java Is there anything wrong with the following codes? If any fix them all. a. public class UnSafeClass { public static void unsafeMethod() throws Exception, RuntimeException { System.out.println("This is an unsafe method!"); } public static void main(String[] args) { try { unsafeMethod(); } catch (IOException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } catch (RuntimeException e) { e.printStackTrace(); } } } b. public class Nobody { public static void main(String[] args) { Somebody person = new Somebody() { String name = "nobody"; public void printName() { System.out.println("I am " + name); } public void printName(String name) { System.out.println("I am " + name); } }; person.printName("nobody"); } } interface Somebody { public void printName(); } c. public class SafeClass { public static double sqrt(double number) throws Exception { if (number < 0) throw new Exception("Negative number"); else return Math.sqrt(number); } public static void main(String[] args) { System.out.println(sqrt(3)); } }
java
Is there anything wrong with the following codes? If any fix them all.
a.
public class UnSafeClass {
public static void unsafeMethod() throws Exception,
RuntimeException {
System.out.println("This is an unsafe method!");
}
public static void main(String[] args) {
try {
unsafeMethod();
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} catch (RuntimeException e) {
e.printStackTrace();
}
}
}
b.
public class Nobody {
public static void main(String[] args) {
Somebody person = new Somebody() {
String name = "nobody";
public void printName() {
System.out.println("I am " + name);
}
public void printName(String name) {
System.out.println("I am " + name);
}
};
person.printName("nobody");
}
}
interface Somebody {
public void printName();
}
c.
public class SafeClass {
public static double sqrt(double number) throws Exception {
if (number < 0) throw new Exception("Negative number");
else return Math.sqrt(number);
}
public static void main(String[] args) {
System.out.println(sqrt(3));
}
}

Step by step
Solved in 3 steps with 4 images

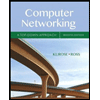
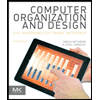
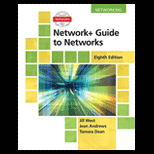
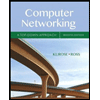
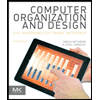
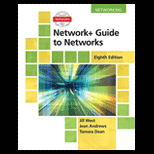
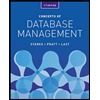
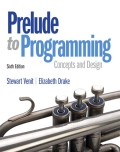
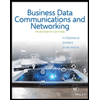