Javascript toggleCommentSection function The function toggleCommentSection should exist. The function toggleCommentSection should return undefined if not passed a parameter. The function toggleCommentSection should return null if passed a parameter that does not match a post ID. The function toggleCommentSection should select and return the correct section element. The function toggleCommentSection should toggle the 'hide' class on the section The following are requirements of the function toggleCommentSection: element.toggleCommentSection a. Receives a postId as the parameter b. Selects the section element with the data-post-id attribute equal to the postId received as a parameter c. Use code to verify the section exists before attempting to access the classList property d. At this point in your code, the section will not exist. You can create one to test if desired. e. Toggles the class 'hide' on the section element f. Return the section element Here is what I have so far: function toggleCommentSection(postId) { // If Post Id Is Passed, Return Undefined if (!postId) { return undefined; } else { // Else, Get All Comment Sections const commentSections = document.querySelectorAll('[data-post-id]'); // Loop Through Each Comment Section for (let i = 0; i < commentSections.length; i++) { const commentSection = commentSections[i]; // If Post Id Attribut Of Comment Section Is Equal To Post Id Passed Arg if (commentSection.getAttribute('data-post-id') === postId) { // Toggle Hide Class commentSection.classList.toggle('hide'); // Return Comment Section Element return commentSection; } } // If We Are Here, No Matching Post Id Is Found // Return NULL return null; } } The 1st 3 requirements pass but the last 2 failed: The function toggleCommentSection should exist. - passed The function toggleCommentSection should return undefined if not passed a parameter. -passed The function toggleCommentSection should return null if passed a parameter that does not match a post ID. - passed The function toggleCommentSection should select and return the correct section element - failed TypeError: Cannot read properties of null (reading 'dataset') The function toggleCommentSection should toggle the 'hide' class on the section element. - failed TypeError: Cannot read properties of null (reading 'classList') Do you need more information?
Javascript
toggleCommentSection function
The function toggleCommentSection should exist.
The function toggleCommentSection should return undefined if not passed a parameter.
The function toggleCommentSection should return null if passed a parameter that does not match a post ID.
The function toggleCommentSection should select and return the correct section element.
The function toggleCommentSection should toggle the 'hide' class on the section
The following are requirements of the function toggleCommentSection: element.toggleCommentSection a. Receives a postId as the parameter b. Selects the section element with the data-post-id attribute equal to the postId received as a parameter c. Use code to verify the section exists before attempting to access the classList property d. At this point in your code, the section will not exist. You can create one to test if desired. e. Toggles the class 'hide' on the section element f. Return the section element
Here is what I have so far:
function toggleCommentSection(postId) {
// If Post Id Is Passed, Return Undefined
if (!postId) {
return undefined;
} else {
// Else, Get All Comment Sections
const commentSections = document.querySelectorAll('[data-post-id]');
// Loop Through Each Comment Section
for (let i = 0; i < commentSections.length; i++) {
const commentSection = commentSections[i];
// If Post Id Attribut Of Comment Section Is Equal To Post Id Passed Arg
if (commentSection.getAttribute('data-post-id') === postId) {
// Toggle Hide Class
commentSection.classList.toggle('hide');
// Return Comment Section Element
return commentSection;
}
}
// If We Are Here, No Matching Post Id Is Found
// Return NULL
return null;
}
}
The 1st 3 requirements pass but the last 2 failed:
- The function toggleCommentSection should exist. - passed
- The function toggleCommentSection should return undefined if not passed a parameter. -passed
- The function toggleCommentSection should return null if passed a parameter that does not match a post ID. - passed
- The function toggleCommentSection should select and return the correct section element - failed TypeError: Cannot read properties of null (reading 'dataset')
- The function toggleCommentSection should toggle the 'hide' class on the section element. - failed TypeError: Cannot read properties of null (reading 'classList')
Do you need more information?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

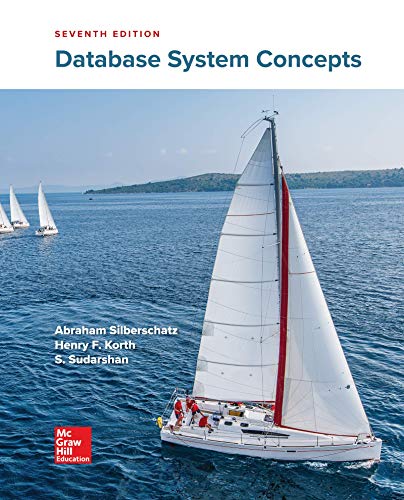
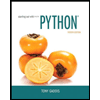
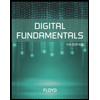
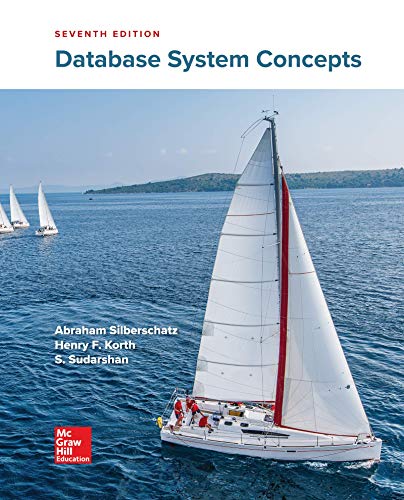
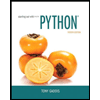
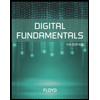
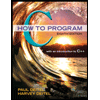
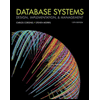
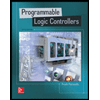