Jason opened a coffee shop at the beach and sells coffee in three sizes: small (9oz), medium (12oz), and large (15oz). The cost of one small cup is $1.75, one medium cup is $1.90, and one large cup is $2.00. Write a menu-driven program that will make the coffee shop operational. Your program should allow the user to do the following: a.Buy coffee in any size and in any number of cups. b.At any time show the total number of cups of each size sold. c.At any time show the total amount of coffee sold. d.At any time show the total money made. Analysis The buy coffee option allows the customer to select the number of cups in each of the sizes they want to order. When the customer has completed the order, an itemized receipt for that order is displayed.The other three options are used by the owner to check on the status of the coffee shop resources and revenue. The program is started when the coffee shop opens and shut down whenthe coffee shop closes,and the manager has gotten the final tally of the amount of revenue collectedand the amount of resources used. However, the manager may want to check on the resourcesand/orrevenueat any time while the coffee shop is open. The program providesinformation about the coffee shop over a number of hours. When an order is placed, the customer isasked what size of coffee is needed and how many cups of each chosen size. The customer is presented with a bill for that transaction. Each time an order is placed, the number of cups of each size sold isaccumulated so it can be used to calculate the total number of cups of each size sold, the total amount of coffee sold, and the total amount of money made when a request for one of those quantities is madeby the manager. As you analyze the problem it becomes clear that there are two main tasks in this problem, one to sell coffee to a customer and one to provide the manager with inventory and revenue information. While the purpose of the coffee selling task is to sell coffee to an individual customer, the information for the inventory and revenue reports is gathered each time an order is placed. Use reference parameters to communicate between the twotop leveltasks. Use functional decomposition to break each of the two top-leveltasksinto one or more cohesive sub tasks. Make the application menu driven. You may also want to use a menu for the sale of coffee. In addition to the code, provide atest plan in the form of scenarios.For the input, describethe interaction between the customer and the cashier or the manager. For the output, describe the outcome the program is expected to produce for each transaction. To simulate time passing, intersperse customer andmanager interaction to show how the inventory changes over time. To understand the data flows for this problem here is structure chart which also shows how data is moving to and from the input, output, and functions of the program. IPO analysis for each function int main(); Controls the processing of the coffee shop's main menu which contains cashier and manager functions Input - Program Input: User choice for which menu function to process (or to quit) Processing - Repeat until user selects "Quit" showMainMenu() Get menu selection Based on selection process one of: 1.Buy coffee (buyCoffee) (cashier) 2.Show cups sold (cupsSold) (manager) 3.Show coffee sold (coffeeSold) (manager) 4.Show money made (moneyMade) (manage) 5.Quit C++
Jason opened a coffee shop at the beach and sells coffee in three sizes: small (9oz), medium (12oz), and large (15oz). The cost of one small cup is $1.75, one medium cup is $1.90, and one large cup is $2.00. Write a menu-driven program that will make the coffee shop operational. Your program should allow the user to do the following:
a.Buy coffee in any size and in any number of cups.
b.At any time show the total number of cups of each size sold.
c.At any time show the total amount of coffee sold.
d.At any time show the total money made.
Analysis
The buy coffee option allows the customer to select the number of cups in each of the sizes they want to order. When the customer has completed the order, an itemized receipt for that order is displayed.The other three options are used by the owner to check on the status of the coffee shop resources and revenue. The program is started when the coffee shop opens and shut down whenthe coffee shop closes,and the manager has gotten the final tally of the amount of revenue collectedand the amount of resources used. However, the manager may want to check on the resourcesand/orrevenueat any time while the coffee shop is open.
The program providesinformation about the coffee shop over a number of hours. When an order is placed, the customer isasked what size of coffee is needed and how many cups of each chosen size. The customer is presented with a bill for that transaction. Each time an order is placed, the number of cups of each size sold isaccumulated so it can be used to calculate the total number of cups of each size sold, the total amount of coffee sold, and the total amount of money made when a request for one of those quantities is madeby the manager.
As you analyze the problem it becomes clear that there are two main tasks in this problem, one to sell coffee to a customer and one to provide the manager with inventory and revenue information. While the purpose of the coffee selling task is to sell coffee to an individual customer, the information for the inventory and revenue reports is gathered each time an order is placed. Use reference parameters to communicate between the twotop leveltasks. Use functional decomposition to break each of the two top-leveltasksinto one or more cohesive sub tasks.
Make the application menu driven. You may also want to use a menu for the sale of coffee.
In addition to the code, provide atest plan in the form of scenarios.For the input, describethe interaction between the customer and the cashier or the manager. For the output, describe the outcome the program is expected to produce for each transaction.
To simulate time passing, intersperse customer andmanager interaction to show how the inventory changes over time.
To understand the data flows for this problem here is structure chart which also shows how data is moving to and from the input, output, and functions of the program.
IPO analysis for each function
int main();
Controls the processing of the coffee shop's main menu which contains cashier and manager functions
Input -
Program Input: User choice for which menu function to process (or to quit)
Processing -
Repeat until user selects "Quit"
showMainMenu()
Get menu selection
Based on selection process one of:
1.Buy coffee (buyCoffee) (cashier)
2.Show cups sold (cupsSold) (manager)
3.Show coffee sold (coffeeSold) (manager)
4.Show money made (moneyMade) (manage)
5.Quit
C++


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

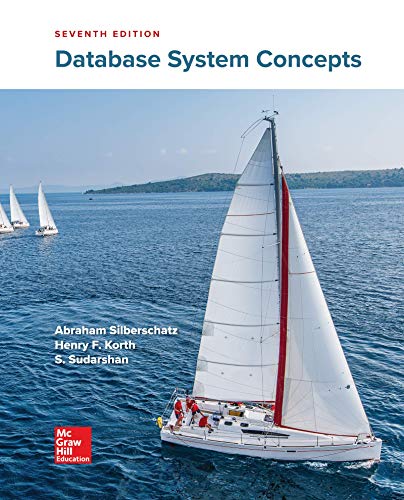
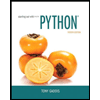
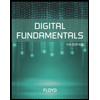
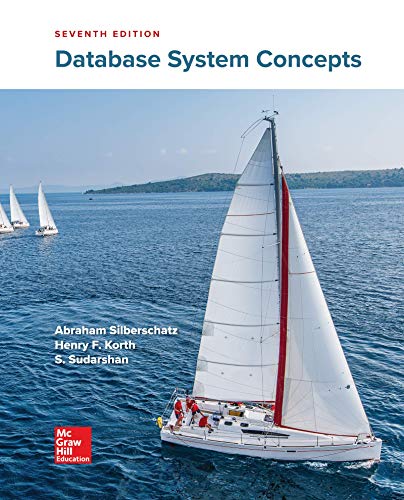
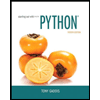
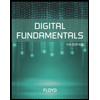
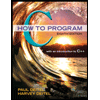
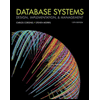
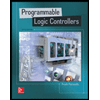