In python Code so far: # TODO: Declare global variables here. recursions = 0 comparisons = 0 def binary_search(nums, target, lower, upper): # Type your code here. target = (lower + upper) //2 global if target == nums[index]: return index if (lower == upper): comparisons +=1 if target == nums[lower]: return lower else: return -1 if (nums[index] < target): recursions += 1 return binary_search(nums,target, index, upper) if nums[index] > target: recursions += 1 return binary_search(nums, target, 0, lower) if __name__ == '__main__': # Input a list of nums from the first line of input nums = [int(n) for n in input().split()] # Input a target value target = int(input()) # Start off with default values: full range of list indices index = binary_search(nums, target, 0, len(nums) - 1) # Output the index where target was found in nums, and the # number of recursions and comparisons performed print(f'index: {index}, recursions: {recursions}, comparisons: {comparisons}')
In python
Code so far:
# TODO: Declare global variables here.
recursions = 0
comparisons = 0
def binary_search(nums, target, lower, upper):
# Type your code here.
target = (lower + upper) //2
global
if target == nums[index]:
return index
if (lower == upper):
comparisons +=1
if target == nums[lower]:
return lower
else:
return -1
if (nums[index] < target):
recursions += 1
return binary_search(nums,target, index, upper)
if nums[index] > target:
recursions += 1
return binary_search(nums, target, 0, lower)
if __name__ == '__main__':
# Input a list of nums from the first line of input
nums = [int(n) for n in input().split()]
# Input a target value
target = int(input())
# Start off with default values: full range of list indices
index = binary_search(nums, target, 0, len(nums) - 1)
# Output the index where target was found in nums, and the
# number of recursions and comparisons performed
print(f'index: {index}, recursions: {recursions}, comparisons: {comparisons}')

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

I'm getting this error for the above code
![1: Compare output
Output differs. See highlights below.
Input
Your output
Expected output
2: Compare output
Input
Your output
Output differs. See highlights below.
Expected output
3: Compare output
Input
Your output
Expected output
4: Compare output
1 2 3 4 5 6 7 8 9
2
index: 1, recursions: 1, comparisons: 1
Output differs. See highlights below.
Input
index: 1, recursions: 2, comparisons:
Your output
11 22 33 44 55 66 77 88 99
11
index: 0, recursions: 2, comparisons: 1
index: 0, recursions: 3, comparisons: 5
10 15 20 25 30 35 40 45
50
index: -1, recursions: 4, comparisons:
Output differs. See highlights below.
index: -1, recursions: 4, comparisons: 7
10 20 20 20 20 20 25 30 35 40 45 50 60
20
index: 2, recursions: 1, comparisons:
Expected output index: 2, recursions: 2, comparisons:
5: Unit test A
Test binary_search() with [11, 22, 33, 44, 55, 66, 77, 88, 99] and target = 99. Should return 8.
Test feedback binary_search() correctly returned 8
0/2
0/2
0/2
0/2
2/2](https://content.bartleby.com/qna-images/question/45d19586-ebc3-4f1a-8702-47e93c6193b1/f32efbbd-169b-4aed-bb1c-d9fa5b959b58/mjaobcp_thumbnail.png)
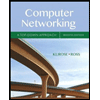
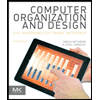
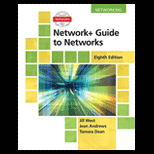
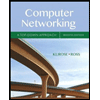
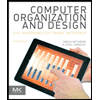
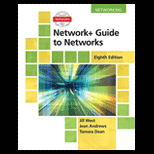
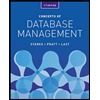
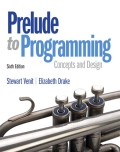
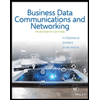