In this activity, you will complete the following template functions: • Using char_to_ascii(), write a program that converts each character in the given string word into its integer counterpart (ASCII decimal value) and returns them in the list ascii_values. Using ascii_to_binary(), write a program that will convert the given list, ascii_values, into strings containing their binary values and return the converted values in the list binary_values. e.g. the list [4, 3, 1] will be returned as ['100', '11', '1]] Hint: you can solve each of these using Python's in-built functions.
How to apply this python code?
def char_to_ascii(data_list):
ascii_values= []
for value in data_list:
converted_value = ''
### Do not modify anything above this line ###
# insert your code here #
print (char, ord(char))
### Do not modify anything below this line ###
ascii_values.append(converted_value)
return ascii_values
def ascii_to_binary(data_list):
binary_values= []
for value in data_list:
converted_value = ''
### Do not modify anything above this line ###
# insert your code here #
print(ord, char(ord))
### Do not modify anything below this line ###
binary_values.append(converted_value)
return binary_values
if __name__ == "__main__":
word = input()
ascii_data = char_to_ascii(word)
binary_data = ascii_to_binary(ascii_data)
for character, ascii, binary in zip(word, ascii_data, binary_data):
print('{}: {} - {}'.format(character, ascii, binary))
![In this activity, you will complete the following template functions:
• Using char_to_ascii(), write a program that converts each character in the given string word into its integer
counterpart (ASCII decimal value) and returns them in the list ascii_values.
• Using ascii to_binary(), write a program that will convert the given list, ascii_values, into strings containing
their binary values and return the converted values in the list binary_values. e.g. the list [4, 3, 1] will be
returned as ['100', '11', '1]]
Hint: you can solve each of these using Python's in-built functions.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5e4b46f5-fd0b-4b69-8fa1-4775c5e817f6%2Fcdd6bdc6-9bac-42b0-94e5-9701f080b884%2F6nqel4r_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

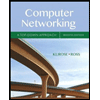
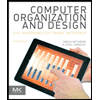
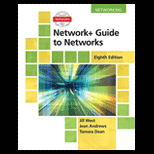
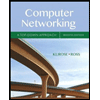
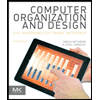
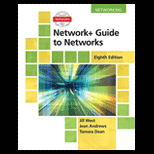
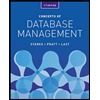
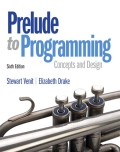
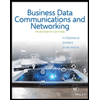