Integer numVals is read from input representing the number of floating-point values to be read next. Use a loop to read the remaining floating-point values from input. For each floating-point value read, output "Value read: " followed by the value. Then, output "Lowest:" followed by the lowest of the floating-point values read. End each output with a newline. Ex: If the input is:
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
![## Challenge Activity: Find Extreme Value
### Task Description
You need to read a certain number of floating-point values and determine the lowest of them. Here is how the program should work:
1. An integer, `numVals`, is read from input, indicating how many floating-point values will follow.
2. Use a loop to read the specified number of floating-point values.
3. For each value, output: `Value read:` followed by the actual value.
4. At the end, output `Lowest:` followed by the minimum value among those read.
**Example:**
If the input is:
```
2
86.4 -62.8
```
then the output should be:
```
Value read: 86.4
Value read: -62.8
Lowest: -62.8
```
**Note:** All floating-point values are of type `double`.
### Java Code Outline
Here is a basic structure to get you started on the implementation:
```java
import java.util.Scanner;
public class LowestValues {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int numVals;
double inValue;
double lowestVal = 0.0;
numVals = scnr.nextInt();
// Your code goes here
}
}
```
### Explanation
The task involves reading a sequence of floating-point numbers and identifying the smallest among them. The example provided illustrates the logic by reading the number of values to process (`2` in this case) and subsequently handling the values `86.4` and `-62.8`. The output confirms the values read and clearly identifies `-62.8` as the lowest value.
Carefully implement the logic to handle loops and conditions correctly to compare and store the minimum value as you process each input.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9f8d83e6-c87f-4567-a9a5-747d584a2169%2F0e180226-2b5f-4894-9ef9-71863bd89424%2Flk8hlir_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

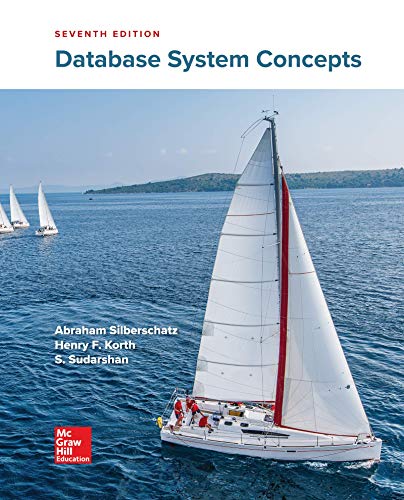
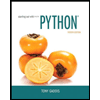
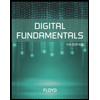
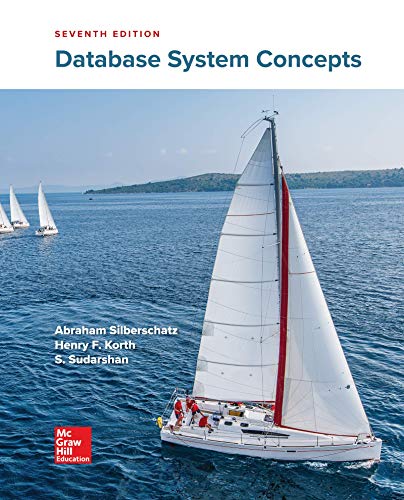
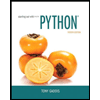
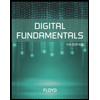
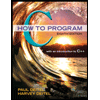
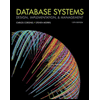
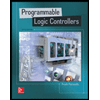