In C++ Stuck and could use help with coding a loop to find the array location of each source node inside the file input loop in main(). Use get_value() to compare a node’s value to source_name and If the node does not exist, add it to the array using the set_value() function and incrementing n. Save index. Comment out the output lines in the file input loop in main(). Sample Run Enter file name: Data.txt Sunday Monday Tuesday Wednesday Thursday Friday Note that Saturday is not shown Node.h // Node Declarations #define ERR -1 #define NODE_MAX 20 #define EDGE_MAX 4 // Node class class node { public: node(); // Constructor void set_value(string); // Set string value string get_value(); // Return string value void connect(node *); // Connect this node to another void put(ostream &); // Output node and neighbors private: string value; // Node value node *edge[EDGE_MAX]; // Edges array }; Node.cpp #include #include using namespace std; #include "Node.h" /****************************** * Null constructor ******************************/ node::node() { int i; value = ""; for(i=0;i edge[i] = NULL; } /****************************** * set_value() ******************************/ void node::set_value(string arg) { value = arg; } /****************************** * get_value() ******************************/ string node::get_value() { return value; } Main.cpp #include #include #include #include using namespace std; #include "Node.h" /****************************** * main() ******************************/ void main() { int i,n; int source_index,target_index,distance; string fname,source_name,target_name; fstream in; node map[NODE_MAX]; // Initialize n = 0; cout << left; // Get file name cout << "Enter file name: "; cin >> fname; // Open file in.open(fname,ios::in); // Loop through file while(!in.eof()) { in >> source_name >> target_name >> distance; // Add to array if(in.good()) { cout << setw(12) << source_name; cout << setw(12) << target_name; cout << setw(4) << distance; cout << endl; }; }; // Close file in.close(); // Display array for(i=0;i cout << map[i].get_value() << endl; } Data.txt Sunday Monday 10 Sunday Tuesday 20 Monday Thursday 30 Tuesday Friday 30 Wednesday Monday 20 Wednesday Tuesday 10 Thursday Sunday 50 Friday Sunday 60 Thursday Wednesday 10 Friday Wednesday 20 Thursday Saturday 20 Friday Saturday 10
In C++
Stuck and could use help with coding a loop to find the array location of each source node inside the file input loop in main(). Use get_value() to compare a node’s value to source_name and If the node does not exist, add it to the array using the set_value() function and incrementing n. Save index.
Comment out the output lines in the file input loop in main().
Sample Run
Enter file name: Data.txt
Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Note that Saturday is not shown
Node.h
// Node Declarations
#define ERR -1
#define NODE_MAX 20
#define EDGE_MAX 4
// Node class
class node
{ public:
node(); // Constructor
void set_value(string); // Set string value
string get_value(); // Return string value
void connect(node *); // Connect this node to another
void put(ostream &); // Output node and neighbors
private:
string value; // Node value
node *edge[EDGE_MAX]; // Edges array
};
Node.cpp
#include
#include
using namespace std;
#include "Node.h"
/******************************
* Null constructor
******************************/
node::node()
{ int i;
value = "";
for(i=0;i
edge[i] = NULL;
}
/******************************
* set_value()
******************************/
void node::set_value(string arg)
{ value = arg;
}
/******************************
* get_value()
******************************/
string node::get_value()
{ return value;
}
Main.cpp
#include
#include
#include
#include
using namespace std;
#include "Node.h"
/******************************
* main()
******************************/
void main()
{ int i,n;
int source_index,target_index,distance;
string fname,source_name,target_name;
fstream in;
node map[NODE_MAX];
// Initialize
n = 0;
cout << left;
// Get file name
cout << "Enter file name: ";
cin >> fname;
// Open file
in.open(fname,ios::in);
// Loop through file
while(!in.eof())
{ in >> source_name >> target_name >> distance;
// Add to array
if(in.good())
{ cout << setw(12) << source_name;
cout << setw(12) << target_name;
cout << setw(4) << distance;
cout << endl;
};
};
// Close file
in.close();
// Display array
for(i=0;i
cout << map[i].get_value() << endl;
}
Data.txt
Sunday Monday 10
Sunday Tuesday 20
Monday Thursday 30
Tuesday Friday 30
Wednesday Monday 20
Wednesday Tuesday 10
Thursday Sunday 50
Friday Sunday 60
Thursday Wednesday 10
Friday Wednesday 20
Thursday Saturday 20
Friday Saturday 10

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

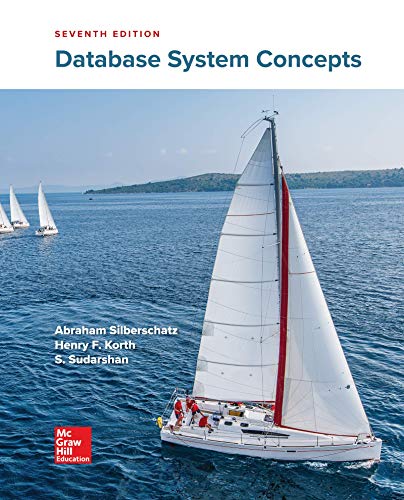
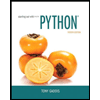
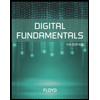
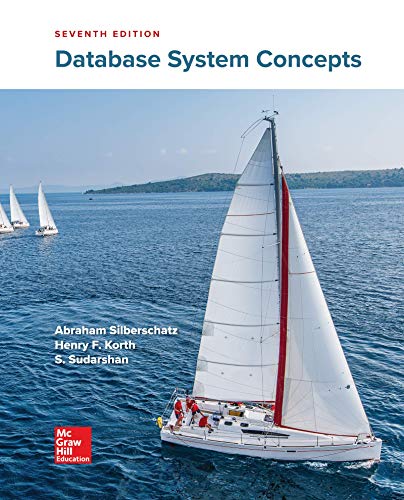
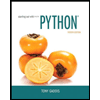
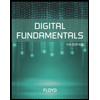
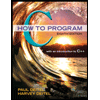
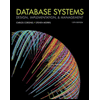
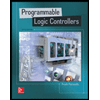