Instructions: Using the game below, you will update it to utilize the following: File writing and reading Modify the game to utilize file storage so that information is stored during gameplay and/or between running the program. Ideas: You might keep track of a high score, or save the gamestate so that you can continue with your game after leaving the program and then running the program later. It's up to you to decide how you want to do file writing and reading. Program: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary header files #include #include "GamePurse.h" #include #include using namespace std; int main(){ // create the object of GamePurse class GamePurse dice(100); int amt=1; // seed the random generator srand(time(0)); // to play the dice game repeatedly while(amt!=0){ cout<<"Welcome to the diceware game. You have 100 in your game purse."<>amt; // exit condition if(amt==0){ break; } // to to bet only a positive amount to play a game. while(amt<0){ cout<<"Enter a bet amount to play (0 means exit the program):"<>amt; } // generate the dice randomly int comp_dice = rand()%5 + 1; int user_dice = rand()%5 + 1; cout<<"Your dice is "<=comp_dice){ cout<<"You won "<< amt <<" dollars!"<
Instructions:
- Using the game below, you will update it to utilize the following:
- File writing and reading
- Modify the game to utilize file storage so that information is stored during gameplay and/or between running the
program .- Ideas: You might keep track of a high score, or save the gamestate so that you can continue with your game after leaving the program and then running the program later.
- It's up to you to decide how you want to do file writing and reading.
- Modify the game to utilize file storage so that information is stored during gameplay and/or between running the
- File writing and reading
Program:
File GamePurse.h:
class GamePurse
{
// data
int purseAmount;
public:
// public functions
GamePurse(int);
void Win(int);
void Loose(int);
int GetAmount();
};
File GamePurse.cpp:
#include "GamePurse.h"
// constructor initilaizes the purseAmount variable
GamePurse::GamePurse(int amount){
purseAmount = amount;
}
// function definations
// add a winning amount to the purseAmount
void GamePurse:: Win(int amount){
purseAmount+= amount;
}
// deduct an amount from the purseAmount.
void GamePurse:: Loose(int amount){
purseAmount-= amount;
}
// return the value of purseAmount.
int GamePurse::GetAmount(){
return purseAmount;
}
File main.cpp:
// include necessary header files
#include <stdlib.h>
#include "GamePurse.h"
#include<iostream>
#include<time.h>
using namespace std;
int main(){
// create the object of GamePurse class
GamePurse dice(100);
int amt=1;
// seed the random generator
srand(time(0));
// to play the dice game repeatedly
while(amt!=0){
cout<<"Welcome to the diceware game. You have 100 in your game purse."<<endl;
cout<<"Enter a bet amount to play (0 means exit the program):"<<endl;
cin>>amt;
// exit condition
if(amt==0){
break;
}
// to to bet only a positive amount to play a game.
while(amt<0){
cout<<"Enter a bet amount to play (0 means exit the program):"<<endl;
cin>>amt;
}
// generate the dice randomly
int comp_dice = rand()%5 + 1;
int user_dice = rand()%5 + 1;
cout<<"Your dice is "<<user_dice<<endl;
cout<<"Computer dice is "<<comp_dice<<endl;
// update the purse amount
if(user_dice>=comp_dice){
cout<<"You won "<< amt <<" dollars!"<<endl;
dice.Win(amt);
}else{
cout<<"You lost "<< amt <<" dollars!"<<endl;
dice.Loose(amt);
}
cout<<"Your game purse now has "<< dice.GetAmount()<<" dollars"<<endl<<endl;
}
// exit statements
cout<<"You choose to exit the program. Thank you and goodbye."<<endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

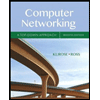
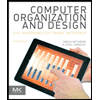
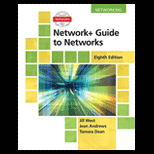
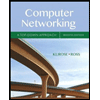
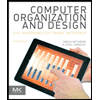
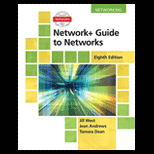
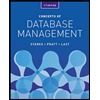
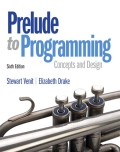
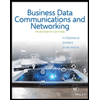