Java Given a 2 dimensional array, write a method to return the average of each row. The result must be returned as a one dimensional array. The array may be ragged. For example: int[][] my2DArray = { {2,5,5}, {2,6,8,4}, {6,5,2,3} } The result should be: int[] myArrayAverage = { 4, //Average of {2,5,5} 5, //Average of {2,6,8,4} 4 //Average of {6,5,2,3} } Note: You only need to write down the code inside the myMethod() code block public class CST1201Test3 { public static void main(String[] args) { int[][] my2DArray = { {2,5,5}, {2,6,8,4}, {6,5,2,3} }; int[] myArrayAverage = myMethod(my2DArray)); } static int[] myMethod(int[][] numbers) { // your answer } }
Java
Given a 2 dimensional array, write a method to return the average of each row.
The result must be returned as a one dimensional array. The array may be ragged.
For example:
int[][] my2DArray = {
{2,5,5},
{2,6,8,4},
{6,5,2,3}
}
The result should be:
int[] myArrayAverage = {
4, //Average of {2,5,5}
5, //Average of {2,6,8,4}
4 //Average of {6,5,2,3}
}
Note: You only need to write down the code inside the myMethod() code block
public class CST1201Test3
{
public static void main(String[] args)
{
int[][] my2DArray = {
{2,5,5},
{2,6,8,4},
{6,5,2,3}
};
int[] myArrayAverage = myMethod(my2DArray));
}
static int[] myMethod(int[][] numbers)
{
// your answer
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

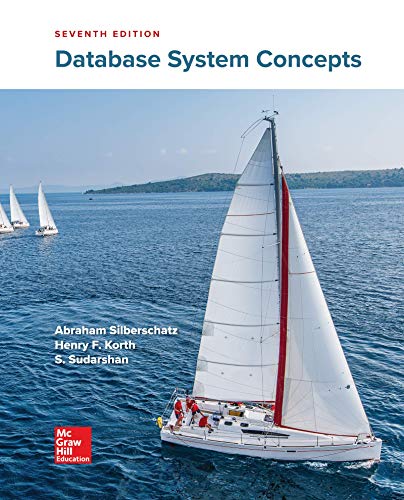
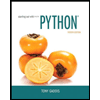
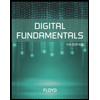
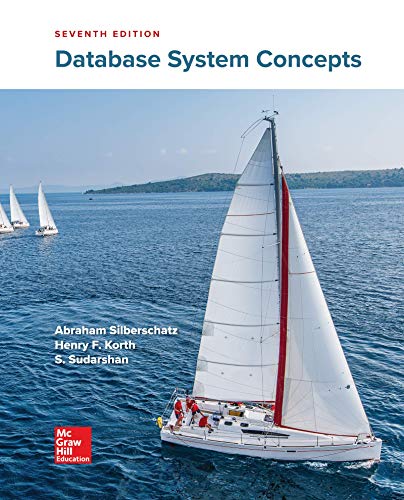
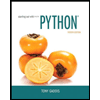
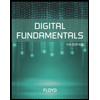
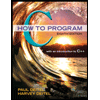
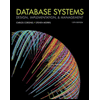
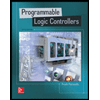