Instead of using scanf to get the strings, use getchar() to get characters one by one, and check for the \0 to end the string. For this program please: #include<stdio.h> int main(){ int i; char first_name[50], last_name[50]; for(i=0;i<3;i++){ if(i==0){ printf("\n"); printf("First Name: "); scanf("%s",first_name); printf("Last Name: "); scanf("%s",last_name); printf("Full Name of First User is: %s %s\n",first_name,last_name); getchar(); } else if(i==1){ printf("\n"); printf("First Name: "); scanf("%s",first_name); printf("Last Name: "); scanf("%s",last_name); printf("Full Name of Second User is: %s %s\n",first_name,last_name); getchar(); } else{ printf("\n"); printf("First Name: "); scanf("%s",first_name); printf("Last Name: "); scanf("%s",last_name); printf("Full Name of Third User is: %s %s\n",first_name,last_name); getchar(); printf("\n"); } } return 0; }
Instead of using scanf to get the strings, use getchar() to get characters one by one, and check for the \0 to end the string.
For this program please:
#include<stdio.h>
int main(){
int i;
char first_name[50], last_name[50];
for(i=0;i<3;i++){
if(i==0){
printf("\n");
printf("First Name: ");
scanf("%s",first_name);
printf("Last Name: ");
scanf("%s",last_name);
printf("Full Name of First User is: %s %s\n",first_name,last_name);
getchar();
}
else if(i==1){
printf("\n");
printf("First Name: ");
scanf("%s",first_name);
printf("Last Name: ");
scanf("%s",last_name);
printf("Full Name of Second User is: %s %s\n",first_name,last_name);
getchar();
}
else{
printf("\n");
printf("First Name: ");
scanf("%s",first_name);
printf("Last Name: ");
scanf("%s",last_name);
printf("Full Name of Third User is: %s %s\n",first_name,last_name);
getchar();
printf("\n");
}
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

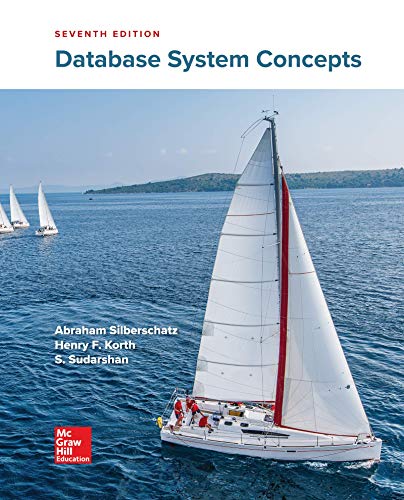
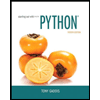
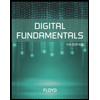
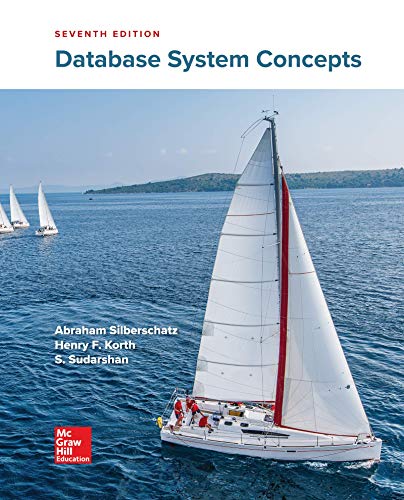
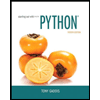
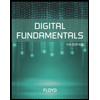
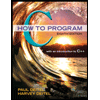
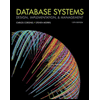
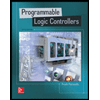