Instructions Sahara desert explorers call us for help once again. They want to know some statistics about the temperature in Sahara, but sometimes their thermometer fails to record the proper temperature. Help them to find which temperature is from correct recordings and which is broken and calculate statistics on given data. During the night, the temperature in Sahara varies from -4 to -10 C. During the day, the temperature varies from 20 to 50 C. You can use (copy/paste here) the previously-defined function check_input(temperature) that will return True if this temperature can be from Sahara and False if it is from a broken thermometer (i.e., outside of the expected range). This time, scientists need to be able to check more than 5 observations, and since their readings might vary from day to day, they hope that you can help, if they tell you how many measurements they have. Write a program to use the check_input to create a list of valid temperatures and compute their statistics: 1. Create an empty list, where you will store the "possible" temperatures. Let's say temperatures list. 2. Read and store the total number of measurements from the user. 3. Repeat the following actions as many times, as was input in the previous step. 3.1. Read the value from user (use input()) 3.2. Check it with your function 3.3. If the function tells that it is a possible temperature for Sahara - add this value to the temperatures list. 4. Find the minimum, maximum, and average temperature in temperatures and print it to match the example below:
Instructions Sahara desert explorers call us for help once again. They want to know some statistics about the temperature in Sahara, but sometimes their thermometer fails to record the proper temperature. Help them to find which temperature is from correct recordings and which is broken and calculate statistics on given data. During the night, the temperature in Sahara varies from -4 to -10 C. During the day, the temperature varies from 20 to 50 C. You can use (copy/paste here) the previously-defined function check_input(temperature) that will return True if this temperature can be from Sahara and False if it is from a broken thermometer (i.e., outside of the expected range). This time, scientists need to be able to check more than 5 observations, and since their readings might vary from day to day, they hope that you can help, if they tell you how many measurements they have. Write a program to use the check_input to create a list of valid temperatures and compute their statistics: 1. Create an empty list, where you will store the "possible" temperatures. Let's say temperatures list. 2. Read and store the total number of measurements from the user. 3. Repeat the following actions as many times, as was input in the previous step. 3.1. Read the value from user (use input()) 3.2. Check it with your function 3.3. If the function tells that it is a possible temperature for Sahara - add this value to the temperatures list. 4. Find the minimum, maximum, and average temperature in temperatures and print it to match the example below:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### Example Input
```
5
-10
-20
20
48
21
```
### Example Output
```
The minimum value is -10,
the maximum value is 48,
the average value is 19.75.
```
### Example Input
```
2
30
32
```
### Example Output
```
The minimum value is 30,
the maximum value is 32,
the average value is 31.00.
```
### Hints!
- **Check out your solution from 5.20**, it can help simplify things for you.
- **To calculate the minimum and maximum**, you can search for the function that we've covered in the zyBooks "List" section. For average, think about `sum` and `len` functions.
- **Average** of some values is the sum of these values, divided by the number of values.

Transcribed Image Text:**Learning Objectives**
- Practice using a `for` loop to achieve a concrete number of iterations
- Use `input()` and `.append()` functions inside the loop
- Create a function to match the specifications
- Use the `if/else` statements to detect a range of values
- Use lists to store the results
- Write a program to get the user input and call the custom function to produce the desired output
**Instructions**
Sahara desert explorers call us for help once again. They want to know some statistics about the temperature in Sahara, but sometimes their thermometer fails to record the proper temperature. Help them to find which temperature is from correct recordings and which is broken and calculate statistics on given data.
During the night, the temperature in Sahara varies from -4 to -10 C. During the day, the temperature varies from 20 to 50 C. You can use (copy/paste here) the previously-defined function `check_input(temperature)` that will return `True` if this temperature can be from Sahara and `False` if it is from a broken thermometer (i.e., outside of the expected range).
This time, scientists need to be able to check more than 5 observations, and since their readings might vary from day to day, they hope that you can help if they tell you how many measurements they have.
Write a program to use the `check_input` to create a list of valid temperatures and compute their statistics:
1. Create an empty list, where you will store the "possible" temperatures. Let's say `temperatures` list.
2. Read and store the total number of measurements from the user.
3. Repeat the following actions as many times, as was input in the previous step.
1. Read the value from user (use `input()`)
2. Check it with your function
3. If the function tells that it is a possible temperature for Sahara - add this value to the `temperatures` list.
4. Find the minimum, maximum, and average temperature in `temperatures` and print it to match the example below:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
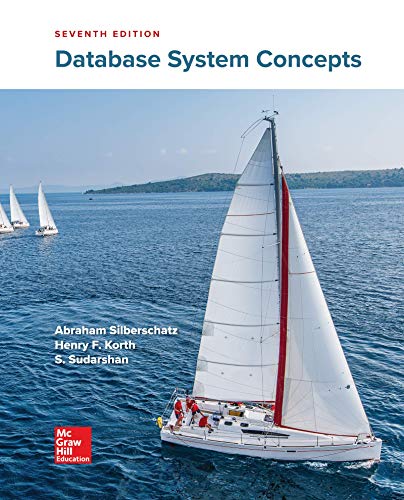
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
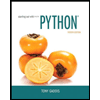
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
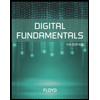
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
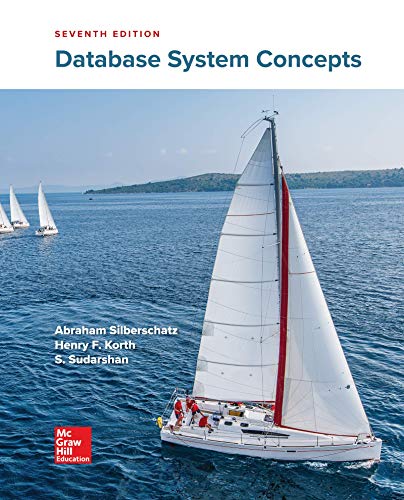
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
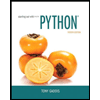
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
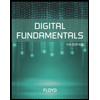
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
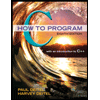
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
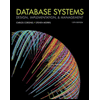
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
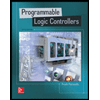
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education