Java Programming language Help please.
Java
Help please.



1 public class SinglyLinked List<E>
{
(nested Node class goes here)
......
14
15 // instance variables of the SinglyLinked List private Node<E> head = null;
private Node<E> tail = null; private int size = 0;
16 // head node of the list (or null if empty) last node of the list (or null if empty) number of nodes in the list
17 // constructs an initially empty list public SinglyLinkedList()
{
}
// access methods public int size()
{
return size;
}
public boolean isEmpty()
{
return size == 0;
}
public E first()
{
// returns (but does not remove) the first element
if (isEmpty()) return null;
24 return head.getElement();
}
public E last()
{
// returns (but does not remove) the last element
if (isEmpty())
return null;
28 return tail.getElement();
}
// update methods public void addFirst(E e)
{
head = new Node<>(e, head); 0)
// adds element e to the front of the list create and link a new node
if (size == tail = head;
// special case: new node becomes tail also size++;
36
}
37
38 public void add Last(E e)
{
// adds element e to the end of the list
Node<E> newest = new Node<>(e, null);
// node will eventually be the tail
if (isEmpty())
39
40 head newest;
// special case: previously empty list
41
else
42
tail.setNext(newest);
new node after existing tail
43 tail newest;
// new node becomes the tail
44
size++;
45
}
46 public E removeFirst()
{
// removes and returns the first element
// nothing to remove
47
if (isEmpty())
return null;
E answer = head.getElement();
48 head.getNext();
// will become null
if list had only one node size--; == 0)
if (size)
tail = null;
// special case as list is now empty
return answer;
}
}
Step by step
Solved in 2 steps

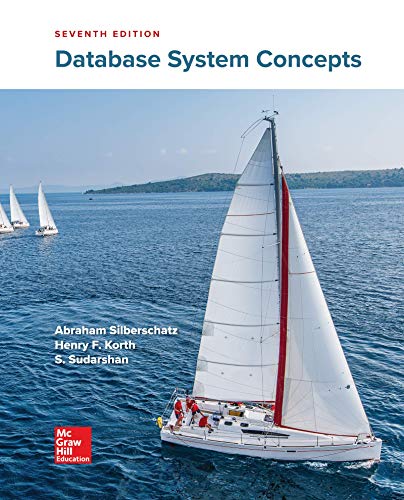
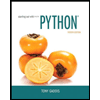
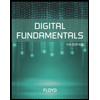
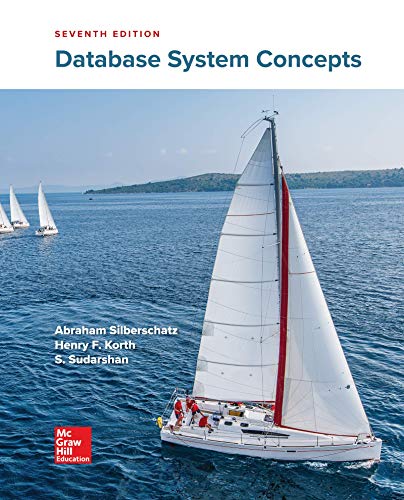
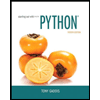
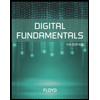
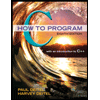
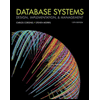
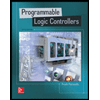