is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both doubles) as input, and output the gas cost les, 75 miles, and 500 miles. ut each floating-point value with two digits after the decimal point, which can be achieved as follows: tem.out.printf("%.2f", yourValue); e output ends with a newline.
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Java
Code a
![### LAB: Driving Costs
Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both doubles) as input, and output the gas cost for 20 miles, 75 miles, and 500 miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
```java
System.out.printf("%.2f", yourValue);
```
The output ends with a newline.
#### Example
If the input is:
```
20.0 3.1599
```
the output is:
```
3.16 11.85 79.00
```
Note: Real per-mile cost would also include maintenance and depreciation.
#### Graphical Explanation
There is a section for "LAB ACTIVITY" indicating the current activity (2.20.1: LAB: Driving costs) and a scripting environment showing the beginning of the Java code:
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
...
}
}
```
In this scenario, you would need to complete writing the Java program to perform the specified calculations.
---
This tabulation helps students to not only understand the theoretical aspects of programming but also gives them practical exposure to coding and handling real-world problems.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc8f23c41-b0a2-4b7f-b455-de9ffcd4a71a%2Fac337d9c-8c79-4890-95c2-ce2885691f9f%2Frhe392o_processed.jpeg&w=3840&q=75)
![### LAB Activity: Driving Costs
#### Lab Activity 2.20.1: LAB: Driving Costs
In this activity, you will be working on calculating driving costs using a Java program. Below is the initial code provided for you to start with:
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
// Your code will go here
}
}
```
- The program begins with importing the `Scanner` class from the `java.util` package, which will allow you to take input from the user.
- The `LabProgram` class is defined as `public`, making it accessible from outside its package. Inside this class, there is a `main` method, which serves as the entry point of the Java application.
- The body of the `main` method is currently empty, and you will need to add the logic to calculate the driving costs.
### Instructions:
1. **Import the Scanner Class**: This allows you to read input from standard input (keyboard). This has already been done for you in the template.
2. **Initialize the Scanner**:
```java
Scanner scnr = new Scanner(System.in);
```
3. **Read user inputs** for the following:
- Cost of gas per gallon
- Miles per gallon the car gets
- Driving distance in miles
4. **Calculate the cost of driving** for the entered distance:
- Use the formula `TotalCost = (Distance / MilesPerGallon) * CostPerGallon`
5. **Print the result** in a user-friendly format.
### Example Code Addition:
Here's an example to guide you:
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
// Get inputs
System.out.println("Enter cost per gallon:");
double costPerGallon = scnr.nextDouble();
System.out.println("Enter miles per gallon:");
double milesPerGallon = scnr.nextDouble();
System.out.println("Enter distance in miles:");
double distance = scnr.nextDouble();
// Calculate total cost
double totalCost = (distance / milesPerGallon) * costPerGallon;
// Print the result
System.out.printf("Cost of driving %.2f miles: $%.2f\n", distance,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc8f23c41-b0a2-4b7f-b455-de9ffcd4a71a%2Fac337d9c-8c79-4890-95c2-ce2885691f9f%2Fg38bw8k_processed.jpeg&w=3840&q=75)

Step by step
Solved in 2 steps with 1 images

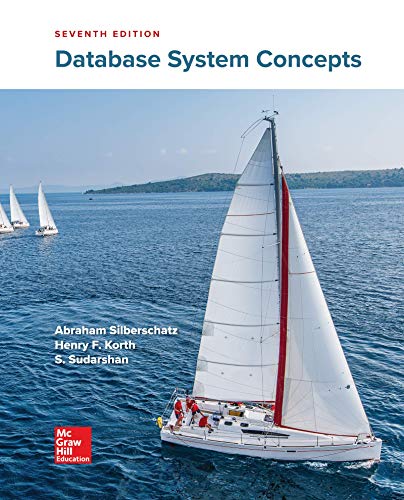
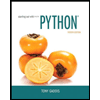
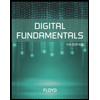
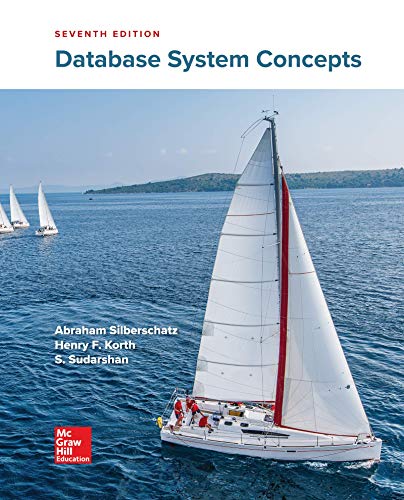
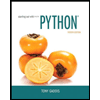
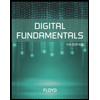
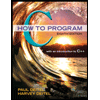
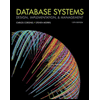
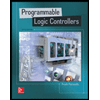