In this python code %recall 5 which is in line 20 keeps giving me a SyntaxError: Invalid Syntax error. How do I fix it? import matplotlib.pyplot as plt import numpy as np import random import seaborn as sns rolls = [random.randrange(1, 7) for i in range(600)] values, frequencies = np.unique(rolls, return_counts=True) title = f'Rolling a Six-Sided Die {len(rolls):,} Times' sns.set_style('whitegrid') axes = sns.barplot(x=values, y=frequencies, palette='bright') print(axes.set_title(title)) print(axes.set(xlabel='Die Value', ylabel='Frequency')) print(axes.set_ylim(top=max(frequencies) * 1.10)) for bar, frequency in zip(axes.patches, frequencies): text_x = bar.get_x() + bar.get_width() / 2.0 text_y = bar.get_height() text = f'{frequency:,}\n{frequency / len(rolls):.3%}' axes.text(text_x, text_y, text, fontsize=11, ha='center', va='bottom') plt.cla() %recall 5 rolls = [random.randrange(1, 7) for i in range(600)] rolls = [random.randrange(1, 7) for i in range(60000)] %recall 6-13 values, frequencies = np.unique(rolls, return_counts=True) title = f'Rolling a Six-Sided Die {len(rolls):,} Times' sns.set_style('whitegrid') axes = sns.barplot(x=values, y=frequencies, palette='bright') axes.set_title(title) axes.set(xlabel='Die Value', ylabel='Frequency') axes.set_ylim(top=max(frequencies) * 1.10) for bar, frequency in zip(axes.patches, frequencies): text_x = bar.get_x() + bar.get_width() / 2.0 text_y = bar.get_height() text = f'{frequency:,}\n{frequency / len(rolls):.3%}' axes.text(text_x, text_y, text, fontsize=11, ha='center', va='bottom')
In this python code %recall 5 which is in line 20 keeps giving me a SyntaxError: Invalid Syntax error. How do I fix it?
import matplotlib.pyplot as plt
import numpy as np
import random
import seaborn as sns
rolls = [random.randrange(1, 7) for i in range(600)]
values, frequencies = np.unique(rolls, return_counts=True)
title = f'Rolling a Six-Sided Die {len(rolls):,} Times'
sns.set_style('whitegrid')
axes = sns.barplot(x=values, y=frequencies, palette='bright')
print(axes.set_title(title))
print(axes.set(xlabel='Die Value', ylabel='Frequency'))
print(axes.set_ylim(top=max(frequencies) * 1.10))
for bar, frequency in zip(axes.patches, frequencies):
text_x = bar.get_x() + bar.get_width() / 2.0
text_y = bar.get_height()
text = f'{frequency:,}\n{frequency / len(rolls):.3%}'
axes.text(text_x, text_y, text,
fontsize=11, ha='center', va='bottom')
plt.cla()
%recall 5
rolls = [random.randrange(1, 7) for i in range(600)]
rolls = [random.randrange(1, 7) for i in range(60000)]
%recall 6-13
values, frequencies = np.unique(rolls, return_counts=True)
title = f'Rolling a Six-Sided Die {len(rolls):,} Times'
sns.set_style('whitegrid')
axes = sns.barplot(x=values, y=frequencies, palette='bright')
axes.set_title(title)
axes.set(xlabel='Die Value', ylabel='Frequency')
axes.set_ylim(top=max(frequencies) * 1.10)
for bar, frequency in zip(axes.patches, frequencies):
text_x = bar.get_x() + bar.get_width() / 2.0
text_y = bar.get_height()
text = f'{frequency:,}\n{frequency / len(rolls):.3%}'
axes.text(text_x, text_y, text,
fontsize=11, ha='center', va='bottom')

Your code is fine. But one thing that needs to keep in mind while using recall is - if you recall any line directly the code will throw an error - no history of about 5 or any line number. The reason during the first compilation there is no code or history of line number 5.
If you run the code again and observe the output you can the right output. For reference please check the below images which I got after running the code.
1st image is after running the program first time.
2nd image is after running the 2nd time when the error is resolved.
Step by step
Solved in 2 steps with 2 images

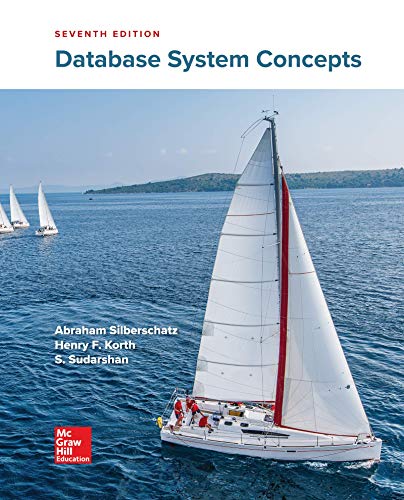
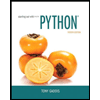
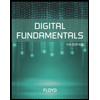
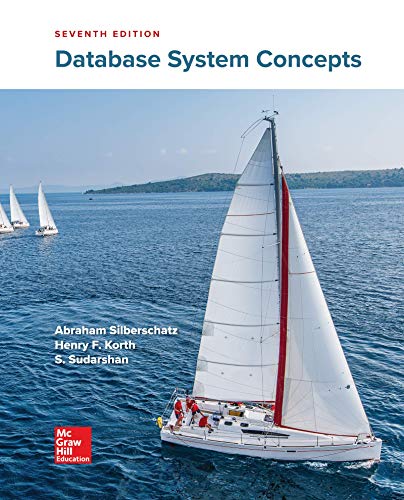
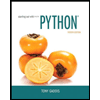
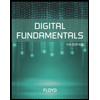
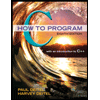
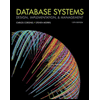
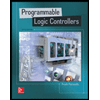