In this Assignment you need to design, analyze and implement an algorithm to print the elements that appear in both arrays in sorted order. Write a C/Java program to read two arrays of N int values, and print all elements that appear in both arrays in a sorted order. You need to calculate the execution time for your algorithm when the input data is randomly created and when the input data is sorted in ascending order. Your program should implement the following operations: Randomly initialize n int values starting from 0 and store them in array inputData(int X[], int n) Call this function to initialize the values of 2 arrays. Initialize n int values starting from 0 in an ascending order and store these values in array X. inputData(int X[], int n, int increment) Call this function two times to initialize the values of 2 arrays sorted in ascending order with different increment. For example, if the increment value is equal to 5 the values of an array will be: 0, 5, 10, 15, 20,25.... PrintElementsinBothArraysAlgforRandomInput (int A[], int B[]) Design an algorithm to print elements in both arrays where both arrays are in a random order. PrintElementsinBothArraysAlgforSortedInput (int A[], int B[]) Design a different algorithm that prints the elements that both arrays where both arrays are in sorted order. Requirements: The program should print all common elements in both arrays. You need to design: PrintElementsinBothArraysAlgforRandomInput takes as an input two arrays and print elements in both arrays. PrintElementsinBothArraysAlgforSortedInput takes as input two sorted arrays and print elements in both arrays. Your program should perform an experimental analysis of their running times by doing the following: For each algorithm, choose at least 3 appropriate large values for n, where n is the input array size, and determine how long it takes to run in nanoseconds. For example, value of n (10000,20000, 40,0000,…..100000, etc). Notes: Try to choose large values for n. to avoid an erratic timing (e.g. 0s or there is no clear increase in time with respect to input size). You are required to use the same values of n for both arrays. Your report should include a write up for the following: Theoretical analysis of the two algorithms in terms of Big-O. Show and explain the experimental analysis of their running times by plotting the running times obtained for algorithm 1 and algorithm 2 as a function of n as scatter plots on a linear scale. Handwritten plot will not be accepted. Justify your answers and compare between theoretical and experimental results.
In this Assignment you need to design, analyze and implement an
You need to calculate the execution time for your algorithm when the input data is randomly created and when the input data is sorted in ascending order.
Your program should implement the following operations:
- Randomly initialize n int values starting from 0 and store them in array
inputData(int X[], int n)
Call this function to initialize the values of 2 arrays.
- Initialize n int values starting from 0 in an ascending order and store these values in array X.
inputData(int X[], int n, int increment)
Call this function two times to initialize the values of 2 arrays sorted in ascending order with different increment. For example, if the increment value is equal to 5 the values of an array will be: 0, 5, 10, 15, 20,25....
- PrintElementsinBothArraysAlgforRandomInput (int A[], int B[])
Design an algorithm to print elements in both arrays where both arrays are in a random order.
- PrintElementsinBothArraysAlgforSortedInput (int A[], int B[])
Design a different algorithm that prints the elements that both arrays where both arrays are in sorted order.
Requirements:
- The program should print all common elements in both arrays. You need to design:
- PrintElementsinBothArraysAlgforRandomInput takes as an input two arrays and print elements in both arrays.
- PrintElementsinBothArraysAlgforSortedInput takes as input two sorted arrays and print elements in both arrays.
- Your program should perform an experimental analysis of their running times by doing the following:
For each algorithm, choose at least 3 appropriate large values for n, where n is the input array size, and determine how long it takes to run in nanoseconds. For example, value of n (10000,20000, 40,0000,…..100000, etc).
Notes:
- Try to choose large values for n. to avoid an erratic timing (e.g. 0s or there is no clear increase in time with respect to input size).
- You are required to use the same values of n for both arrays.
- Your report should include a write up for the following:
- Theoretical analysis of the two algorithms in terms of Big-O.
- Show and explain the experimental analysis of their running times by plotting the running times obtained for algorithm 1 and algorithm 2 as a function of n as scatter plots on a linear scale. Handwritten plot will not be accepted.
- Justify your answers and compare between theoretical and experimental results.

Step by step
Solved in 2 steps with 1 images

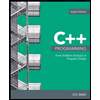
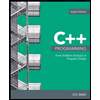