iven the base class Instrument, define a derived class StringInstrument for string instruments. class Instrument: def __init__(self, name, manufacturer, year_built, cost): self.name = name self.manufacturer = manufacturer self.year_built = year_built self.cost = cost def print_info(self): print('Instrument Information:') print(' Name:', self.name) print(' Manufacturer:', self.manufacturer) print(' Year built:', self.year_built) print(' Cost:', self.cost) class StringInstrument(Instrument): # TODO: Define constructor with attributes: # name, manufacturer, year_built, cost, num_strings, num_frets if __name__ == "__main__": instrument_name = input() manufacturer_name = input() year_built = int(input()) cost = int(input()) string_instrument_name = input() string_manufacturer = input() string_year_built = int(input()) string_cost = int(input()) num_strings = int(input()) num_frets = int(input()) my_instrument = Instrument(instrument_name, manufacturer_name, year_built, cost) my_string_instrument = StringInstrument(string_instrument_name, string_manufacturer, string_year_built, string_cost, num_strings, num_frets) my_instrument.print_info() my_string_instrument.print_info() print(' Number of strings:', my_string_instrument.num_strings) print(' Number of frets:', my_string_instrument.num_frets)
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Given the base class Instrument, define a derived class StringInstrument for string instruments.
class Instrument:
def __init__(self, name, manufacturer, year_built, cost):
self.name = name
self.manufacturer = manufacturer
self.year_built = year_built
self.cost = cost
def print_info(self):
print('Instrument Information:')
print(' Name:', self.name)
print(' Manufacturer:', self.manufacturer)
print(' Year built:', self.year_built)
print(' Cost:', self.cost)
class StringInstrument(Instrument):
# TODO: Define constructor with attributes:
# name, manufacturer, year_built, cost, num_strings, num_frets
if __name__ == "__main__":
instrument_name = input()
manufacturer_name = input()
year_built = int(input())
cost = int(input())
string_instrument_name = input()
string_manufacturer = input()
string_year_built = int(input())
string_cost = int(input())
num_strings = int(input())
num_frets = int(input())
my_instrument = Instrument(instrument_name, manufacturer_name, year_built, cost)
my_string_instrument = StringInstrument(string_instrument_name, string_manufacturer, string_year_built, string_cost, num_strings, num_frets)
my_instrument.print_info()
my_string_instrument.print_info()
print(' Number of strings:', my_string_instrument.num_strings)
print(' Number of frets:', my_string_instrument.num_frets)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

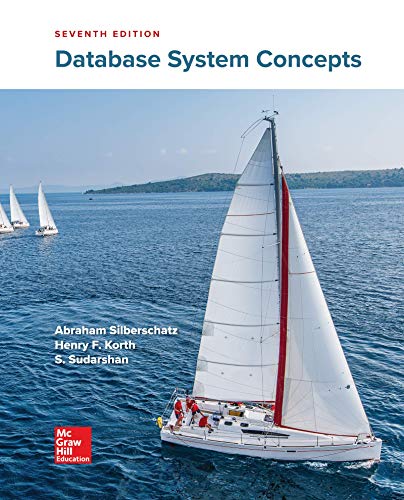
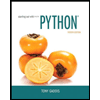
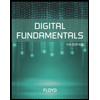
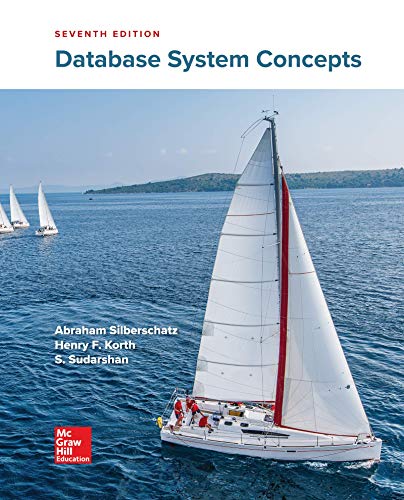
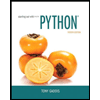
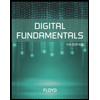
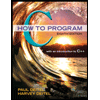
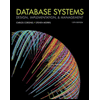
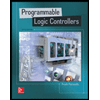