Implement this C program using at least three arrays to store the interest, principle and balance for each payment respectively. For example, interest[0] stores the paid interest in the first payment. Name this C program as loanCalcArr.c.
Implement this C program using at least three arrays to store the interest, principle and balance for each payment respectively. For example, interest[0] stores the paid interest in the first payment. Name this C program as loanCalcArr.c.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C program
![IZATION SCHEDULE
12:20
l 5Gº
Expert Q&A
Done
2) Implement this C program using at least three arrays to store the interest, principle
and balance for each payment respectively. For example, interest[0] stores the paid
interest in the first payment. Name this C program as loanCalcArr.c.
3) Implement this C program using at least three arrays to store the interest, principle
and balance after each payment respectively. For example, interest[0] stores the paid
interest in the first payment. Besides, please use three pointers to visit the element in
the three arrays separately. Name this C program as loanCalcPtr.c.
4) Implement this C program by defining a structure for each payment. The structure
should have at least three members for the interest, principle and balance separately.
And store all the payments in a structure array (the max size of which could be 100).
Name this C program as loanCalcstruct.e
In this C program, the input and output are defined as following:
• Input: amount of loan, interest rate per year and number of payments
• Output: a table of amortization schedule (also called payment schedule) containing
payment number, monthly payment, principal paid, interest paid and new balance at
each row.
The attached screenshot below shows a sample of the output.
Yuans-MacBook-Pro:PC yuanlong$ ./Loancale
Enter amount of loan : $ 500
Enter Interest rate per year :7.5
Enter number of payments : 5
Montly payment should be 101.88
Payment
$101.88
$101.88
$101.88
$101.88
$101.88
AMORTIZATION SCHEDULE
Principal
$98.76
$99.38
$100.00
$100.62
$101.25
Interest
$3.12
$2.51
$1.89
$1.26
$0.63
Balance
$401.24
$301.87
$201.87
$101.25
$0.00
Note
• monthly payments are equal. The way to calculate monthly payment and other values
for each row are provided in Appendix.
• The C program can be implemented within 80 lines of code. If your program is longer
than 80 lines, you may need to think about how to simplify your program.
Hint: To print out a percentage %, please use %%. You may need to use C math library to
calculate the powers of numbers. To compile a C program using math library, you must add
option Im at the end of the cc command to link math library. Eg. gce -o test test.c -Im
1. Calculating the monthly payment.
(Note: The following instruction is from
http://woww.vertex42.com/ExcelArticles/amortization-calculation html )
The formula for calculating the payment amount is shown below.
r(1 +r)"
A = B
(1+r)" – 1
where
• A- payment Amount per period (monthly payment)
• Be initial Balance (loan amount)
• r interest rate per period
• n total number of payments or periods
Example 1: What would the monthly payment be on a 5-year, $20,000 car loan with a
nominal 7.5% annual interest rate?
B-$20,000
r= 7.5% per year /12 months = 0.625%- 0.00625 per period
n-5 years 12 months 60 total periods
A- 20000. (1es - 400.76
0.002s (1
(1+0.00 -
2. Calculating the Principal(P), Interest(INT) and Balance(B) for each
раyment.
The formula is shown below.
INT(n) = B(n - 1) r
P(n) -A- INT (n):
B(n) = B(n - 1)- P(n):
Where INT (n), P(m) and B(n) are the paid principal, paid interest and new balance for the
nth payment. And B(0) means the loan amount.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F298d9fb3-2c12-4d12-a549-bbc8cb245a18%2Fcb705f9a-c254-4bea-8145-46de6ce287c9%2Fvua8eeh_processed.jpeg&w=3840&q=75)
Transcribed Image Text:IZATION SCHEDULE
12:20
l 5Gº
Expert Q&A
Done
2) Implement this C program using at least three arrays to store the interest, principle
and balance for each payment respectively. For example, interest[0] stores the paid
interest in the first payment. Name this C program as loanCalcArr.c.
3) Implement this C program using at least three arrays to store the interest, principle
and balance after each payment respectively. For example, interest[0] stores the paid
interest in the first payment. Besides, please use three pointers to visit the element in
the three arrays separately. Name this C program as loanCalcPtr.c.
4) Implement this C program by defining a structure for each payment. The structure
should have at least three members for the interest, principle and balance separately.
And store all the payments in a structure array (the max size of which could be 100).
Name this C program as loanCalcstruct.e
In this C program, the input and output are defined as following:
• Input: amount of loan, interest rate per year and number of payments
• Output: a table of amortization schedule (also called payment schedule) containing
payment number, monthly payment, principal paid, interest paid and new balance at
each row.
The attached screenshot below shows a sample of the output.
Yuans-MacBook-Pro:PC yuanlong$ ./Loancale
Enter amount of loan : $ 500
Enter Interest rate per year :7.5
Enter number of payments : 5
Montly payment should be 101.88
Payment
$101.88
$101.88
$101.88
$101.88
$101.88
AMORTIZATION SCHEDULE
Principal
$98.76
$99.38
$100.00
$100.62
$101.25
Interest
$3.12
$2.51
$1.89
$1.26
$0.63
Balance
$401.24
$301.87
$201.87
$101.25
$0.00
Note
• monthly payments are equal. The way to calculate monthly payment and other values
for each row are provided in Appendix.
• The C program can be implemented within 80 lines of code. If your program is longer
than 80 lines, you may need to think about how to simplify your program.
Hint: To print out a percentage %, please use %%. You may need to use C math library to
calculate the powers of numbers. To compile a C program using math library, you must add
option Im at the end of the cc command to link math library. Eg. gce -o test test.c -Im
1. Calculating the monthly payment.
(Note: The following instruction is from
http://woww.vertex42.com/ExcelArticles/amortization-calculation html )
The formula for calculating the payment amount is shown below.
r(1 +r)"
A = B
(1+r)" – 1
where
• A- payment Amount per period (monthly payment)
• Be initial Balance (loan amount)
• r interest rate per period
• n total number of payments or periods
Example 1: What would the monthly payment be on a 5-year, $20,000 car loan with a
nominal 7.5% annual interest rate?
B-$20,000
r= 7.5% per year /12 months = 0.625%- 0.00625 per period
n-5 years 12 months 60 total periods
A- 20000. (1es - 400.76
0.002s (1
(1+0.00 -
2. Calculating the Principal(P), Interest(INT) and Balance(B) for each
раyment.
The formula is shown below.
INT(n) = B(n - 1) r
P(n) -A- INT (n):
B(n) = B(n - 1)- P(n):
Where INT (n), P(m) and B(n) are the paid principal, paid interest and new balance for the
nth payment. And B(0) means the loan amount.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
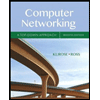
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
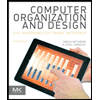
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
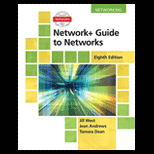
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
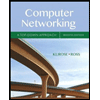
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
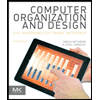
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
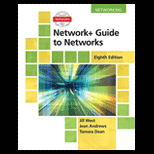
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
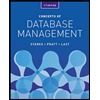
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
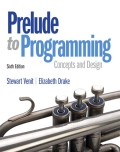
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
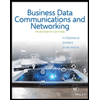
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY