Instruction: Create a program using linked list which continuously prompts user to enter integer scores until user keys in -1. Next, the program will calculate the average off all the numbers and count numbers (scores) that are larger than average. Display the average and number of scores higher than average. Sample output: Please enter your numbers to be averaged [-1 to quit]: 20 Please enter your numbers to be Please enter your numbers to be Please enter your numbers to be Please enter your numbers to be The average of 4 scores is 11 averaged [-1 to quit]: 12 averaged [-1 to quit]: 10 averaged [-1 to quit]: 2 averaged [-1 to quit]: -1 2 scores higher than the average. Follow the steps below: 1. Declare a struct called Data. In the struct, declare one integer called score, and one pointer called next. 2. In main function: (a) Declare one variable for average, of type double. (b) Declare one pointer called head and assign it to zero. (c) Create a while loop and assign its parameter to true. (d) In the loop: Create object called a Data as new Data. Instruct user with this instruction: "Please enter your numbers to be averaged [-1 to quit]: " Read score using cin. Remember to access score using aData (aData - > score) use break statement if score = -1. Assign next to head. - Assign head to aData. (e) Declare two integers called count and sum, assign 0 to both. (f) Declare a pointer called p of type Data(struct). (g) Create a for loop. For the loop header, put p = head; p; p = p->next (h) In the loop, put formula to sum up score. Use variable sum and p->score. Increase count by 1. (i) After the loop (outside), put formula for average. Display the average. Example: The average of (count) scores is (average). (j) Declare an integer called nGreater and assign it with 0. (k) Create a for loop. Follow step (g). (1) In the loop, put an if statement, where it increases nGreater to 1 if p->score > average. (m) Outside of loop, display score which is larger than average. Example: "(nGreater) scores higher than the average."
Instruction: Create a program using linked list which continuously prompts user to enter integer scores until user keys in -1. Next, the program will calculate the average off all the numbers and count numbers (scores) that are larger than average. Display the average and number of scores higher than average. Sample output: Please enter your numbers to be averaged [-1 to quit]: 20 Please enter your numbers to be Please enter your numbers to be Please enter your numbers to be Please enter your numbers to be The average of 4 scores is 11 averaged [-1 to quit]: 12 averaged [-1 to quit]: 10 averaged [-1 to quit]: 2 averaged [-1 to quit]: -1 2 scores higher than the average. Follow the steps below: 1. Declare a struct called Data. In the struct, declare one integer called score, and one pointer called next. 2. In main function: (a) Declare one variable for average, of type double. (b) Declare one pointer called head and assign it to zero. (c) Create a while loop and assign its parameter to true. (d) In the loop: Create object called a Data as new Data. Instruct user with this instruction: "Please enter your numbers to be averaged [-1 to quit]: " Read score using cin. Remember to access score using aData (aData - > score) use break statement if score = -1. Assign next to head. - Assign head to aData. (e) Declare two integers called count and sum, assign 0 to both. (f) Declare a pointer called p of type Data(struct). (g) Create a for loop. For the loop header, put p = head; p; p = p->next (h) In the loop, put formula to sum up score. Use variable sum and p->score. Increase count by 1. (i) After the loop (outside), put formula for average. Display the average. Example: The average of (count) scores is (average). (j) Declare an integer called nGreater and assign it with 0. (k) Create a for loop. Follow step (g). (1) In the loop, put an if statement, where it increases nGreater to 1 if p->score > average. (m) Outside of loop, display score which is larger than average. Example: "(nGreater) scores higher than the average."
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
data structure in c++
pls do use more // comments especially the linked list part
![Instruction: Create a program using linked list which continuously prompts user to enter integer
scores until user keys in -1. Next, the program will calculate the average off all the numbers and
count numbers (scores) that are larger than average. Display the average and number of scores
higher than average.
Sample output:
Please enter your numbers to be
Please enter your numbers to be
Please enter your numbers to be
Please enter your numbers to be
Please enter your numbers to be
The average of 4 scores is 11
2 scores higher than the average.
averaged [-1 to quit]: 20
averaged [-1 to quit]: 12
averaged [-1 to quit]: 10
averaged [-1 to quit]: 2
averaged [-1 to quit]: -1
Follow the steps below:
1. Declare a struct called Data. In the struct, declare one integer called score, and one
pointer called next.
2. In main function:
(a) Declare one variable for average, of type double.
(b) Declare one pointer called head and assign it to zero.
(c) Create a while loop and assign its parameter to true.
(d) In the loop:
Create object called aData as new Data.
Instruct user with this instruction: "Please enter your numbers to be
averaged [-1 to quit]: "
Read score using cin. Remember to access score using aData (aData -
> score)
use break statement if score = -1.
Assign next to head.
Assign head to aData.
(e) Declare two integers called count and sum, assign 0 to both.
(f) Declare a pointer called p of type Data(struct).
(g) Create a for loop. For the loop header, put p = head; p; p = p->next
(h) In the loop, put formula to sum up score. Use variable sum and p->score. Increase
count by 1.
(i) After the loop (outside), put formula for average. Display the average. Example:
The average of (count) scores is (average).
(j) Declare an integer called nGreater and assign it with 0.
(k) Create a for loop. Follow step (g).
(1) In the loop, put an if statement, where it increases nGreater to 1 ifp->score
> average.
(m) Outside of loop, display score which is larger than average. Example:
"(nGreater) scores higher than the average."](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1ddeca0-db0c-4fb2-a11d-d0dc67b17cbe%2Ff50e13fa-c1f6-4bf2-a5fe-a08e14f44a79%2F7aj82x_processed.png&w=3840&q=75)
Transcribed Image Text:Instruction: Create a program using linked list which continuously prompts user to enter integer
scores until user keys in -1. Next, the program will calculate the average off all the numbers and
count numbers (scores) that are larger than average. Display the average and number of scores
higher than average.
Sample output:
Please enter your numbers to be
Please enter your numbers to be
Please enter your numbers to be
Please enter your numbers to be
Please enter your numbers to be
The average of 4 scores is 11
2 scores higher than the average.
averaged [-1 to quit]: 20
averaged [-1 to quit]: 12
averaged [-1 to quit]: 10
averaged [-1 to quit]: 2
averaged [-1 to quit]: -1
Follow the steps below:
1. Declare a struct called Data. In the struct, declare one integer called score, and one
pointer called next.
2. In main function:
(a) Declare one variable for average, of type double.
(b) Declare one pointer called head and assign it to zero.
(c) Create a while loop and assign its parameter to true.
(d) In the loop:
Create object called aData as new Data.
Instruct user with this instruction: "Please enter your numbers to be
averaged [-1 to quit]: "
Read score using cin. Remember to access score using aData (aData -
> score)
use break statement if score = -1.
Assign next to head.
Assign head to aData.
(e) Declare two integers called count and sum, assign 0 to both.
(f) Declare a pointer called p of type Data(struct).
(g) Create a for loop. For the loop header, put p = head; p; p = p->next
(h) In the loop, put formula to sum up score. Use variable sum and p->score. Increase
count by 1.
(i) After the loop (outside), put formula for average. Display the average. Example:
The average of (count) scores is (average).
(j) Declare an integer called nGreater and assign it with 0.
(k) Create a for loop. Follow step (g).
(1) In the loop, put an if statement, where it increases nGreater to 1 ifp->score
> average.
(m) Outside of loop, display score which is larger than average. Example:
"(nGreater) scores higher than the average."
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
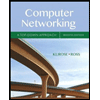
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
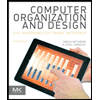
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
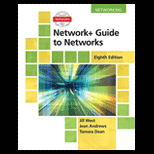
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
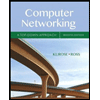
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
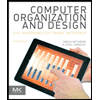
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
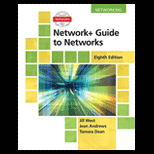
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
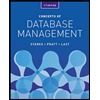
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
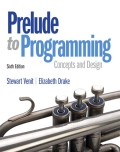
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
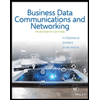
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY