JavaScript imageMosaic: compute image mosaic. You must implement the final version of the image mosaic algorithm we discussed in class (which includes the scaling of mosaic images and also randomization). Since the algorithm involves several nested loops and can take a while to run, this function will be implemented using a non-blocking loop so that the browser will not complain about its running speed. Details are in the comments embedded in the code. function imageMosaic(input, output, mosaic_name) { let width = input.width; let height = input.height; let mimages = mosaics[mosaic_name]; // mimages is an array of mosaic images let w = mimages[0].width; // all mosaic images have the same size wxh let h = mimages[0].height; let num = mimages.length; // number of mosaic images console.log('Computing Image Mosaic...'); let y = 0; (function chunk() { for(x = 0; x <= width-w; x += w) { // x loop // ===YOUR CODE STARTS HERE=== /* for(y=0; y<=input.height-h; y+=h) { for (x=0; x<=input.width-w; x+=w) { // loop over blocks for(k=0; k<#candidates; k++) { // loop over candidates d_r = d_g = d_b = 0; for(j=0; j
JavaScript
-
imageMosaic: compute image mosaic. You must implement the final version of the image mosaic
algorithm we discussed in class (which includes the scaling of mosaic images and also randomization). Since the algorithm involves several nested loops and can take a while to run, this function will be implemented using a non-blocking loop so that the browser will not complain about its running speed. Details are in the comments embedded in the code.
function imageMosaic(input, output, mosaic_name) {
let width = input.width;
let height = input.height;
let mimages = mosaics[mosaic_name]; // mimages is an array of mosaic images
let w = mimages[0].width; // all mosaic images have the same size wxh
let h = mimages[0].height;
let num = mimages.length; // number of mosaic images
console.log('Computing Image Mosaic...');
let y = 0;
(function chunk() {
for(x = 0; x <= width-w; x += w) { // x loop
// ===YOUR CODE STARTS HERE===
/*
for(y=0; y<=input.height-h; y+=h) {
for (x=0; x<=input.width-w; x+=w) { // loop over blocks
for(k=0; k<#candidates; k++) { // loop over candidates
d_r = d_g = d_b = 0;
for(j=0; j<h; j++) {
for(i=0; i<w; i++) { // loop over pixels in block
update d_r, d_g, d_b;
}
}
calculate d = d_r + d_g + d_b;
multiply d with a random noise between 1 and 2
keep track of best matches (i.e. smallest d)
keep track of scales a_r, a_g, a_b of best match
}
copy the best match, multiplied by the scales, to output image block.
}
}
*/
}
}
}
// ---YOUR CODE ENDS HERE---
}
output.updatePixels();
y+=h;
if (y <= height-h) { // y loop in non-blocking version
setTimeout(chunk, 0);
} else {
console.log('Done.');
}
})();
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

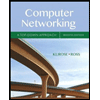
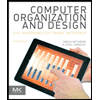
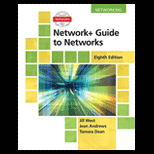
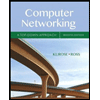
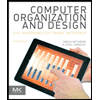
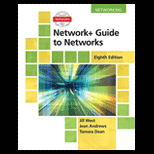
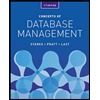
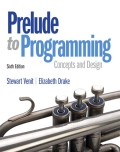
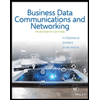