(Java) Activity 4.2 Open up Eclipse and create a new Java project called Sort with a class named Sort.java. Copy and paste the starter code below into the file and add your name in the comment at the top. /** * Sort.java * A program to sort a list of numbers * @author */ public class Sort { public static void main(String[] args) { int top10Scores[] = {95, 92, 88, 99, 93, 89, 94, 97, 100, 91}; System.out.println("Top 10 Exam Scores, Unsorted:"); printArray(top10Scores); //call bubbleSort here! System.out.println("\nAfter Sorting:"); printArray(top10Scores); }//end of main /** * Sorts an array of integers from smallest to largest * using the bubble sort algorithm * @param array the list of integer values */ public static void bubbleSort(int array[]) { return; } /** * Print an array of integers to the console * @param array the list of integer values */ public static void printArray(int[] array) { for (int i = 0; i < array.length; i++) { System.out.println((i + 1) + ". " + array[i]); } } //end of method printArray }//end of class Write the bubbleSort method using the more efficient version of bubble sort's pseudocode provided above. Make sure your bubbleSort method correctly sorts the given array. If you get stuck, post a question on Piazza. When finished, upload your Sort.java file
(Java)
Activity 4.2
- Open up Eclipse and create a new Java project called Sort with a class named Sort.java.
- Copy and paste the starter code below into the file and add your name in the comment at the top.
/**
* Sort.java
* A
* @author
*/
public class Sort {
public static void main(String[] args) {
int top10Scores[] = {95, 92, 88, 99, 93, 89, 94, 97, 100, 91};
System.out.println("Top 10 Exam Scores, Unsorted:");
printArray(top10Scores);
//call bubbleSort here!
System.out.println("\nAfter Sorting:");
printArray(top10Scores);
}//end of main
/**
* Sorts an array of integers from smallest to largest
* using the bubble sort
* @param array the list of integer values
*/
public static void bubbleSort(int array[]) {
return;
}
/**
* Print an array of integers to the console
* @param array the list of integer values
*/
public static void printArray(int[] array) {
for (int i = 0; i < array.length; i++) {
System.out.println((i + 1) + ". " + array[i]);
}
} //end of method printArray
}//end of class
- Write the bubbleSort method using the more efficient version of bubble sort's pseudocode provided above.
- Make sure your bubbleSort method correctly sorts the given array.
- If you get stuck, post a question on Piazza.
- When finished, upload your Sort.java file

Step by step
Solved in 3 steps with 3 images

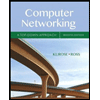
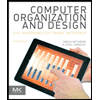
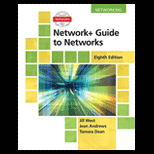
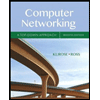
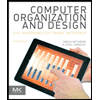
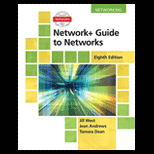
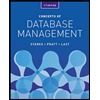
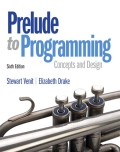
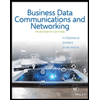