(Java) Take a look
(Java)
Take a look at the following Person.java code:
import java.util.Scanner;
import java.io.*;
class Person {
publicStringname;
publicintage;
publicStringgender;
// add additional member
privateAddressaddress;
// default constructor
publicPerson(){
name="unkonwn";
age=0;
gender="NA";
address=newAddress();
}
// argument constructor
publicPerson(Stringname,intage,Stringgender,Addressaddress){
this.name=name;
this.age=age;
this.gender=gender;
this.address=address;
}
publicvoidgreeting(){
System.out.println("Hi, my name is " + name + "!");
}
publicvoidgreeting(StringotherName){
System.out.println("Hi, " + otherName + "!");
}
publicvoidsetName(Stringname){
this.name=name;
}
publicvoidsetAge(intage){
this.age=age;
}
publicvoidsetGender(Stringgender){
this.gender=gender;
}
publicStringgetName(){
returnthis.name;
}
publicintgetAge(){
returnthis.age;
}
publicStringgetGender(){
returnthis.gender;
}
publicAddressgetAddress(){
// fill in method body here
returnaddress;
}
publicvoidsetAddress(Addressa){
// fill in method body here
address=a;
}
@Override
publicStringtoString(){
return"Name: "+name+"\nAge: "+age+"\nGender: "+gender+"\nAddress: "+address;// fill in here!;
}
}
- Let's update it to contain a copy constructor, as follows:
- It takes in a Person object as a parameter
- It assigns this to have the same age, name and gender as the parameter
- It assigns address a deep copy of the address field. In other words, you will need to call the Address constructor to achieve the deep copy.
- See example above of a deep copy in the Copy Constructor portion of the lesson notes.
- Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file), and update the incomplete line.
/**
* PersonTest.java, Activity 9.1
* @author
* CIS 36B
*/
import java.util.Scanner;
public class PersonTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String name, gender, street;
int age, number;
Person person1;
System.out.print("Welcome!\n\nEnter your name: ");
name = input.nextLine();
System.out.print("Enter your age: ");
age = input.nextInt();
System.out.print("Enter your gender: ");
gender = input.next();
System.out.print("Enter the street number of your address: ");
number = input.nextInt();
System.out.print("Enter the name of your street: ");
input.nextLine();
street = input.nextLine();
Address address = new Address(number, street);
person1 = new Person(name, age, gender, address);
Person person2 = //fill in line to call copy constructor here
System.out.print("\nEnter the name of another person with your age and gender: ");
name = input.nextLine();
System.out.print("Enter " + name + "'s street number: ");
number = input.nextInt();
System.out.print("Enter " + name + "'s street name: ");
input.nextLine();
street = input.nextLine();
person2.setName(name);
person2.getAddress().setNumber(number);
person2.getAddress().setStreet(street);
System.out.println("\nYour Summary:\n" + person1);
System.out.println("\nAnother student with your "
+ "same age and gender: \n" + person2);
input.close();
}
}
- When you get the correct output (as shown below), upload Address.java, Person.java and PersonTest.java to Canvas
Sample Output (Note that user input may vary):
Welcome!
Enter your name: Wen Li
Enter your age: 23
Enter your gender: Male
Enter the street number of your address: 2141
Enter the name of your street: W. Elm Ave
Enter the name of another person with your age and gender: C.J. Nguyen
Enter C.J. Nguyen's street number: 4567
Enter C.J. Nguyen's street name: S. 7th St
Your Summary:
Name: Wen Li
Age: 23
Gender: Male
Address: 2141 W. Elm Ave
Another student with your same age and gender:
Name: C.J. Nguyen
Age: 23
Gender: Male
Address: 4567 S. 7th St

I have answered this question in step 2.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

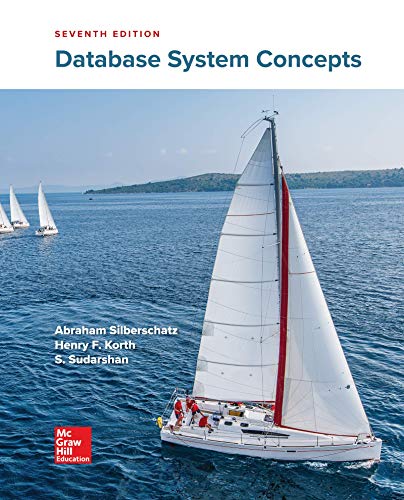
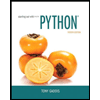
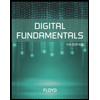
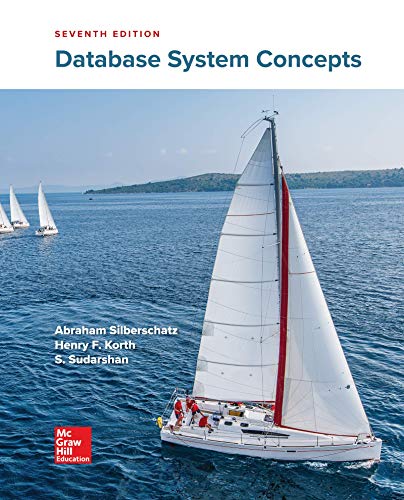
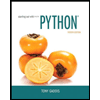
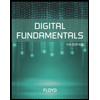
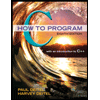
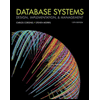
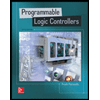