import requests from bs4 import BeautifulSoup import pandas as pd # Prompt user to enter URLs to scrape urls = input("Enter URLs to scrape (comma-separated): ").split(",") # Create an empty list to store scraped data data = [] # Loop through each URL and scrape relevant data for url in urls: response = requests.get(url) soup = BeautifulSoup(response.content, "html.parser") # Extract relevant data from HTML using BeautifulSoup # In this example, we extract all the links in the HTML links = [link.get("href") for link in soup.find_all("a")] # Append the scraped data to the list data.append(links) # Create a pandas DataFrame from the scraped data, if the list is not empty if data: df = pd.DataFrame(data) # Write the DataFrame to an Excel file df.to_excel("scraped_data.xlsx", index=False) else: print("No URLs entered. Exiting program.") I keep running into a syntax error, could you help me edit this program. I am trying to create a webscraper that asks user to enter a website and then it will put the data collected into an excelsheet.
import requests
from bs4 import BeautifulSoup
import pandas as pd
# Prompt user to enter URLs to scrape
urls = input("Enter URLs to scrape (comma-separated): ").split(",")
# Create an empty list to store scraped data
data = []
# Loop through each URL and scrape relevant data
for url in urls:
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
# Extract relevant data from HTML using BeautifulSoup
# In this example, we extract all the links in the HTML
links = [link.get("href") for link in soup.find_all("a")]
# Append the scraped data to the list
data.append(links)
# Create a pandas DataFrame from the scraped data, if the list is not empty
if data:
df = pd.DataFrame(data)
# Write the DataFrame to an Excel file
df.to_excel("scraped_data.xlsx", index=False)
else:
print("No URLs entered. Exiting
I keep running into a syntax error, could you help me edit this program.
I am trying to create a webscraper that asks user to enter a website and then it will put the data collected into an excelsheet.

Step by step
Solved in 3 steps

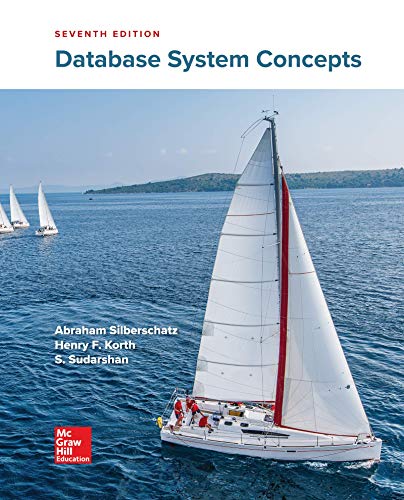
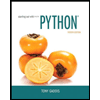
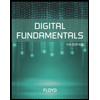
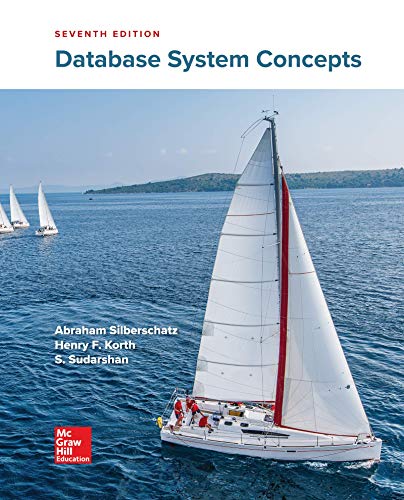
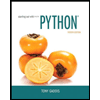
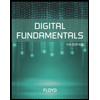
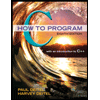
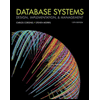
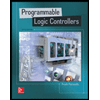