and attempts to open and read from it. If the file does not exist, an exception is caught, and an error message is displayed.
### Instructions
1. **Read input for filename:**
A string `figDataName` is read from input, which is the name of the file you wish to read.
2. **Try-with-resources statement:**
This block of code opens a file using the provided filename and attempts to read an integer from it into the variable `figData`.
3. **Exception Handling:**
If the file is not found, a `FileNotFoundException` is caught. The program then outputs the filename followed by `": File not available"`.
### Example Outputs
- If the input filename is `fig1.txt`, and the file exists with the content `36`, the output will be:
```
Value read from fig1.txt: 36
```
- If the input filename is `nosuchfile.txt`, and the file does not exist, the output will be:
```
nosuchfile.txt: File not available
```
### Java Code
Here's a breakdown of the Java code used for this task:
```java
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
public class ReadDataFile {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String figDataName;
int figData;
figDataName = scnr.next();
try (Scanner finScanner = new Scanner(new FileInputStream(figDataName))) {
figData = finScanner.nextInt();
System.out.println("Value read from " + figDataName + ": " + figData);
} catch (FileNotFoundException e) {
System.out.println(figDataName + ": File not available");
}
}
}
```
### Key Concepts
- **Try-with-resources:** This ensures that the `Scanner` is closed automatically after the try block is terminated.
- **FileNotFoundException:** This exception is specific to the scenario where the specified file is not present.
### Additional Resources
- [Java Documentation on Try-with
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
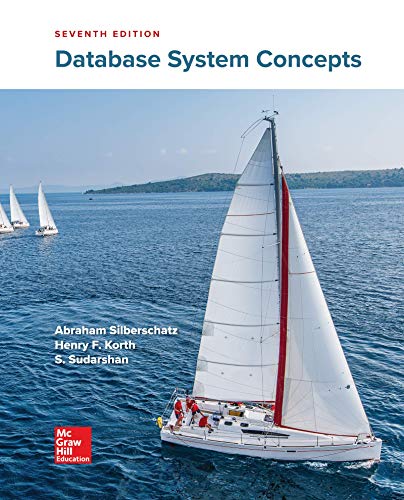
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
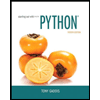
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
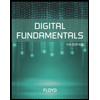
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
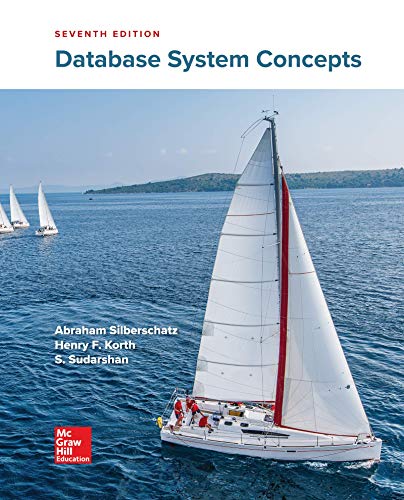
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
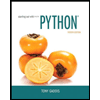
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
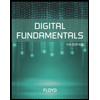
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
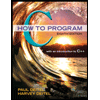
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
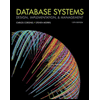
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
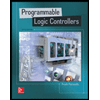
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education