Im working on homework and am stuck. I have created the code for taking the input of the user but I am not sure how to separate them to find how many are left. The program must compute the number of terms a student has remaining, and this should be an integer. For example, if a student has 15 courses remaining and plans to take 2 a term then they need 8 terms to complete the courses. In other words, you can’t have 7.5 terms because the student must attend an entire term. import java.util.Scanner; import java.util.Arrays; public class MainClass { public static void main(String[] args) { Scanner input = new Scanner(System.in); int[][] students = new int [10][2]; for(int i = 0; i < 10; i++) { for(int j = 0; j < 2; j++) { students[i][j] = input.nextInt(); } } int grades = 0; System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student1 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student2 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student3 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student4 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student5 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student6 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student7 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student8 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student9 = input.nextInt(); System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: "); int Student10 = input.nextInt(); } }
Im working on homework and am stuck. I have created the code for taking the input of the user but I am not sure how to separate them to find how many are left.
The program must compute the number of terms a student has remaining, and this should be an integer. For example, if a student has 15 courses remaining and plans to take 2 a term then they need 8 terms to complete the courses. In other words, you can’t have 7.5 terms because the student must attend an entire term.
import java.util.Scanner;
import java.util.Arrays;
public class MainClass
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
int[][] students = new int [10][2];
for(int i = 0; i < 10; i++)
{
for(int j = 0; j < 2; j++)
{
students[i][j] = input.nextInt();
}
}
int grades = 0;
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student1 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student2 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student3 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student4 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student5 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student6 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student7 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student8 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student9 = input.nextInt();
System.out.print("Enter number of courses student has left to graduate and how many courses they plan to take each term: ");
int Student10 = input.nextInt();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

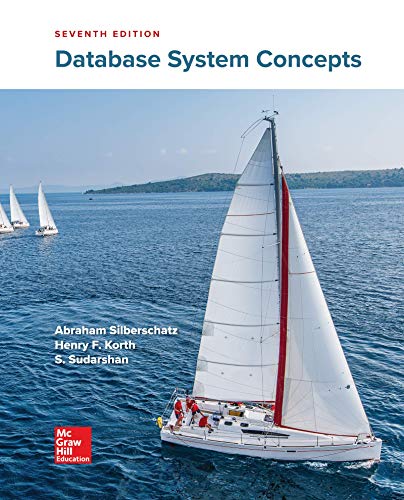
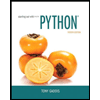
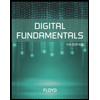
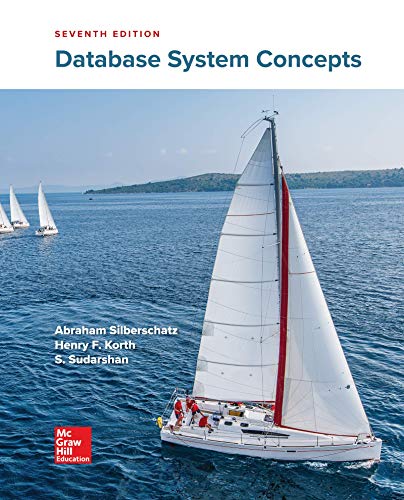
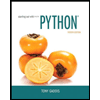
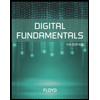
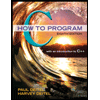
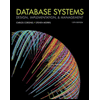
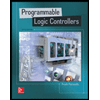