In this exercise, you create an application that keeps track of cookie sales. Create a Windows Forms application. Use the following names for the project and solution, respectively: Cookies Project and Cookies Solution. Save the application in the VB2017\Chap11 folder. Figure 11-59 shows the Sales table contained in the VB2017\Chap11\Databases\Cookies.mdf file. The table contains the numbers of boxes of cookies sold in each of three weeks. The Week field is an auto-numbered field. Open the Data Sources window and click Add New Data Source to start the Data Source Configuration Wizard. Connect the Cookies.mdf file to the application. Include the entire Sales table in the dataset. Set the Cookies.mdf file’s Copy to Output Directory property to “Copy if newer”. Display the dataset information in a DataGridView control and then make the necessary modifications to the control. Enter an appropriate Try...Catch statement in the SalesBindingNavigatorSaveItem_Click procedure. Save the solution and then start the application. Change the Chocolate Chip cookie sales in Week 1 to 205. Then, enter the following record for Week 4: 150, 112, and 76. Save the changes. Stop the application and then start it again to verify that the changes you made were saved. Option Explicit On Option Strict On Option Infer Off Imports System.Data.sqlclient Public Class form1 Dim con As sqlconnection Dim da As sqldataadapter Dim cmd As sqlcommand Dim ds As dataset Private Sub form1_load(sender As Object, e As EventArgs) Handles MyBase.load salesbindingnavigation() End Sub Sub salesbindingnavigation() con = New sqlconnection("data source=(localdb)/v11.0;attachdbfilename=" + application.startuppath + "/cockies.mdf;integrated security = true; connect timeout = 30") con.open() da = New sqldataadapter("select * from sales", con) ds = New dataset da.fill(ds, "sales") dgvsales.datasource = ds.tables(0) End Sub Private Sub dgvsales_rowenter(sender As Object, e As datagridviewcelleventargs) Handles dgvsales.rowenter End Sub Private Sub dgvsales_click(sender As Object, e As EventArgs) Handles dgvsales.click Try Dim n1 As String = dgvsales.rows(dgvsales.CurrnetRow.index).cells(1).value Dim n2 As String = dgvsales.rows(dgvsales.CurrnetRow.index).cells(2).value Dim n3 As String = dgvsales.rows(dgvsales.CurrnetRow.index).cells(3).value cmd = New SqlCommand("insert into [sales] values(NEXT VALUE FOR Week_seq," + n1 + "," + n2 + "," + n3 + ")", con) cmd.ExecuteNonQuery() salesbindingnavigation() MsgBox("inserted") Catch ex As Exception End Try End Sub End Class Public Class frmMain Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click Me.Close() End Sub End Class
In this exercise, you create an application that keeps track of cookie sales. Create a Windows Forms application. Use the following names for the project and solution, respectively: Cookies Project and Cookies Solution. Save the application in the VB2017\Chap11 folder.
- Figure 11-59 shows the Sales table contained in the VB2017\Chap11\
Databases \Cookies.mdf file. The table contains the numbers of boxes of cookies sold in each of three weeks. The Week field is an auto-numbered field. Open the Data Sources window and click Add New Data Source to start the Data Source Configuration Wizard. Connect the Cookies.mdf file to the application. Include the entire Sales table in the dataset. - Set the Cookies.mdf file’s Copy to Output Directory property to “Copy if newer”.
- Display the dataset information in a DataGridView control and then make the necessary modifications to the control.
- Enter an appropriate Try...Catch statement in the SalesBindingNavigatorSaveItem_Click procedure.
- Save the solution and then start the application. Change the Chocolate Chip cookie sales in Week 1 to 205. Then, enter the following record for Week 4: 150, 112, and 76. Save the changes.
- Stop the application and then start it again to verify that the changes you made were saved.
Option Explicit On
Option Strict On
Option Infer Off
Imports System.Data.sqlclient
Public Class form1
Dim con As sqlconnection
Dim da As sqldataadapter
Dim cmd As sqlcommand
Dim ds As dataset
Private Sub form1_load(sender As Object, e As EventArgs) Handles MyBase.load
salesbindingnavigation()
End Sub
Sub salesbindingnavigation()
con = New sqlconnection("data source=(localdb)/v11.0;attachdbfilename=" +
application.startuppath + "/cockies.mdf;integrated security = true; connect timeout = 30")
con.open()
da = New sqldataadapter("select * from sales", con)
ds = New dataset
da.fill(ds, "sales")
dgvsales.datasource = ds.tables(0)
End Sub
Private Sub dgvsales_rowenter(sender As Object, e As datagridviewcelleventargs) Handles
dgvsales.rowenter
End Sub
Private Sub dgvsales_click(sender As Object, e As EventArgs) Handles dgvsales.click
Try
Dim n1 As String = dgvsales.rows(dgvsales.CurrnetRow.index).cells(1).value
Dim n2 As String = dgvsales.rows(dgvsales.CurrnetRow.index).cells(2).value
Dim n3 As String = dgvsales.rows(dgvsales.CurrnetRow.index).cells(3).value
cmd = New SqlCommand("insert into [sales] values(NEXT VALUE FOR Week_seq," + n1 + "," + n2 + "," + n3 + ")", con)
cmd.ExecuteNonQuery()
salesbindingnavigation()
MsgBox("inserted")
Catch ex As Exception
End Try
End Sub
End Class
Public Class frmMain
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Me.Close()
End Sub
End Class


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

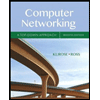
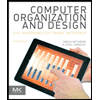
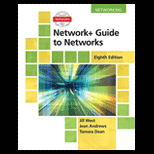
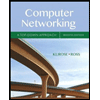
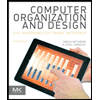
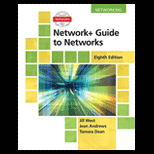
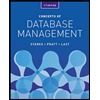
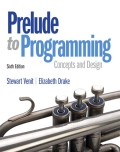
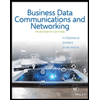