java.lang.StringBuilder uses a resizing array to represent a string of length n, with the first character in the string at array index 0 and the last character in the string at array index n - 1. Assume the array length is doubled when full and halved when one-quarter full. For reference, here is a partial API public class StringBuilder StringBuilder() create an empty string void append(char c) add character c to the end of the string void prepend(char c) add character c to the beginning of the string char charAt(int i) return the character at index i and a diagram of the underlying representation for reference. first character last character a[] M. D R M. 1. 2. 3 7. Mark all properties that apply. O e(1) time in the worst case for charAt(). O e(1) time in the worst case for append. Starting from an empty StringBuilder, any sequence of n append operations takes O(n) time in the worst case. Starting from an empty stringBuilder, any sequence of n append and prepend operations takes (n) time in the worst case. OUses (n) memory in the worst case.
java.lang.StringBuilder uses a resizing array to represent a string of length n, with the first character in the string at array index 0 and the last character in the string at array index n - 1. Assume the array length is doubled when full and halved when one-quarter full. For reference, here is a partial API public class StringBuilder StringBuilder() create an empty string void append(char c) add character c to the end of the string void prepend(char c) add character c to the beginning of the string char charAt(int i) return the character at index i and a diagram of the underlying representation for reference. first character last character a[] M. D R M. 1. 2. 3 7. Mark all properties that apply. O e(1) time in the worst case for charAt(). O e(1) time in the worst case for append. Starting from an empty StringBuilder, any sequence of n append operations takes O(n) time in the worst case. Starting from an empty stringBuilder, any sequence of n append and prepend operations takes (n) time in the worst case. OUses (n) memory in the worst case.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please answer ASAP
![### Java StringBuilder Overview
The `java.lang.StringBuilder` class uses a resizing array to represent a string of length \( n \). The first character in the string is placed at array index 0, and the last character is at array index \( n - 1 \). The array length is doubled when full and halved when one-quarter full to efficiently manage memory.
#### Partial API Reference
```java
public class StringBuilder {
StringBuilder() // create an empty string
void append(char c) // add character c to the end of the string
void prepend(char c) // add character c to the beginning of the string
char charAt(int i) // return the character at index i
}
```
#### Diagram of Underlying Representation
A diagram is provided to illustrate how the `StringBuilder` manages its character array:
- **Array Indexing:**
- The array is indexed from 0 to \( n \).
- Characters are stored sequentially in the array with unused space illustrated after the last character.
- **Example:**
- First character: Index 0 ('M')
- Last character: Index 6 ('M')
- Array contents: ['M', 'I', 'D', 'T', 'E', 'R', 'M', '-']
- Unused space (indicated by '-') follows the last character.
```plaintext
first character last character
a[] -> M I D T E R M -
0 1 2 3 4 5 6 7
```
#### Performance Properties
Please mark any properties that apply:
- [ ] \( \Theta(1) \) time in the worst case for `charAt()`.
- [ ] \( \Theta(1) \) time in the worst case for `append`.
- [ ] Starting from an empty `StringBuilder`, any sequence of \( n \) `append` operations takes \( \Theta(n) \) time in the worst case.
- [ ] Starting from an empty `StringBuilder`, any sequence of \( n \) `append` and `prepend` operations takes \( \Theta(n) \) time in the worst case.
- [ ] Uses \( \Theta(n) \) memory in the worst case.
This overview is designed to assist students in](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F88167701-6d3f-46b8-9f75-5ab21a2347bf%2F0feb2e81-a030-42cf-bec2-dfac42b9c8bf%2Ftarpfeg_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Java StringBuilder Overview
The `java.lang.StringBuilder` class uses a resizing array to represent a string of length \( n \). The first character in the string is placed at array index 0, and the last character is at array index \( n - 1 \). The array length is doubled when full and halved when one-quarter full to efficiently manage memory.
#### Partial API Reference
```java
public class StringBuilder {
StringBuilder() // create an empty string
void append(char c) // add character c to the end of the string
void prepend(char c) // add character c to the beginning of the string
char charAt(int i) // return the character at index i
}
```
#### Diagram of Underlying Representation
A diagram is provided to illustrate how the `StringBuilder` manages its character array:
- **Array Indexing:**
- The array is indexed from 0 to \( n \).
- Characters are stored sequentially in the array with unused space illustrated after the last character.
- **Example:**
- First character: Index 0 ('M')
- Last character: Index 6 ('M')
- Array contents: ['M', 'I', 'D', 'T', 'E', 'R', 'M', '-']
- Unused space (indicated by '-') follows the last character.
```plaintext
first character last character
a[] -> M I D T E R M -
0 1 2 3 4 5 6 7
```
#### Performance Properties
Please mark any properties that apply:
- [ ] \( \Theta(1) \) time in the worst case for `charAt()`.
- [ ] \( \Theta(1) \) time in the worst case for `append`.
- [ ] Starting from an empty `StringBuilder`, any sequence of \( n \) `append` operations takes \( \Theta(n) \) time in the worst case.
- [ ] Starting from an empty `StringBuilder`, any sequence of \( n \) `append` and `prepend` operations takes \( \Theta(n) \) time in the worst case.
- [ ] Uses \( \Theta(n) \) memory in the worst case.
This overview is designed to assist students in
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
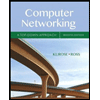
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
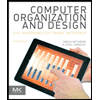
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
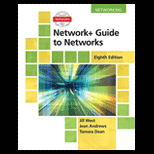
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
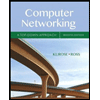
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
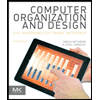
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
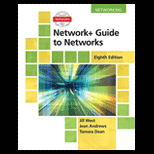
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
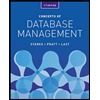
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
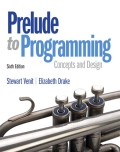
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
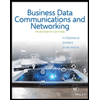
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY