Requirement. 1. Use the C++ library's random number generator, and include the srand statement so that the result is not the same every time you run the program. Program I/O. Input: none. Output: either Heads or Tails. Example. Heads


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

#include <iostream>
#include <ctime>
using namespace std;
//main function
int main()
{
//calling random number generator function
srand(time(0));
int input;
cout << "Enter the number of tosses to perform: ";
cin >> input; //read input
int counter=0; //initialize counter to 0
while(1) //infinite loop
{
if(counter==input) //if limit reached
break; //exit from loop
int flip = rand() % 2; //get value
if (flip == 0) //if value is 0
cout << "Heads" << endl;
else //if value is 1
cout << "Tails" << endl;
counter=counter+1; //add 1 to counter
}
return 0;
}
the program should be based on the code above, and should not use arrays.
![Coin Toss, v.3.0
Purpose. Learn how to use "nested loops" to put "replay" a program that already contains a loop.
Requirements. Rewrite your coinToss2.cpp from exercise 7.4 as coinToss3.cpp. Modify the
program to add another loop that lets the user replay the entire game. If the user enters sentinel value
of 0 (zero) for the number of tosses, break out of the outer replay loop to end the program.
Be sure to explain this to the user in the prompt -- something like: "Enter the number of tosses to
perform [0=exit]:".
Supplemental. Read about "randomizing" in www.rdb3.com/cpp/exercises/Gaming.supplemental.pdf.
Program I/O. Input: a non-negative number from the console keyboard, repeated in a loop until the
sentinel value of 0 is entered. Output: a series of Heads or Tails repeated in a loop until the sentinel
value is entered.
Example. with user input in blue
Enter the number of tosses to perform [0=exit]: 3
Heads
Tails
Heads
Enter the number of tosses to perform [0=exit]: 2
Tails
Tails
Enter the number of tosses to perform [0=exit]: 0](https://content.bartleby.com/qna-images/question/5a4a4196-402b-41d8-8cc6-400e379b188a/2e8d329e-c0bd-4f1f-ae84-5578b733e8cd/isflu5i_thumbnail.png)
The program needs to be modified.
![Coin Toss, v.3.0
Purpose. Learn how to use "nested loops" to put "replay" a program that already contains a loop.
Requirements. Rewrite your coinToss2.cpp from exercise 7.4 as coinToss3.cpp. Modify the
program to add another loop that lets the user replay the entire game. If the user enters sentinel value
of 0 (zero) for the number of tosses, break out of the outer replay loop to end the program.
Be sure to explain this to the user in the prompt -- something like: "Enter the number of tosses to
perform [0=exit]:".
Supplemental. Read about "randomizing" in www.rdb3.com/cpp/exercises/Gaming.supplemental.pdf.
Program I/O. Input: a non-negative number from the console keyboard, repeated in a loop until the
sentinel value of 0 is entered. Output: a series of Heads or Tails repeated in a loop until the sentinel
value is entered.
Example. with user input in blue
Enter the number of tosses to perform [0=exit]: 3
Heads
Tails
Heads
Enter the number of tosses to perform [0=exit]: 2
Tails
Tails
Enter the number of tosses to perform [0=exit]: 0](https://content.bartleby.com/qna-images/question/6f46ae0e-a0ee-4500-808d-7fa0f129e90c/54268fd9-34ea-4648-87d0-199a80538463/8ui8ba3_thumbnail.png)
The program should be according to the
Please refer to the algorithm highlighted.
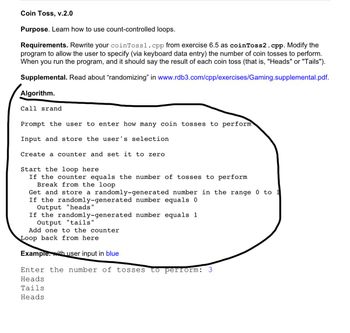
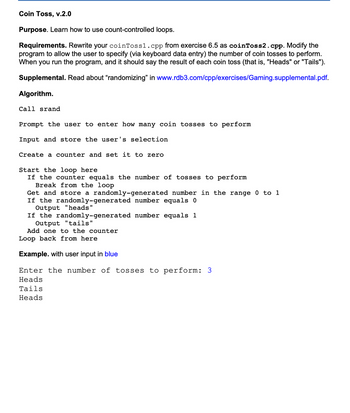
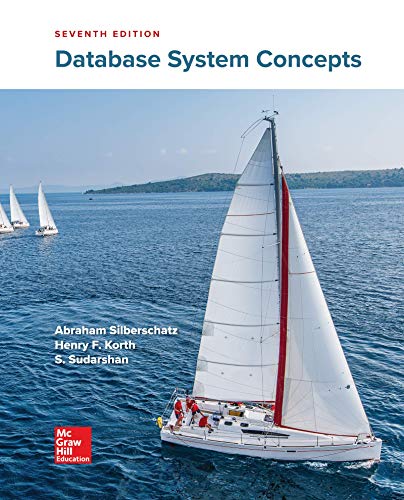
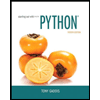
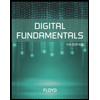
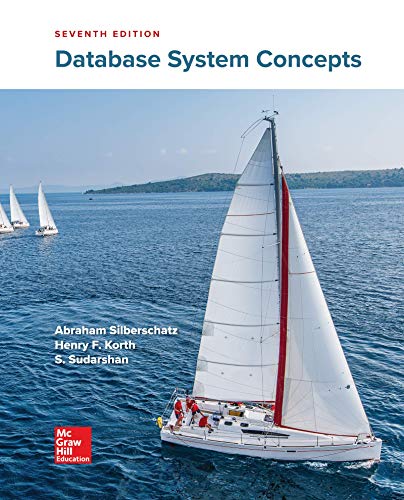
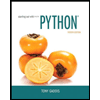
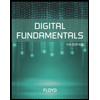
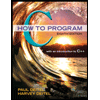
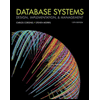
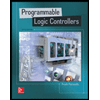