Input Your output Expected output Input Your output Expected output March 1, 1990 April 2 1995 7/15/20 December 13, 2003 -1 3-1-1990 4-2-1995 12-13-2003 3-1-1990 12-13-2003 April 3, 2014 June 10, 2013 May 5 2010 December 10, 2010 -1 4-3-2014 6-10-2013 5-5-2010 12-10-2010 4-3-2014 6-10-2013 12-10-2010
I need help with this Java Problem to output as described in the image below:
Parsing dates
Complete main() to read dates from input, one date per line. Each date's format must be as follows: March 1, 1990. Any date not following that format is incorrect and should be ignored. Use the substring() method to parse the string and extract the date. The input ends with -1 on a line alone. Output each correct date as: 3-1-1990.
Ex: If the input is:
March 1, 1990 April 2 1995 7/15/20 December 13, 2003 -1then the output is:
3-1-1990 12-13-2003Use the provided getMonthAsInt() method to convert a month string to an integer. If the month string is valid, an integer in the range 1 to 12 inclusive is returned, otherwise 0 is returned. Ex: getMonthAsInt("February") returns 2 and getMonthAsInt("7/15/20") returns 0.
//ParserDates
import java.util.Scanner;
public class DateParser {
public static int getMonthAsInt(String monthString) {
switch (monthString) {
case "January":
return 1;
case "February":
return 2;
case "March":
return 3;
case "April":
return 4;
case "May":
return 5;
case "June":
return 6;
case "July":
return 7;
case "August":
return 8;
case "September":
return 9;
case "October":
return 10;
case "November":
return 11;
case "December":
return 12;
default:
return 0;
}
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String inputDate = scnr.nextLine();
while (!inputDate.equals("-1")) {
String[] parts = inputDate.split(" ");
if (parts.length == 3) {
String month = parts[0];
int day = Integer.parseInt(parts[1].replaceAll(",", ""));
int year = Integer.parseInt(parts[2]);
int monthInt = getMonthAsInt(month);
if (monthInt != 0) {
System.out.printf("%d-%d-%d\n", monthInt, day, year);
}
}
inputDate = scnr.nextLine();
}
}
}

Unlock instant AI solutions
Tap the button
to generate a solution
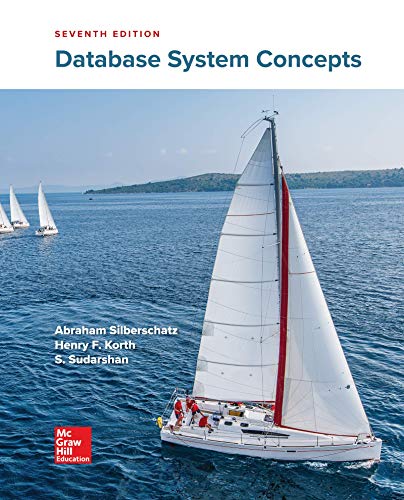
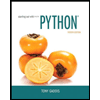
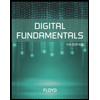
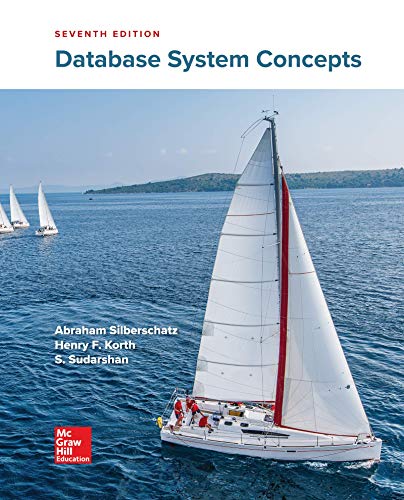
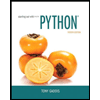
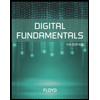
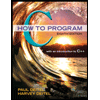
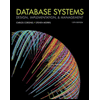
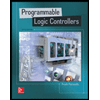