Java program In main method: Create 2-D Array, called m: the row size of array m is 15 ask user to input column size of array m fill all array elements as double numbers in the range of (7.0, 17.0) by using random object create 2-d array, called double [][]md = {{1,1,1},{2,0,1},{4,7,4}}; pass the above array m and md to call the following two methods MaxCol(m); MaxCol(md); System.out.println (“the average of array m is: ” + returnLast3RowAvg(m)); System.out.println (“the average of array md is: ” + returnLast3RowAvg(md)); Print out the largest column sum, and the average of the last 3 rows of array m and md. In MaxCol(double[][]array) method, find and print the largest column sum in the array. In returnLast3RowAvg(double[][]array) method, find the average of the elements in the last 3 rows in the array and return this average value.
Java program
In main method:
- Create 2-D Array, called m:
- the row size of array m is 15
- ask user to input column size of array m
- fill all array elements as double numbers in the range of (7.0, 17.0) by using random object
- create 2-d array, called double [][]md = {{1,1,1},{2,0,1},{4,7,4}};
- pass the above array m and md to call the following two methods
- MaxCol(m);
- MaxCol(md);
- System.out.println (“the average of array m is: ” + returnLast3RowAvg(m));
- System.out.println (“the average of array md is: ” + returnLast3RowAvg(md));
- Print out the largest column sum, and the average of the last 3 rows of array m and md.
In MaxCol(double[][]array) method, find and print the largest column sum in the array.
In returnLast3RowAvg(double[][]array) method, find the average of the elements in the last 3 rows in the array and return this average value.

import java.util.Scanner;
public class MyArrayTest {
public static void main(String[] args) {
//declaring 2D array
double m[][]=new double[15][];
Scanner sc=new Scanner(System.in);
//asking user for input
System.out.print("Enter Column size of array : ");
int n=sc.nextInt();
//populate m array by random values
for(int i=0;i<15;i++) {
m[i]=new double[n];
for(int j=0;j<n;j++) {
m[i][j]=(Math.random() * ((17.0 - 7.0) + 1)) + 7.0;
}
}
//create 2D array
double [][]md={{1,1,1},{2,0,1},{4,7,4}};
//calling methods and print result
MaxCol(m);
MaxCol(md);
System.out.println("Average of Last three Row element Array m : "+returnLast3RowAvg(m));
System.out.println("Average of Last three Row element Array md : "+returnLast3RowAvg(md));
}
public static void MaxCol(double [][]array) {
//creating variable to hold max value
double max=0;
//iterating from column by row order
for(int j=0;j<array[0].length;j++) {
double sum=0;
for(int i=0;i<array.length;i++)
//find sum
sum=sum+array[i][j];
//check for sum is greater than max
if(max<sum)
max=sum;
}
//print result
System.out.println("Largest Column sum is : "+max);
}
public static double returnLast3RowAvg(double[][]array) {
//creating variable to hold sum value
double sum=0;
int k=0;
//this loop will run for three rows only
for(int i=array.length-3;i<array.length;i++) {
for(int j=0;j<array[i].length;j++) {
//find sum
sum=sum+array[i][j];
k++;
}
}
//return average
return sum/k;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

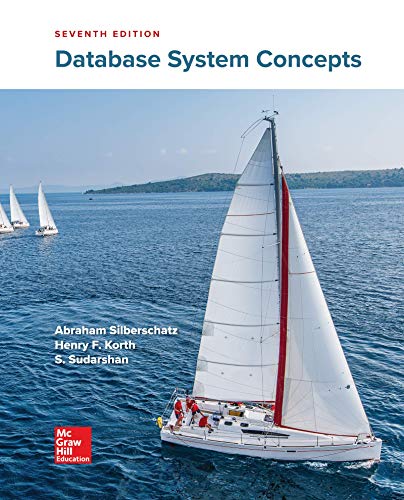
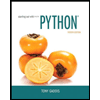
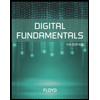
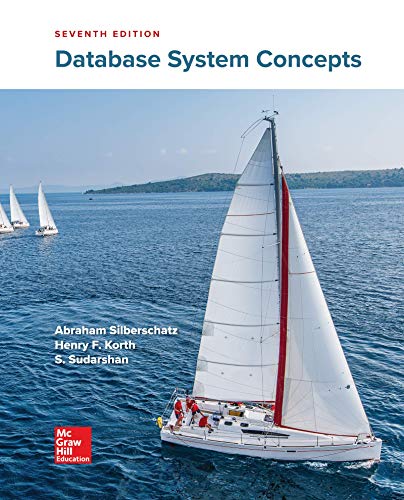
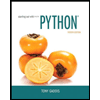
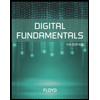
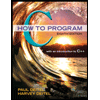
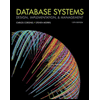
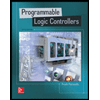