#include class A { private: class B { public: B() { x = 1; } int x; int& getx() { return x; } void print() { printf(“%d”,x); } }; private: B b; public: class C { private: int w; public: C() { w = 5; } B b; int &getw() { return w; } void print() { b.print(); printf(“%d”,w); } }; C c; void print() { b.print(); c.print(); } int& getx() { return b.getx(); } }; Assume each code snippet in each row below runs independently in a main function. For each one, say whether it's legal code or not. If it is, show the output and explain why it happens. If it’s illegal (e.g. syntax error), explain why. Try to do it without running the code, and then check and correct your answers as needed. # Code snippet Errors? (Yes or No) Output or explanation of error. 1 A a; a.print(); 2 A a; a.c.b.x = -1; a.print(); 3 A a; a.c.w = 10; a.c.print(); 4 A a; int& x = a.getx(); x = 21; a.print(); 5 A a; int& w = a.c.getw(); w = 19; a.c.print(); 6 A::B b; b.print(); 7 A a; int& w = a.c.getw(); int& x = a.getx(); w = 19; x = w; w = 20; a.print(); 8 C c; 9 A::C a; a.print();
Consider the following class declaration and variable definitions, then answer the following questions.
#include <stdio.h>
class A {
private:
class B {
public:
B() { x = 1; }
int x;
int& getx() { return x; }
void print() { printf(“%d”,x); }
};
private:
B b;
public:
class C {
private:
int w;
public:
C() { w = 5; }
B b;
int &getw() { return w; }
void print() { b.print(); printf(“%d”,w); }
};
C c;
void print() { b.print(); c.print(); }
int& getx() { return b.getx(); }
};
Assume each code snippet in each row below runs independently in a main function. For each one, say whether it's legal code or not. If it is, show the output and explain why it happens. If it’s illegal (e.g. syntax error), explain why. Try to do it without running the code, and then check and correct your answers as needed.
# |
Code snippet |
Errors? (Yes or No) |
Output or explanation of error. |
1 |
A a; a.print(); |
|
|
2 |
A a; a.c.b.x = -1; a.print(); |
|
|
3 |
A a; a.c.w = 10; a.c.print(); |
|
|
4 |
A a; int& x = a.getx(); x = 21; a.print(); |
|
|
5 |
A a; int& w = a.c.getw(); w = 19; a.c.print(); |
|
|
6 |
A::B b; b.print(); |
|
|
7 |
A a; int& w = a.c.getw(); int& x = a.getx(); w = 19; x = w; w = 20; a.print(); |
|
|
8 |
C c; |
|
|
9 |
A::C a; a.print(); |
|
|

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

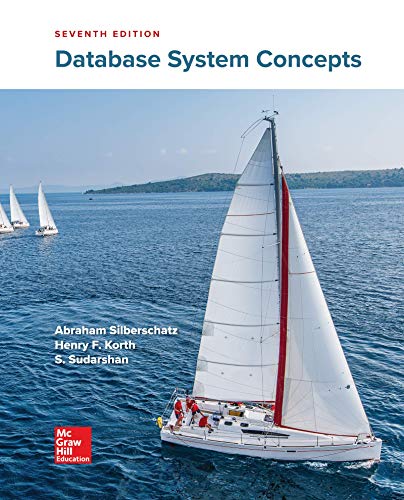
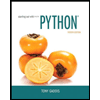
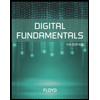
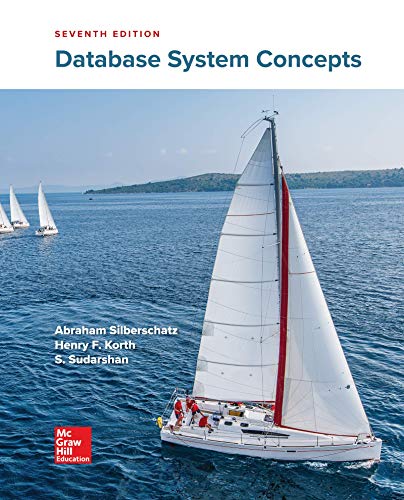
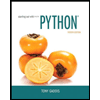
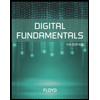
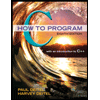
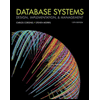
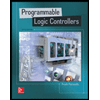