In Python I keep getting a whitespace error Write a program that replaces words in a sentence. The input begins with word replacement pairs (original and replacement). The next line of input is the sentence where any word on the original list is replaced. Ex: If the input is: automobile car manufacturer maker children kids The automobile manufacturer recommends car seats for children if the automobile doesn't already have one. the output is: The car maker recommends car seats for kids if the car doesn't already have one. You can assume the original words are unique.
In Python
I keep getting a whitespace error
Write a program that replaces words in a sentence. The input begins with word replacement pairs (original and replacement). The next line of input is the sentence where any word on the original list is replaced.
Ex: If the input is:
automobile car manufacturer maker children kids The automobile manufacturer recommends car seats for children if the automobile doesn't already have one.
the output is:
The car maker recommends car seats for kids if the car doesn't already have one.
You can assume the original words are unique.
My Code:
#Define main() function
def main():
#Read data from the user
replace_pairs = input()
#Split replacement pairs into list
replace_pairs = replace_pairs.split()
#Create two lists for original and replacement words
originalWord = []
replacementWord = []
#Iterate through replacement pairs
for i in range(len(replace_pairs)):
#If it is even place
if i % 2 == 0:
#Append current item to original
originalWord.append(replace_pairs[i])
#Otherwise
else:
#append current item to replacement
replacementWord.append(replace_pairs[i])
#Read input sentence
inputSentence = input()
#Split sentence into list
inputSentence = inputSentence.split()
#Create a loop
for i in range(len(inputSentence)):
#Iterate through original list
for j in range(len(originalWord)):
#if current item in sentence matches to any item in original list
if(inputSentence[i] == originalWord[j]):
'''Replace current item in sentence by an item in
replacement which corresponds to item in original'''
inputSentence[i] = replacementWord[j]
#Print output
for i in range(len(inputSentence)):
print(inputSentence[i], end = " ")
print("\n")
#Call main() function
if __name__=="__main__":
main()


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

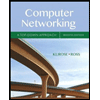
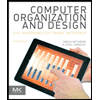
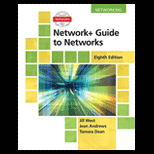
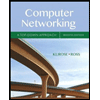
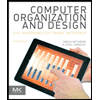
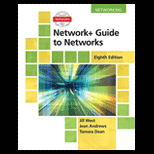
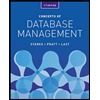
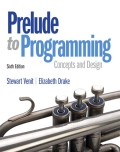
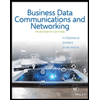