Improve the code below to include and (please describe) each activity pre and post-conditions. Thank you #Import the package import random #Declare and initialize the variable guesses_made = 0 #Read the name from user name = raw_input('Hello! What is your name?\n') #Declare and initialize the variable using random number = random.randint(1, 20) #Display the message print 'Well, {0}, I am thinking of a number between 1 and 20.'.format(name) #Loop executes until the guess is less than "6" while guesses_made < 6: #Read the guess from user guess = int(raw_input('Take a guess: ')) #Increment the guess count guesses_made += 1 #Check whether the guess is less than "number" if guess < number: #Display the error message print 'Your guess is too low.' #Check whether the guess is greater than "number" if guess > number: #Display the error message print 'Your guess is too high.' #Check whether the guess is equal than "number" if guess == number: #Break the loop break #Check whether the guess is equal than "number" if guess == number: #Display the success message print 'Good job, {0}! You guessed my number in {1} guesses!'.format(name, guesses_made) #Otherwise, display the error message else: print 'Nope. The number I was thinking of was {0}'.format(number)
Improve the code below to include and (please describe) each activity pre and post-conditions. Thank you
#Import the package
import random
#Declare and initialize the variable
guesses_made = 0
#Read the name from user
name = raw_input('Hello! What is your name?\n')
#Declare and initialize the variable using random
number = random.randint(1, 20)
#Display the message
print 'Well, {0}, I am thinking of a number between 1 and 20.'.format(name)
#Loop executes until the guess is less than "6"
while guesses_made < 6:
#Read the guess from user
guess = int(raw_input('Take a guess: '))
#Increment the guess count
guesses_made += 1
#Check whether the guess is less than "number"
if guess < number:
#Display the error message
print 'Your guess is too low.'
#Check whether the guess is greater than "number"
if guess > number:
#Display the error message
print 'Your guess is too high.'
#Check whether the guess is equal than "number"
if guess == number:
#Break the loop
break
#Check whether the guess is equal than "number"
if guess == number:
#Display the success message
print 'Good job, {0}! You guessed my number in {1} guesses!'.format(name, guesses_made)
#Otherwise, display the error message
else:
print 'Nope. The number I was thinking of was {0}'.format(number)

Step by step
Solved in 5 steps with 2 images

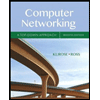
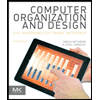
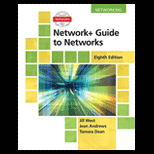
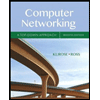
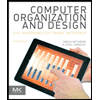
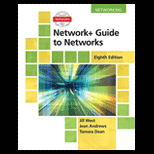
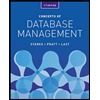
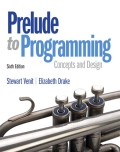
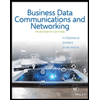