In Java Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: Total rainfall for the year, The average monthly rainfall The month with the most rain The month with the least rain The program should not accept negative numbers for monthly rainfall figures. You may use the following main function to test the methods: public static void main(String[] args) { // Array with this year's rainfall data double[] thisYear = {1.6, 2.1, 1.7, 3.5, 2.6, 3.7, 3.9, 2.6, 2.9, 4.3, 2.4, 3.7 }; int high; // To hold the month with the highest amount int low; // To hold the month with the lowest amount // Display the total rainfall. System.out.println("The total rainfall for this year is " + getTotalRainFall(thisYear)); // Display the average rainfall. System.out.println("The average rainfall for this year is " + getAverageRainFall(thisYear)); // Get and display the month with the highest rainfall. high = getHighestMonth(thisYear); System.out.println("The month with the highest amount of rain " + "is " + (high+1) + " with " + this year[high] + " inches."); // Get and display the month with the lowest rainfall. } // end main // your code… }
In Java
Write a RainFall class that stores the total rainfall for each of 12 months into an
array of doubles. The program should have methods that return the following:
Total rainfall for the year,
The average monthly rainfall
The month with the most rain
The month with the least rain
The program should not accept negative numbers for monthly rainfall figures.
You may use the following main function to test the methods:
public static void main(String[] args)
{
// Array with this year's rainfall data
double[] thisYear = {1.6, 2.1, 1.7, 3.5, 2.6, 3.7,
3.9, 2.6, 2.9, 4.3, 2.4, 3.7 };
int high; // To hold the month with the highest amount
int low; // To hold the month with the lowest amount
// Display the total rainfall.
System.out.println("The total rainfall for this year is " +
getTotalRainFall(thisYear));
// Display the average rainfall.
System.out.println("The average rainfall for this year is " +
getAverageRainFall(thisYear));
// Get and display the month with the highest rainfall.
high = getHighestMonth(thisYear);
System.out.println("The month with the highest amount of rain " +
"is " + (high+1) + " with " + this year[high] +
" inches.");
// Get and display the month with the lowest rainfall.
} // end main
// your code…
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

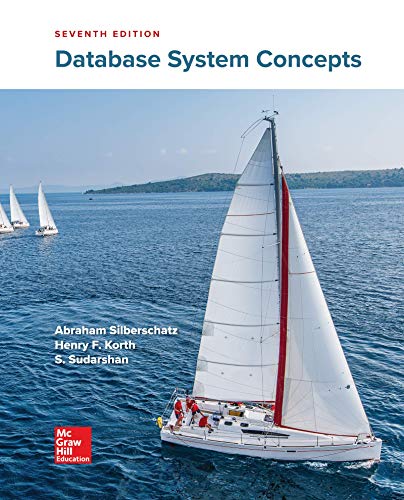
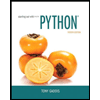
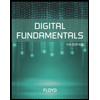
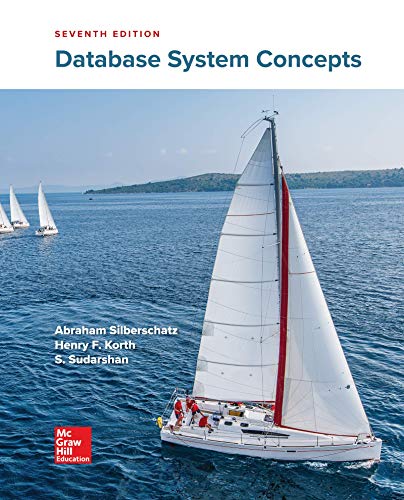
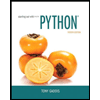
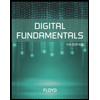
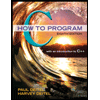
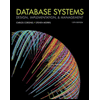
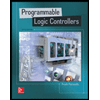