modify the python code below to 1. receive a command-line argument indicating the number of games of craps to execute and use two lists to track the total numbers of games won and lost on the first roll, second roll, third roll, etc. Summarize the results as follows: 2. Display a horizontal bar plot indicating how many games are won and how many are lost on the first roll, second roll, third roll, etc. Since the game could continue indefinitely, you might track wins and losses through the first dozen rolls (of a pair of dice), then maintain two counters that keep track of wins and losses after 12 rolls—no matter how long the game gets. Create separate bars for wins and losses. Therefore, the plot should have 13 horizontal bars for wins, and 13 horizontal bars for losses. Part (2) should have output with matplitlib the code to modify import random def roll_dice(): die1 = random.randrange(1, 7) die2 = random.randrange(1, 7) return (die1, die2) def display_dice(dice): die1, die2 = dice print(f'Player rolled {die1} + {die2} = {sum(dice)}') die_values = roll_dice() display_dice(die_values) sum_of_dice = sum(die_values) if sum_of_dice in (7, 11): game_status = 'WON' elif sum_of_dice in (2, 3, 12): game_status = 'LOST' else: game_status = 'CONTINUE' my_point = sum_of_dice print('Point is', my_point) while game_status == 'CONTINUE': die_values = roll_dice() display_dice(die_values) sum_of_dice = sum(die_values) if sum_of_dice == my_point: game_status = 'WON' elif sum_of_dice == 7: game_status = 'LOST' if game_status == 'WON': print('Player wins') else: print('Player loses')
modify the python code below to
1. receive a command-line argument indicating the number of games of craps to execute and use two lists to track the total numbers of games won and lost on the first roll, second roll, third roll, etc.
Summarize the results as follows:
2. Display a horizontal bar plot indicating how many games are won and how many are lost on the first roll, second roll, third roll, etc. Since the game could continue indefinitely, you might track wins and losses through the first dozen rolls (of a pair of dice), then maintain two counters that keep track of wins and losses after 12 rolls—no matter how long the game gets. Create separate bars for wins and losses. Therefore, the plot should have 13 horizontal bars for wins, and 13 horizontal bars for losses.
Part (2) should have output with matplitlib
the code to modify
import random
def roll_dice():
die1 = random.randrange(1, 7)
die2 = random.randrange(1, 7)
return (die1, die2)
def display_dice(dice):
die1, die2 = dice
print(f'Player rolled {die1} + {die2} = {sum(dice)}')
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice in (7, 11):
game_status = 'WON'
elif sum_of_dice in (2, 3, 12):
game_status = 'LOST'
else:
game_status = 'CONTINUE'
my_point = sum_of_dice
print('Point is', my_point)
while game_status == 'CONTINUE':
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice == my_point:
game_status = 'WON'
elif sum_of_dice == 7:
game_status = 'LOST'
if game_status == 'WON':
print('Player wins')
else:
print('Player loses')

As per the given question, we need to modify given code such that the code receives number of games of craps to execute as command-line argument and keeps tracks of numbers of games won and lost on each roll and then plots the horizontal bar plot for 13 rolls.
Step by step
Solved in 4 steps with 3 images

Is there a way to make it so this code becomes dynamic bar chart using from matplotlib import animation.
When ever I run the code it give me a
n=int(sys.argv[1])
IndexError: list index out of range
How do I fix it?
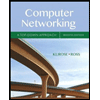
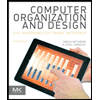
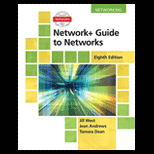
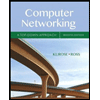
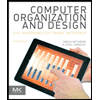
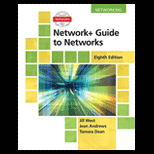
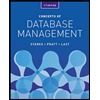
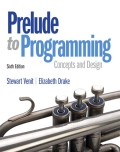
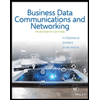