JAVA Create a class called Quadratic for representing a one-variable quadratic expression of the form: ax2 + bx + c a,b and c here are the coefficients. The class should contain the following methods: * A constructor that accepts values for a, b, and c. * public double getA() * public double getB() * public double getC() * public double evaluate (int x) * will return the value of the expression at point x * public double discriminant() - that will return (b2 - 4ac) * public boolean isImaginaryRoots() - roots are imaginary if (b2 - 4ac) < 0 * public boolean isRealRoots() - roots are real if (b2 - 4ac) >= 0 // these methods can only be invoked if the roots are not imaginary * public float firstRoot() * public float secondRoot() * public boolean isPerfectSquare(); // If the first and second roots are equal * Try to override the toString methods * Write a sample main program that will work as shown below. Example output: coefficient a: 4 coefficient b: 4 coefficient c: 1 Quadratic expression: 4x2 + 4x + 1 The roots are real: x1 = -0.5 ; x2 = -0.5 It is a perfect square. Evaluating the expression: Enter x: 2 Result : 25 coefficient a: 2 coefficient b: 5 coefficient c: 10 Quadratic expression: 2x2 + 5x + 10 The roots are imaginary. Evaluating the expression: Enter x: 1 Result : 17 Enter coefficient a: 1 Enter coefficient b: 0 Enter coefficient c: -1 Quadratic expression: x2 + 0x + -1 The roots are real. x1 = -1 ; x = 1 It is not a perfect square. Evaluating the expression: Enter x: 5 Result : 24
JAVA
Create a class called Quadratic for representing a one-variable quadratic expression of the form:
ax2 + bx + c
a,b and c here are the coefficients.
The class should contain the following methods:
* A constructor that accepts values for a, b, and c.
* public double getA()
* public double getB()
* public double getC()
* public double evaluate (int x)
* will return the value of the expression at point x
* public double discriminant()
- that will return (b2 - 4ac)
* public boolean isImaginaryRoots()
- roots are imaginary if (b2 - 4ac) < 0
* public boolean isRealRoots()
- roots are real if (b2 - 4ac) >= 0
// these methods can only be invoked if the roots are not imaginary
* public float firstRoot()
* public float secondRoot()
* public boolean isPerfectSquare();
// If the first and second roots are equal
* Try to override the toString methods
* Write a sample main program that will work as shown below.
Example output:
coefficient a: 4
coefficient b: 4
coefficient c: 1
Quadratic expression: 4x2 + 4x + 1
The roots are real: x1 = -0.5 ; x2 = -0.5
It is a perfect square.
Evaluating the expression:
Enter x: 2
Result : 25
coefficient a: 2
coefficient b: 5
coefficient c: 10
Quadratic expression: 2x2 + 5x + 10
The roots are imaginary.
Evaluating the expression:
Enter x: 1
Result : 17
Enter coefficient a: 1
Enter coefficient b: 0
Enter coefficient c: -1
Quadratic expression: x2 + 0x + -1
The roots are real. x1 = -1 ; x = 1
It is not a perfect square.
Evaluating the expression:
Enter x: 5
Result : 24

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

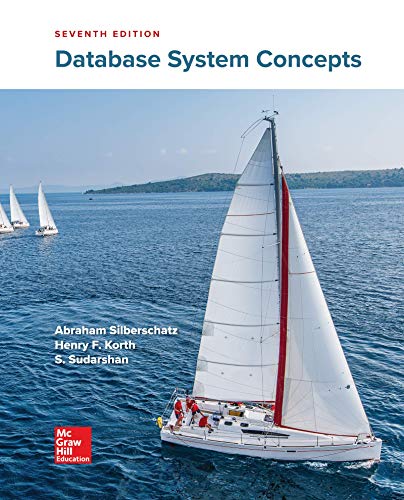
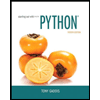
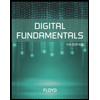
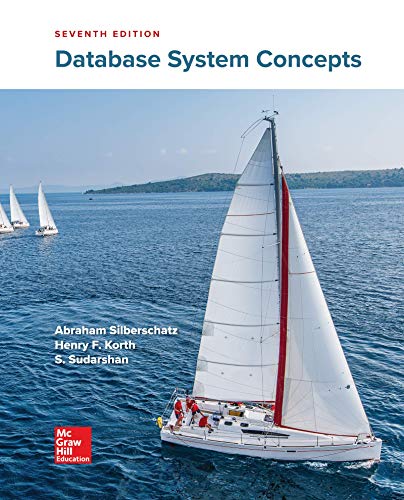
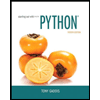
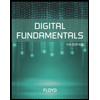
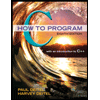
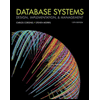
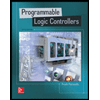