In Java code: Write course management program that allows professors to create course entries to the course library and student to manage course registration. Your program will present a menu with the following options: 1. Professor 1.1 creates a course 1.2 show all courses 2. Student 2.1 views a course's details 2.2 enrolls in a course 2.3 drops a course 2.4 shows all enrolled courses 3. Exit Follow these guidelines to define these classes 1. Professor Class Data fields: id, name, courses taught by the professor Constructors: several no-argument, multi-argument constructors Accessor methods that return the values of data fields: return name, courses Mutator methods that set the data fields, e.g, add/remove a course showCourses: list all the courses taught or created by the professor 2. Student Class Data fields: id, name, gpa, courses enrolled, etc. Constructors: several no-argument, multi-argument constructors Accessor methods that return the values of data fields: return name, courses Mutator methods that set the data fields, e.g, enroll/drop a course showRegistration method: return detailed information about the course the student is enrolled: course id, course name, professor's name, etc. toString(): String representation of the student profile 3. Course Class Data fields: id, course name, credit hours, professor's name, max class size, etc. Constructors: several no-argument, multi-argument constructors Accessor methods that return the values of data fields: return course name, credit hours, max size, professor name, etc. Mutator methods that set the data fields, e.g, change class size, name, etc. showRegistration(): all students enrolled in this class toString(): String representation of the course 4. CourseLibrary Class Data fields: data structure to hold course objects (array, List, Map, etc.) Constructors: several no-argument, multi-argument constructors Accessor methods that return the values of data fields: return Course objects Mutator methods that set the data fields: add/remove courses by name, id, etc. find method: search for courses by id, name, professor's name showAll(): display all courses
In Java code:
Write course management program that allows professors to create course entries to the course library and student to manage course registration.
Your program will present a menu with the following options:
1. Professor
1.1 creates a course
1.2 show all courses
2. Student
2.1 views a course's details
2.2 enrolls in a course
2.3 drops a course
2.4 shows all enrolled courses
3. Exit
Follow these guidelines to define these classes
1. Professor Class
- Data fields: id, name, courses taught by the professor
- Constructors: several no-argument, multi-argument constructors
- Accessor methods that return the values of data fields: return name, courses
- Mutator methods that set the data fields, e.g, add/remove a course
- showCourses: list all the courses taught or created by the professor
2. Student Class
- Data fields: id, name, gpa, courses enrolled, etc.
- Constructors: several no-argument, multi-argument constructors
- Accessor methods that return the values of data fields: return name, courses
- Mutator methods that set the data fields, e.g, enroll/drop a course
- showRegistration method: return detailed information about the course the student is enrolled: course id, course name, professor's name, etc.
- toString(): String representation of the student profile
3. Course Class
- Data fields: id, course name, credit hours, professor's name, max class size, etc.
- Constructors: several no-argument, multi-argument constructors
- Accessor methods that return the values of data fields: return course name, credit hours, max size, professor name, etc.
- Mutator methods that set the data fields, e.g, change class size, name, etc.
- showRegistration(): all students enrolled in this class
- toString(): String representation of the course
4. CourseLibrary Class
- Data fields: data structure to hold course objects (array, List, Map, etc.)
- Constructors: several no-argument, multi-argument constructors
- Accessor methods that return the values of data fields: return Course objects
- Mutator methods that set the data fields: add/remove courses by name, id, etc.
- find method: search for courses by id, name, professor's name
- showAll(): display all courses
5. EntryValidator Class
A utility class that helps validate various user input such as name, class name.
6. CourseManager Class
Define a driver class as the main user interface:
- Data field: data structure holding course collection (array, list, map, ...)
- displays option menu
- takes user input
- parse user input
- displays results
7. Any other class deems necessary
These are just the guideline. Your classes need not be exactly as specified. Create additional classes/methods when needed.
Draw a UML class diagram to show your design. To learn about the basics of UML class diagram, find a tutorial on line.
- Java source code (.java). Name your file appropriately. Do not compress the files.
- A UML class diagram showing class definition and relationships
- Test your program with 5+ different sets of input data. Make sure you capture the interactions,

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

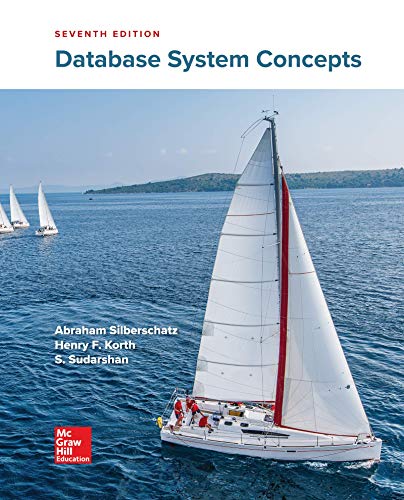
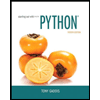
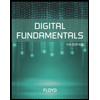
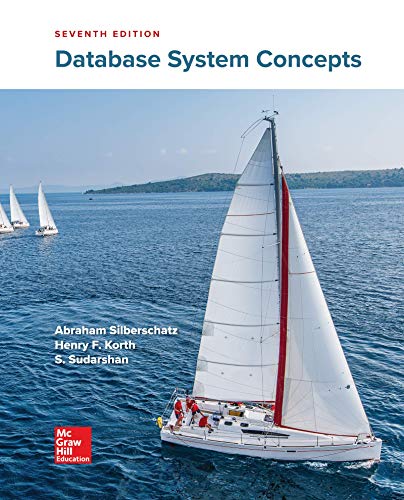
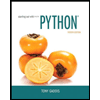
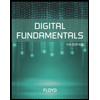
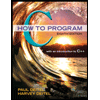
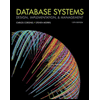
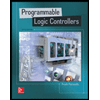