In your index.html, notice the following: In your script.js file, create the following: // Courtesy Comments: Name and Date at bare minimum // Add your code here let quoteStart = 'Don't judge each day by the harvest you reap '; // Don't edit the code below here! const section = document.querySelector('section'); let para1 = document.createElement('p'); para1.textContent = finalQuote; section.appendChild(para1); Don't forget to add your courtesy comments! (Your name, the date, etc.) In the Javascript provided above, you have half of a famous quote inside a variable called quoteStart. To complete the assignment, you must do the following: Look up the other half of the quote Add the second half of the quote inside a variable called quoteEnd. Concatenate the two strings together as variables (do not just re-write the strings!) to make a single string containing the complete quote. The result of these two strings should be assigned to a variable called finalQuote. Run your repl. You'll find that you get an error at this point. Fix the problem with quoteStart, so that the full quote displays correctly, then re-run your repl. Submit your repl by clicking the Submit button replit css p { color: purple; margin: 0.5em 0; } * { box-sizing: border-box;
In your index.html, notice the following:
<section class="preview"></section>
In your script.js file, create the following:
// Courtesy Comments: Name and Date at bare minimum
// Add your code here
let quoteStart = 'Don't judge each day by the harvest you reap ';
// Don't edit the code below here!
const section = document.querySelector('section');
let para1 = document.createElement('p');
para1.textContent = finalQuote;
section.appendChild(para1);
Don't forget to add your courtesy comments! (Your name, the date, etc.)
In the Javascript provided above, you have half of a famous quote inside a variable called quoteStart.
To complete the assignment, you must do the following:
- Look up the other half of the quote
- Add the second half of the quote inside a variable called quoteEnd.
- Concatenate the two strings together as variables (do not just re-write the strings!) to make a single string containing the complete quote. The result of these two strings should be assigned to a variable called finalQuote.
- Run your repl. You'll find that you get an error at this point.
- Fix the problem with quoteStart, so that the full quote displays correctly, then re-run your repl.
- Submit your repl by clicking the Submit button
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>replit</title>
<link href="style.css" rel="stylesheet" type="text/css" />
</head>
<body>
<section class="preview"></section>
<script src="script.js"></script>
</body>
</html>
css
p {
color: purple;
margin: 0.5em 0;
}
* {
box-sizing: border-box;
}

In this question we have to write a HTML CSS JS based code, where we need to complete the JavaScript code snippet to create a famous quote and display it on a web page.
Let's code and hope this helps, if you find any issues on the solution utilize threaded question feature.
Step by step
Solved in 3 steps with 2 images

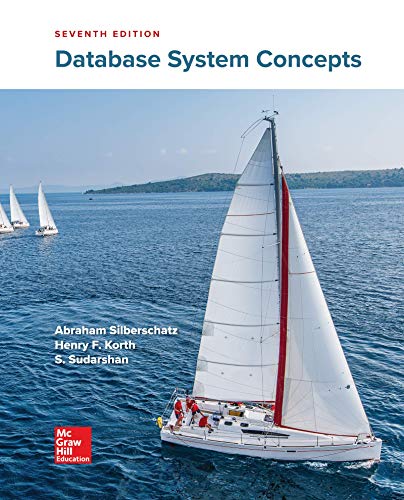
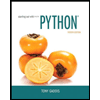
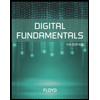
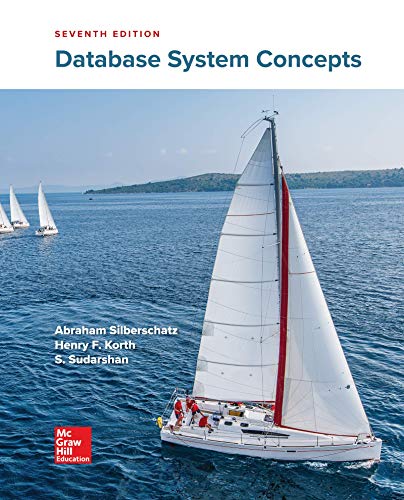
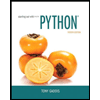
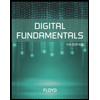
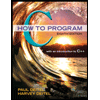
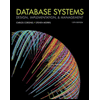
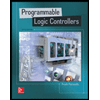