In Java, what are the best practices for implementing the Comparable interface to ensure consistent and predictable sorting behavior across different scenarios?
In Java, what are the best practices for implementing the Comparable interface to ensure consistent and predictable sorting behavior across different scenarios?

You must utilise Java's Comparable interface to establish a natural ordering for the items in your class. You should abide by the following best practices and suggestions to provide predictable and consistent sorting behaviour in a variety of situations:
Train yourself to use the Comparable interface:
The compare To method must be replaced by the Comparable interface in your class. Depending on how the current object compares to the item being compared to, this function should either return a negative integer, zero, or a positive integer.
The following is a basic structure:
1public class YourClass implements Comparable<YourClass> {
2 // ...
3
4 @Override
5 public int compareTo(YourClass other) {
6 // Compare this object with 'other' and return:
7 // -1 if this < other
8 // 0 if this == other
9 // 1 if this > other
10 }
11}
12
Step by step
Solved in 3 steps

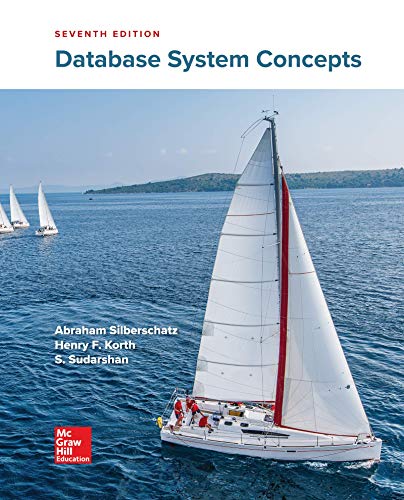
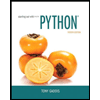
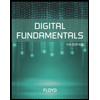
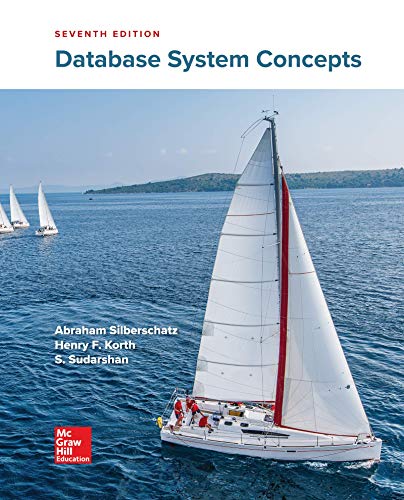
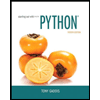
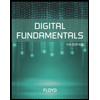
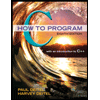
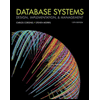
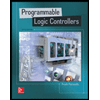