In this assignment, you will design a program in which you will ask the user to enter a number and use a while loop to see if the number entered by the user is a palindrome number or not. A palindrome number is a number which remains the same, even when the digits are reversed. For example: 121 is a palindrome number. 277 is not a palindrome number. Start Start of main function § Prompt the user a number ‘n’ § Assign ‘n’ to another temporary variable ‘temp’ § Initialize a variable ‘rev’ to 0 § While ‘n’ is greater than 0 divide ‘n’ by by 10 and assign the remainder to a variable ‘dig’ multiply ‘rev’ by 10 and add to ‘dig’ and assign result to ‘rev’ divide ‘n’ by 10 to get the quotient and assign to ‘n’ § If ‘temp’ is equal to variable ‘rev’ Print ‘The number is a palindrome’ § Else Print ‘The number is not a palindrome’ End of main function End
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
In this assignment, you will design a
a while loop to see if the number entered by the user is a palindrome number or not. A palindrome
number is a number which remains the same, even when the digits are reversed. For example: 121 is a
palindrome number. 277 is not a palindrome number.
Start
Start of main function
§ Prompt the user a number ‘n’
§ Assign ‘n’ to another temporary variable ‘temp’
§ Initialize a variable ‘rev’ to 0
§ While ‘n’ is greater than 0
divide ‘n’ by by 10 and assign the remainder to a variable ‘dig’
multiply ‘rev’ by 10 and add to ‘dig’ and assign result to ‘rev’
divide ‘n’ by 10 to get the quotient and assign to ‘n’
§ If ‘temp’ is equal to variable ‘rev’
Print ‘The number is a palindrome’
§ Else
Print ‘The number is not a palindrome’
End of main function
End

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

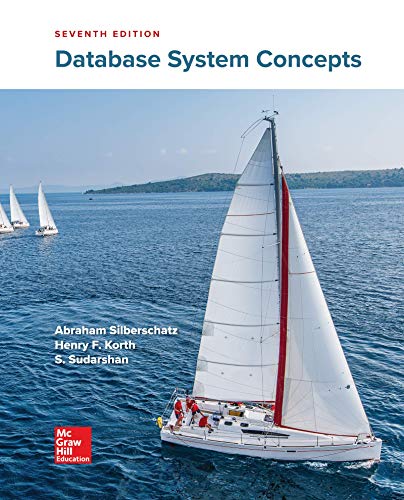
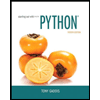
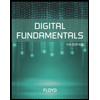
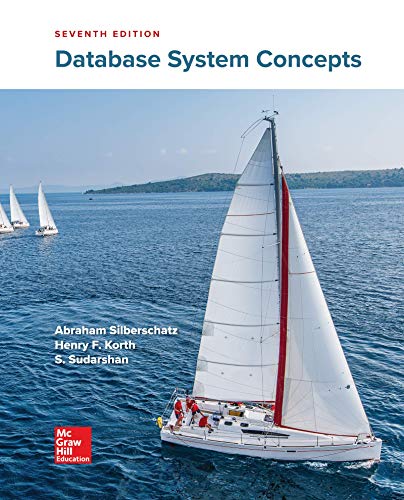
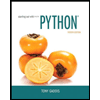
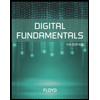
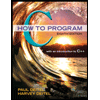
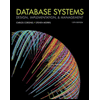
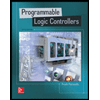