Input file data : Nunavut,35000,1 Alberta,4067000,34 Saskatchewan,1098000,14 Yukon,35000,1 Manitoba,1278000,14 British Columbia,4648000,42 Ontario,13448000,121 Quebec,8164000,78 Prince Edward Island,142000,4 Newfoundland,519000,7 Northwest Territories,41000,1 Nova Scotia,923000,11 New Brunswick,747000,10
Input file data : Nunavut,35000,1 Alberta,4067000,34 Saskatchewan,1098000,14 Yukon,35000,1 Manitoba,1278000,14 British Columbia,4648000,42 Ontario,13448000,121 Quebec,8164000,78 Prince Edward Island,142000,4 Newfoundland,519000,7 Northwest Territories,41000,1 Nova Scotia,923000,11 New Brunswick,747000,10
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Input file data :
Nunavut,35000,1
Alberta,4067000,34
Saskatchewan,1098000,14
Yukon,35000,1
Manitoba,1278000,14
British Columbia,4648000,42
Ontario,13448000,121
Quebec,8164000,78
Prince Edward Island,142000,4
Newfoundland,519000,7
Northwest Territories,41000,1
Nova Scotia,923000,11
New Brunswick,747000,10

Transcribed Image Text:In Canada, the federal government is made up of a number of seats. The seats are divided among the
provinces; for example, Saskatchewan has 14 seats, while Ontario has 121.
For this question, you will write a program using population data from the Canadian census to determine
which provinces are over- or under- reprented in the federal government based on their populations.
Using Numpy Arrays
The main purpose of this question is to practice data manipulation using numpy arrays. Therefore, for full
marks, you must perform all of the significant calculations by using numpy array operations.
You will need all three of: array arithmetic, array relations, and logical array indexing. The only place where
you will use loops in your program is at the very beginning (for reading from file) and at the very end (for
printing the results).
Task Breakdown
The steps below will help you break down this process into manageable pieces.
Before you start anything, make sure you have imported the numpy module. This module is NOT standard,
so if you are working on your own machine, you may need to install it first.
Load the Data into Arrays
Download the file provincial_seats.txt from the course Moodle. The file looks like this:
Nunavut ,35000,1
Alberta , 4067000 ,34
Saskatchewan , 1098000 , 14
Yukon , 35000,1
Each line consists of a province or territory's name, its population (rounded to the nearest thousand) and
its number of seats in the government, all separated by commas.
Write code to open this file and read this data into three separate lists (one for the names, one for the
populations, and one for the seats). Then, convert each list to a numpy array using the .array() method
from numpy. Make sure to maintain the order so that the lists line up by position (i.e. Nunavut is first in the
array of names, and its matching population and seats are the first items in their respective arrays).
Test these arrays before you move on! You will use them to perform all the following array calculations.
Predicted Seats
Using array operations on the loaded data, create an array that contains, for each province, the expected
number of seats that each province WwOULD have if its seats were exactly proportional to its population.
Round these values to the nearest integer (you can use numpy's .around () method).
Hint: As part of this calculation, you'll probably need Canada's total population, and the total number of
seats. Think about how you can get these using the data you already have using numpy's .sum() method.

Transcribed Image Text:Finding Over- and Under-represented provinces
If a province has more actual seats than the number of seats you calculated based on its population above,
we'll call that province over-represented. If it has fewer actual seats than predicted seats, then it is under-
represented.
Use array relational operators and logical indexing to create three arrays for:
• the names of the over-represented provinces
• the expected seats of those provinces
• the actual seats of those provinces
Then do the same thing for the under-represented provinces.
Finally, print your results to the console. You can use loops for this final task.
Sample Run
The output of your program might look something like this:
Based on population, the following provinces are over -repres ented :
Actual : 1
Nuna vut
Expected: o
Saskatchevan
Expected : 11
Actual: 14
Expected : 0
Expected : 12
Yukon
Actual: 1
Manitoba
Actual: 14
Prince Edward Island
Expected: 1
Actual: 4
Newfoundland
Expected : 5
Actual: 7
Northwest Territories
Expected: 0
Actual : 1
Nova Scotia
Expected: 9
Actual: 11
New Brunswick
Expected: 7
Actual: 10
The following provinces are under -represented:
Alberta
Expected: 39
Actual: 34
British Columbia
Expected: 45
Actual: 42
Expected: 129
Expected: 79
Ontario
Actual: 121
Quebec
Actual : 78
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
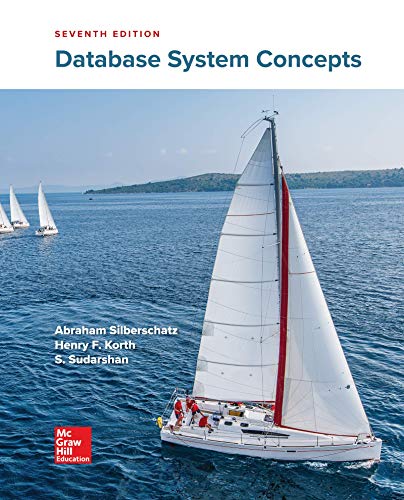
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
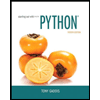
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
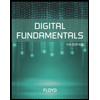
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
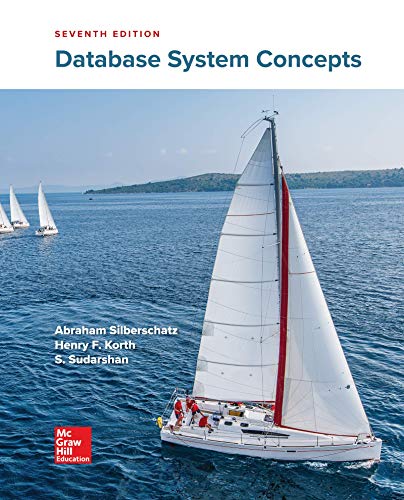
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
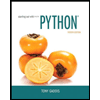
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
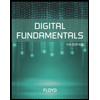
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
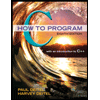
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
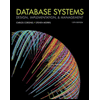
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
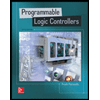
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education