Implement solution for put( Student s) (see code and description below) public class Course { public String code; public int capacity; public SLinkedList[] studentTable; public int size; public SLinkedList waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList(); this.capacity = 10; } public Course(String code, int capacity) { this.code = code; this.studentTable = new SLinkedList[capacity]; this.size = 0; this.waitlist = new SLinkedList<>(); this.capacity = capacity; } public void changeArrayLength(int m) { public SLinkedList[] newStudentTable; for (int i = 0; i < m; i++) { newStudentTable[i] = new SLinkedList; } for (int i = 0; i < studentTable.length; i++) { for (int j = 0; j < studentTable[i].size(); j++) { int tempIndex = studentTable[i].get(j).getId() % m; newStudentTable[tempIndex].add(studentTable[i].get(j)); } } studentTable = newStudentTable; } public boolean put(Student s) { // insert your solution here and modify the return statement return false; } put( Student s) – returns true if the method successfully registers the student or adds the student onto the waitlist; returns false otherwise, namely in one of the three following cases: (1) if the student was already registered in the course, or (2) the student was already on the waitlist, or (3) the student had already reached their personal maximum of three courses. Assuming none of these three conditions was met, the student can then be either registered for the course or put on the waitlist. If the course is not yet at capacity, then the student can be registered. In that case, the student’s id is first used to determine where the student goes in the student table, namely in which linked list it goes: then, the student is put in the linked list in array slot id % m, where m is the length of the array (the course capacity). Note that you should go directly to slot id % m rather than iterating through all the slots. Indeed this is the entire point of taking the mod! If the course had already reached its registration capacity, then the student is added to the waitlist. If the waitlist was already at its full capacity, then the put() method increases the registration capacity of the course by 50%, as mentioned earlier – see waitlist field. (Increasing the capacity of the course by expanding the table is analogous to finding a bigger room). Use the changeArrayLength() method as a helper here. The put() method then registers all students currently on the waitlist, by moving them from the waitlist to the studentTable. (Note that the waitlist capacity is 50% of the course capacity, which was conveniently chosen such that all students in the waitlist can be registered.) Finally, the new student is added to the empty waitlist. For example, if the studentTable array length is 10 and the course is full (10 students registered) and the waitlist was already full (5 students on waitlist), the method would increase the table size – that is, capacity – to 15 and make a new empty waitlist. The new student would then be added to this empty waitlist. Note that the maximum size of the new waitlist would be 7, since the course capacity has increased.
Implement solution for put( Student s) (see code and description below)
public class Course {
public String code;
public int capacity;
public SLinkedList<Student>[] studentTable;
public int size;
public SLinkedList<Student> waitlist;
public Course(String code) {
this.code = code;
this.studentTable = new SLinkedList[10];
this.size = 0;
this.waitlist = new SLinkedList<Student>();
this.capacity = 10;
}
public Course(String code, int capacity) {
this.code = code;
this.studentTable = new SLinkedList[capacity];
this.size = 0;
this.waitlist = new SLinkedList<>();
this.capacity = capacity;
}
public void changeArrayLength(int m) {
public SLinkedList<Student>[] newStudentTable;
for (int i = 0; i < m; i++) {
newStudentTable[i] = new SLinkedList<Student>;
}
for (int i = 0; i < studentTable.length; i++) {
for (int j = 0; j < studentTable[i].size(); j++) {
int tempIndex = studentTable[i].get(j).getId() % m;
newStudentTable[tempIndex].add(studentTable[i].get(j));
}
}
studentTable = newStudentTable;
}
public boolean put(Student s) {
// insert your solution here and modify the return statement
return false;
}
put( Student s) – returns true if the method successfully registers the student or adds the student onto the waitlist; returns false otherwise, namely in one of the three following cases: (1) if the student was already registered in the course, or (2) the student was already on the waitlist, or (3) the student had already reached their personal maximum of three courses. Assuming none of these three conditions was met, the student can then be either registered for the course or put on the waitlist. If the course is not yet at capacity, then the student can be registered. In that case, the student’s id is first used to determine where the student goes in the student table, namely in which linked list it goes: then, the student is put in the linked list in array slot id % m, where m is the length of the array (the course capacity).
Note that you should go directly to slot id % m rather than iterating through all the slots. Indeed this is the entire point of taking the mod!
If the course had already reached its registration capacity, then the student is added to the waitlist. If the waitlist was already at its full capacity, then the put() method increases the registration capacity of the course by 50%, as mentioned earlier – see waitlist field. (Increasing the capacity of the course by expanding the table is analogous to finding a bigger room). Use the changeArrayLength() method as a helper here. The put() method then registers all students currently on the waitlist, by moving them from the waitlist to the studentTable. (Note that the waitlist capacity is 50% of the course capacity, which was conveniently chosen such that all students in the waitlist can be registered.) Finally, the new student is added to the empty waitlist. For example, if the studentTable array length is 10 and the course is full (10 students registered) and the waitlist was already full (5 students on waitlist), the method would increase the table size – that is, capacity – to 15 and make a new empty waitlist. The new student would then be added to this empty waitlist. Note that the maximum size of the new waitlist would be 7, since the course capacity has increased.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

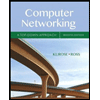
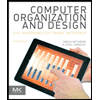
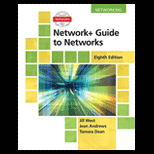
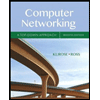
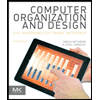
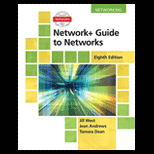
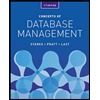
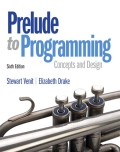
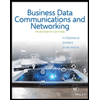