import random # 1. make List of numbers from 0 to n-1 # 2. randomly shuffle the List # 3. insert the random List elements in order into a tree. # 4. return the height of the resulting ree. def run_single_experiment (n): # your code here nums = [] for i in range (n): nums.append(i) random.shuffle (nums) root Node (nums [0]) for i in range (1,n): root.insert(nums[i]) return height (root)
import random # 1. make List of numbers from 0 to n-1 # 2. randomly shuffle the List # 3. insert the random List elements in order into a tree. # 4. return the height of the resulting ree. def run_single_experiment (n): # your code here nums = [] for i in range (n): nums.append(i) random.shuffle (nums) root Node (nums [0]) for i in range (1,n): root.insert(nums[i]) return height (root)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help me complete this python code.
Faster answer
![import random.
# 1. make List of numbers from 0 to n-1
# 2.
randomly shuffle the list
# 3.
insert the random List elements in order into a tree.
# 4. return the height of the resulting ree.
def run_single_experiment (n):
# your code here
nums = []
for i in range (n):
nums.append(i)
random.shuffle (nums)
root Node (nums[0])
for i in range (1,n):
root.insert(nums[i])
return height (root)
def run_multiple_trials (n, numTrials):
1st_of_depths = [run_single_experiment (n) for j in range(numTrials)]
return (sum(1st_of_depths)/1en (1st_of_depths), 1st_of_depths)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0b00dca9-c4c6-41f8-8514-8224c60ce61e%2F2578abd5-3a77-495b-9c34-9c00829fbc0c%2F9dsezn_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import random.
# 1. make List of numbers from 0 to n-1
# 2.
randomly shuffle the list
# 3.
insert the random List elements in order into a tree.
# 4. return the height of the resulting ree.
def run_single_experiment (n):
# your code here
nums = []
for i in range (n):
nums.append(i)
random.shuffle (nums)
root Node (nums[0])
for i in range (1,n):
root.insert(nums[i])
return height (root)
def run_multiple_trials (n, numTrials):
1st_of_depths = [run_single_experiment (n) for j in range(numTrials)]
return (sum(1st_of_depths)/1en (1st_of_depths), 1st_of_depths)
![7]: %matplotlib inline
from matplotlib import pyplot as plt
import math
(avg64, 1st_of_results_64) = run_multiple_trials (64, 1000)
plt.hist (1st_of_results_64)
plt.xlim (0,64)
plt.xlabel('Depth of Tree')
plt.ylabel('Frequency')
plt.title('Histogram of depths for n = 64')
print (f'Average depth for 64 = {avg64}')
assert avg64 <= 12 and avg64 >= 8
plt.figure()
(avg128, 1st_of_results_128) = run_multiple_trials (128,1000)
print (f'Average depth for 128 = {avg128}')
assert avg128 <= 16 and avg128>= 12.
plt.hist (1st_of_results_128)
plt.xlim (0,128)
plt.xlabel('Depth of Tree')
plt.ylabel('Frequency')
plt.title('Histogram of depths for n = 128')
nmin=16
nmax=64
1st_of_average_depths = [ run_multiple_trials (j, 1000) [0] for j in range(nmin, nmax)]
plt. figure()
11 = plt.plot(range (nmin, nmax), 1st_of_average_depths, label='Avg. Depth')
plt.xlabel('n')
plt.ylabel('depth')
12
plt.plot(range (nmin, nmax), [1.6* math.log(j)/math.log(2) for j in range(nmin, nmax)], --¹, label='1.6log2 (n)')
13 = plt.plot(range (nmin, nmax), [2.2* math.log(j)/math.log(2) for j in range (nmin, nmax)], ' --b',label='2.21og2 (n)')
#plt. Legend (handles-[L1, L2, L3])
plt.title('Average depth as a function of n and comparison with 1.6 log2 (n), 2.2 log2 (n)')
print('Passed all tests -- 15 points')](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0b00dca9-c4c6-41f8-8514-8224c60ce61e%2F2578abd5-3a77-495b-9c34-9c00829fbc0c%2Fmk1t0ys_processed.jpeg&w=3840&q=75)
Transcribed Image Text:7]: %matplotlib inline
from matplotlib import pyplot as plt
import math
(avg64, 1st_of_results_64) = run_multiple_trials (64, 1000)
plt.hist (1st_of_results_64)
plt.xlim (0,64)
plt.xlabel('Depth of Tree')
plt.ylabel('Frequency')
plt.title('Histogram of depths for n = 64')
print (f'Average depth for 64 = {avg64}')
assert avg64 <= 12 and avg64 >= 8
plt.figure()
(avg128, 1st_of_results_128) = run_multiple_trials (128,1000)
print (f'Average depth for 128 = {avg128}')
assert avg128 <= 16 and avg128>= 12.
plt.hist (1st_of_results_128)
plt.xlim (0,128)
plt.xlabel('Depth of Tree')
plt.ylabel('Frequency')
plt.title('Histogram of depths for n = 128')
nmin=16
nmax=64
1st_of_average_depths = [ run_multiple_trials (j, 1000) [0] for j in range(nmin, nmax)]
plt. figure()
11 = plt.plot(range (nmin, nmax), 1st_of_average_depths, label='Avg. Depth')
plt.xlabel('n')
plt.ylabel('depth')
12
plt.plot(range (nmin, nmax), [1.6* math.log(j)/math.log(2) for j in range(nmin, nmax)], --¹, label='1.6log2 (n)')
13 = plt.plot(range (nmin, nmax), [2.2* math.log(j)/math.log(2) for j in range (nmin, nmax)], ' --b',label='2.21og2 (n)')
#plt. Legend (handles-[L1, L2, L3])
plt.title('Average depth as a function of n and comparison with 1.6 log2 (n), 2.2 log2 (n)')
print('Passed all tests -- 15 points')
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
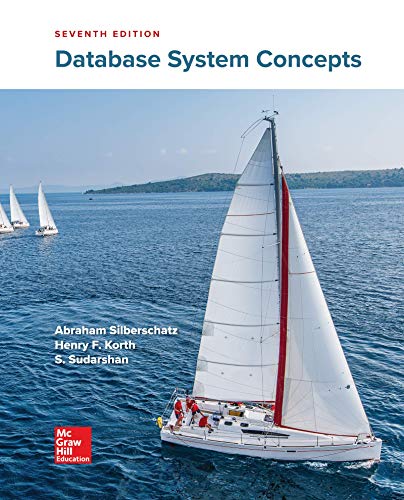
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
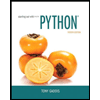
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
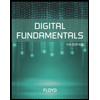
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
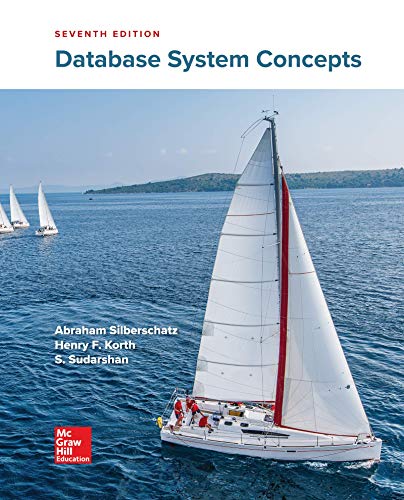
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
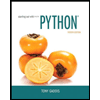
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
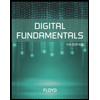
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
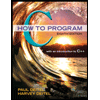
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
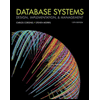
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
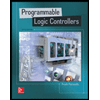
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education