in c++ how would i make a function that takes in an array int nums[] and in size that will sort the array by the last digit of each element so in theory [44, 55, 21, 33] would sort to [21, 33, 44, 55]
in c++
how would i make a function that takes in an array int nums[] and in size that will sort the array by the last digit of each element so in theory [44, 55, 21, 33]
would sort to [21, 33, 44, 55]

#include <iostream>
using namespace std;
void sorte(int nums[], int n);
int main()
{ int nums[4] = { 33, 44, 21, 55}; // declaring and initializing an array
sorte(nums, 4); // calling function for sorting
cout<<"Sorted Array\n";
for(int i=0; i<4; i++)
{
cout<<nums[i]<<" "; // priting each element of array
}
return 0;
}
void sorte(int nums[], int n)
{ int ary[4], temp; // Declaring an another array to store the last digit of each element
for(int i=0; i<n; i++)
{
int a = nums[i]%10; // calculating last dgit of each element
ary[i] = a; // Storing each last digit in array i.e. ary[]
}
for(int i = 0; i<4; i++)
{
for(int j = i+1; j<4; j++)
{
if(ary[j] < ary[i]) // Sorting each element by its last digit
{
temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
}
}
Step by step
Solved in 2 steps with 1 images

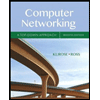
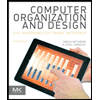
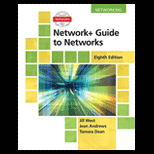
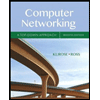
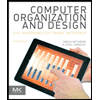
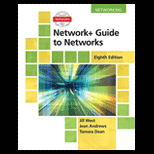
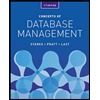
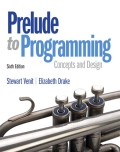
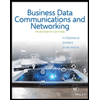