import unittest # -------------------------------------------------------------- # Average # -------------------------------------------------------------- def average(start, end) : ''' Assume that start and end are integers. Return the average all of the numbers from start to end inclusive. For example, average(3, 5) returns 4.0, since (3 + 5) / 2 = 4 ''' pass # -------------------------------------------------------------- # The Testing # -------------------------------------------------------------- class myTests(unittest.TestCase): def test1(self): self.assertAlmostEqual(average(3, 5), 4.0, delta=0.00001) def test2(self): self.assertAlmostEqual(average(-5, 5), 0.0, delta=0.00001) def test3(self): self.assertAlmostEqual(average(100, 200), 150, delta=0.00001) if __name__ == '__main__': unittest.main(exit=True)
import unittest
# --------------------------------------------------------------
# Average
# --------------------------------------------------------------
def average(start, end) :
'''
Assume that start and end are integers.
Return the average all of the numbers from start to end inclusive.
For example,
average(3, 5) returns 4.0, since (3 + 5) / 2 = 4
'''
pass
# --------------------------------------------------------------
# The Testing
# --------------------------------------------------------------
class myTests(unittest.TestCase):
def test1(self):
self.assertAlmostEqual(average(3, 5), 4.0, delta=0.00001)
def test2(self):
self.assertAlmostEqual(average(-5, 5), 0.0, delta=0.00001)
def test3(self):
self.assertAlmostEqual(average(100, 200), 150, delta=0.00001)
if __name__ == '__main__':
unittest.main(exit=True)

We need to define average function in given scenario
Step by step
Solved in 3 steps with 1 images

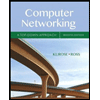
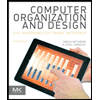
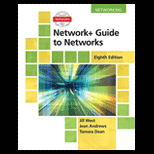
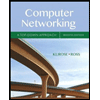
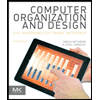
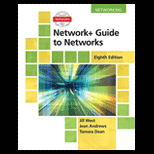
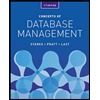
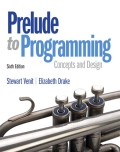
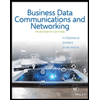